Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Movie Data Exchange Components
This chapter provides background information about movie data exchange components. After reading this chapter, you should understand why these components exist and whether you need to create or use one.
Movie data exchange components allow applications to place various types of data into a QuickTime movie or extract data from a movie in a specified format. Movie data import components translate foreign (that is, nonmovie) data formats into QuickTime movie data format. For example, a movie data import component might convert images from a paint application into frames in a QuickTime movie.
Conversely, movie data export components convert movie data into other formats, so that the data can be used by other applications. As an example, a movie data export component might allow an application to extract the sound track from a QuickTime movie in AIFF format. The extracted sound track may then be manipulated by applications that are not QuickTime-aware.
Applications use the services of movie data exchange components by calling the Movie Toolbox. Figure 5-1 shows the relationship between the Movie Toolbox and movie data import components while Figure 5-2 shows how movie data export components fit into the picture.
If you are writing a media handler that works with a new type of data, you will probably need to use one or more data exchange components to facilitate the importing and exporting of data to QuickTime movies.
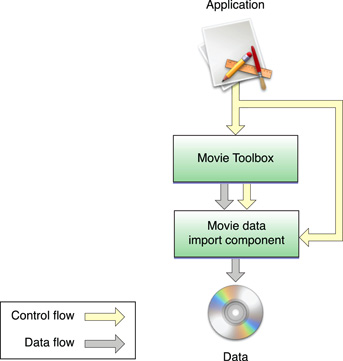
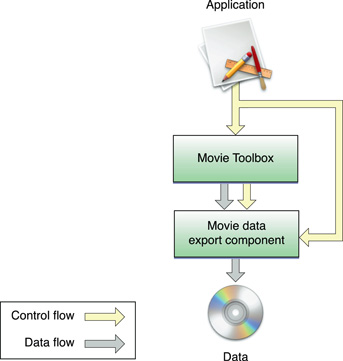
Saving and Restoring Settings
QuickTime has always provided many support mechanisms for importing media from other formats into QuickTime movies, as well as exporting from QuickTime movies to other media formats. Importing and exporting are handled by movie data exchange components.
You write import and export components to allow a user to perform importing and exporting, respectively. Your component provides a routine that presents a dialog box for the user to change options. For an import component, you need to implement MovieImportDoUserDialog
; for an export component, you must implement MovieExportDoUserDialog
. For example, the text import component presents a dialog box with options for setting the font, size, and style of the text media it will add to the movie. The WAVE audio export component presents the standard sound compression dialog box, so that sample rate and sample size can be specified for the generated WAVE file.
QuickTime lets you retrieve the current settings from the still-open import or export component. In addition, you can restore a component’s current settings to previously-retrieved settings. The restoration does not involve any user interface. This may be advantageous for application developers who want to provide preferences for the last settings used or want to perform batch importing or exporting, using previously-established settings.
QuickTime makes it possible for your application to retrieve and store the settings of import and export components without having to present the user with a user interface, such as a settings dialog box, to accomplish the task.
Two scenarios illustrate how saved settings can be useful. In the first scenario, an application presents an importer or exporter component’s configuration dialog the first time that component is used and then saves the settings so they can be restored without the user having to go fill out the configuration dialog again. In another scenario, an application might use settings to implement preset configurations that the user often wants.
QuickTime enables movie export components to associate resources that hold one or more named presets for that exporter. The dialog accessible through ConvertMovieToFile
automatically builds a menu of all presets for the currently selected exporter allowing a user to export without having to go through the exporter’s custom dialog.
It is also possible to include component resources that serve as named presets to be used with the export component. These resources include the same kind of settings just described. See Movie Exporter Presets.
For information about using the save-and-restore component settings mechanism, refer to the section Implementing Movie Data Exchange Components.
Movie Exporter Presets
The ConvertMovieToFile
function retrieves preset information and includes an additional popup menu showing presets for the currently selected exporter. Current preset component resources include 'stg#'
and 'sttg'
.
Implementing Movie Data Exchange Components
The following section discusses how you can implement component routines to save and restore component settings available in QuickTime.
Standard Compression Components and Settings
QuickTime includes two settings-related component calls to both the video and sound Standard Compression components: SCGetSettingsAsAtomContainer
and SCGetSettingsAsAtomContainer
These may also be useful for implementing movie data exchange components. The SCGetSettingsAsAtomContainer
routine returns a QT atom container with the current configuration of the particular compression component. SCSetSettingsFromAtomContainer
resets the compression component’s current configuration. Applications that want to save settings for standard compression components should use these calls.
Exporting Text
The text export and import components provide features that make it easier to work with the data in a text track in a QuickTime movie. Text descriptors are formatting commands that you can embed within a text file. Time stamps describe a text sample’s starting time and duration.
The text export and import components make it easier to edit and format text using an external tool, such as a text editor or word processor. When you export text from a text track, you can optionally export text descriptors and time stamps for the text. You can open the text file in a word processor and make changes to the text, style, color, and time stamps. You can then import the edited text to a text track where all the timing, style, color and time stamp information will be present.
When you export text, you control whether text descriptors and time stamps are to be exported by selecting the appropriate options in the text export settings dialog box, shown in Figure 5-3. To display this dialog box programmatically, you call the MovieExportDoUserDialog
function.
Based on the options you specify in the text export settings dialog box, the text export component is assigned one of three text export option constants: kMovieExportTextOnly
, kMovieExportAbsoluteTime
, or kMovieExportRelativeTime
.
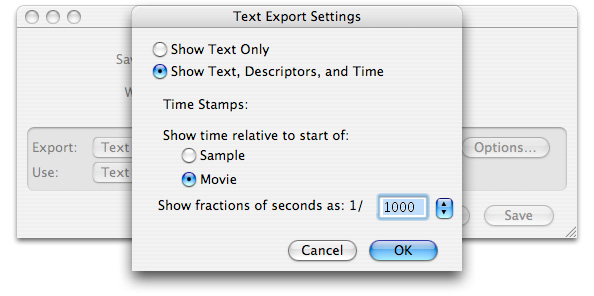
If you choose “Show Text Only,” the text component is assigned the export option constant kMovieExportTextOnly
. In this case, the text component exports only text samples, without text descriptors or time stamps. This option is useful when you want to export only the text from a movie and you do not intend to import the text back into a movie.
If you select “Show Text, Descriptors, and Time,” the text component is assigned one of two export option constants, depending on the format you specify for time stamps:
If you specify time stamps to be relative to the start of the movie, the text component is assigned the export option constant
kMovieExportAbsoluteTime
. In this case, time stamps are calculated relative to the start of the movie. For example, in exported text with absolute time stamps, the time stamp [00:00:04.000] indicates that a text sample begins 4 seconds after the start of the movie.If you specify time stamps to be relative to the sample, the text component is assigned the export option constant
kMovieExportRelativeTime
. In this case, the time stamp for each sample is calculated relative to the end of the previous sample. For example, in exported text with relative time stamps, the time stamp [00:00:04.000] indicates that a text sample begins 4 seconds after the beginning of the previous sample. In other words, the previous sample lasts 4 seconds.
In both cases, text export component exports text, along with both text descriptors and time stamps. For more information about time stamps, see Time Stamps.
The text export component provides two functions you can use to access the component’s text export option programmatically. To retrieve the current value of the text export option, you call TextExportGetSettings
. To set the value of the text export option, you call TextExportSetSettings
.
The Text Export Settings dialog box also allows you to specify the time scale the text component uses to specify the fractional part of a time stamp. The value should be between 1 and 10000, inclusive. The text export component provides two functions you can use to access the component’s time scale programmatically. To retrieve the time scale, call TextExportGetTimeFraction
. To set the time scale, call TextExportSetTimeFraction
.
Time Stamps
When you export text and text descriptors from a text track, the text component also exports a time stamp for each sample. The time stamp indicates the starting and ending time of the sample, either relative to the start of the movie (kMovieExportAbsoluteTime
) or to the end of the previous sample (kMovieExportRelativeTime
). On import, the time stamps maintain the timing positions of the text samples relative to other media in the movie.
The format of a time stamp is
[HH:MM:SS.xxx] |
where HH
represents the number of hours, MM
represents the number of minutes, SS
represents the number of seconds, and xxx
represents the mantissa (the fractional part of a second). The mantissa is expressed in the time scale of the text track. For example, if the time scale of the text track is 600, the time stamp [00:00:07.300] is interpreted as 7.5 seconds. If the time scale of the text track is 10, the time stamp [00:00:07.5] is also interpreted as 7.5 seconds. The maximum time scale for a text track is 10000.
When a text export component exports a text sample, it first exports the time stamp, followed by a return character. Then, it exports the sample’s text and text descriptors. If a text sample does not contain any text, the text component exports the time stamp and return character, but no text.
Text Descriptors
A text descriptor is a formatting command that describes the text that follows it. Exporting text with text descriptors allows you to edit text from a text track, including its formatting, in an external program, such as a text editor or word processor. When you import the edited text, the formatting you specified with the text descriptors is preserved. This provides an easy way to localize movies for different languages, correct spelling, change styles, or modify text behavior.
A text descriptor has the format { descriptor }. For example, the text descriptor {bold}
sets the text style in the current text sample and all subsequent text samples. Some text descriptors, such as {bold}
, have no parameters. Other text descriptors have one or more parameters. For text descriptors with parameters, the descriptor is followed by a colon and its parameters, separated by commas. You can specify text descriptors using either uppercase or lowercase characters, with or without spaces separating the parameters:
{descriptor: parameter1, ..., parameterN }
For example, the text descriptor {font
:New
York}
sets the text font in the current text sample and all subsequent text samples to the New York font. The New York font is applied to all text until a second {font
:}
text descriptor is issued.
A text stream that contains text descriptors and time stamps should always begin with the text descriptor {QTtext}
, followed by any number of text descriptors in any order. If the text import component detects a typographical error inside a descriptor while importing a text file, it may generate partial results or an error message stating that the text file cannot be converted.
When text with text descriptors is imported into a track, the information represented by the descriptors is stored in a text display data structure (type TextDisplayData
). Text descriptors whose possible values are on
and off
are used to set flags in the displayFlags
field of the text display data structure. Each sample in the text track has a corresponding text display data structure that contains the text attributes of the sample. For more information, see Text Display Data Structure.
MIME Type List
The MovieImportGetMIMETypeList
function returns a list of the MIME types supported by a movie import component. This list is contained in the QT atom container described in this section. For more information about QT atom containers, see the QuickTime Atom documentation.
At the top level of the atom container are three atoms for each supported MIME type. The atoms whose IDs are 1 describe the first supported MIME type, the atoms whose IDs are 2 describe the second supported MIME type, and so on.
An atom of type kMimeInfoMimeTypeTag
contains a string that identifies the MIME type, such as image/jpeg
or image/x-jpeg
.
The atom of type kMimeInfoFileExtensionTag
contains a string that specifies likely file extensions for files of this MIME type, such as jpg
, jpe
, and jpeg
. If there is more than one extension, the extensions are separated by commas.
The atom of type kMimeInfoDescriptionTag
contains a string describing the MIME type for end users, such as “JPEG Image.”
These atom types contain neither a Pascal nor a C string. The atom types are simply ASCII characters; an atom’s size is the number of characters. For best performance, include a public component resource of type 'mime'
and ID 1 with your exporter.
Figure 5-4 illustrates a MIME type list.
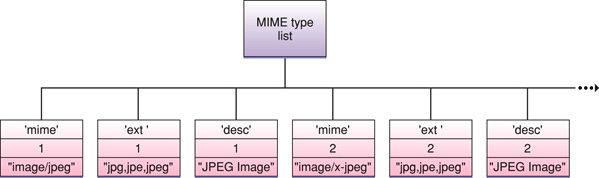
Text Display Data Structure
The TextDisplay
data structure contains formatting information for a text sample. When the text export component exports a text sample, it uses the information in this structure to generate the appropriate text descriptors for the sample. Likewise, when the text import component imports a text sample, it sets the appropriate fields in the text display data structure based on the sample’s text descriptors.
struct TextDisplayData { |
long displayFlags; |
long textJustification; |
RGBColor bgColor; |
Rect textBox; |
short beginHilite; |
short endHilite; |
RGBColor hiliteColor; |
Boolean doHiliteColor; |
SInt8 filler; |
TimeValue scrollDelayDur; |
Point dropShadowOffset; |
short dropShadowTransparency; |
}; |
typedef struct TextDisplayData TextDisplayData; |
Term |
Definition |
---|---|
|
Contains flags that represent the values of the following text descriptors: |
|
Specifies the alignment of the text in the text box. Possible values are |
|
Specifies the background color of the rectangle specified by the |
|
Specifies the rectangle of the text box. |
|
Specifies the one-based index of the first character in the sample to highlight. |
|
Specifies the one-based index of the last character in the sample to highlight. |
|
Specifies whether to use the color specified by the |
|
Reserved. |
|
Specifies a scroll delay. The scroll delay is specified as the number of units of delay in the text track's time scale. For example, if the time scale is 600, a scroll delay of 600 causes the sample text to be delayed one second. In order for this field to take effect, scrolling must be enabled. |
|
Specifies an offset for the drop shadow. For example, if the point specified is (3,4), the drop shadow is offset 3 pixels to the right and 4 pixels down. In order for this field to take effect, drop shadowing must be enabled. |
|
Specifies the intensity of the drop shadow as a value between 0 and 255. In order for this field to take effect, drop shadowing must be enabled. |
Importing Text
When you import text, you can override the text descriptors in the text file by specifying options in the Text Import Settings dialog box, shown in Figure 5-5.
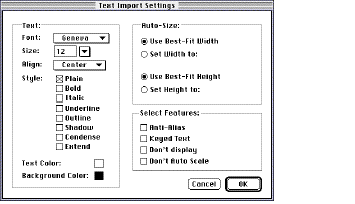
On import, the settings specified in the dialog box are applied to all imported samples. To display this dialog box programmatically, you can call the MovieImportDoUserDialog
function.
Importing In Place
Some movie data import components can create a movie from a file without having to write to a separate disk file. Examples include MPEG, AIFF, DV, and AVI import components; data in files of these types can be played directly by the appropriate media handler components without any data conversion. In such cases it is inappropriate for the user to have to specify a destination file, because there is no need for such a file.
If your import component can operate in this manner, set the canMovieImportInPlace
flag to 1 in your component flags when you register your component. The standard file dialog box uses this flag to determine how to import files. The OpenMovieFile
and NewMovieFromFile
functions use this flag to open some kinds of files as movies.
Audio CD Import Component
QuickTime includes an audio CD import component. This movie import component allows users to open audio CD tracks from the QuickTime standard file preview dialog box, then convert and save the audio as a movie.
When you open an audio track on an Apple CD-ROM drive (or equivalent), the Open button changes to a Convert button. When you click Convert, the audio CD import options dialog box appears. Use this dialog box to configure the sound settings. You can specify the sample rate, sample size, and channel settings. You can also select the portion of the track that you want to convert.
DV Video Import and Export Components
QuickTime includes movie data exchange components for DV video.
DV Movie Import Component
The DV movie import component converts a file containing DV video data into a QuickTime movie. The input file must be a Mac OS file of type 'dvc!'
or a Windows file with the .dv file extension. The output file contains a QuickTime movie with two tracks:
A video track whose samples are of type
kDVNTSCCodecType
for NTSC video data orkDVCPALCodecType
for PAL video data.A sound track whose samples are of type
kDVAudioFormat
.
The data is converted in place, as described in Importing In Place, and the import operation typically takes less than a second. Because both tracks in the QuickTime file refer to the same data, flattening the file creates a file that is twice the size of the original DV data.
You can perform the same operations on the resulting QuickTime movie (including playback, editing, and stepping) that you can for other QuickTime movies. Because video and audio are interleaved in the underlying data, applications for editing movies should make it possible to create a separate file that contains only the audio data for the movie.
This can be done by calling the ConvertMovieToFile
function and specifying kQTFileTypeAIFF
as the destination file format.
DV Movie Export Component
The DV movie export component converts a file containing a QuickTime movie to a file containing DV data for the movie.
The input file must contain a video track; the DV movie export component cannot convert a movie that contains only audio.
If the video track in the QuickTime movie is already in DV format, the DV movie export component does not recompress the video. This makes it possible to edit DV video in QuickTime and then export it without any loss of video quality due to recompression.
Exporting DV Data from an Application
An application can export DV data without creating a QuickTime movie file by using a callback procedure to supply media data, as described in Exporting Data from Sources Other Than Movies.
Exporting Data from Sources Other Than Movies
A movie data export component can be written to export data from sources other than QuickTime movies. To do this, the software that exports data must implement callback functions that provide services to the movie data export component. The callback functions and other functions that support this feature are described in this section.
The export component’s MovieExportFromProceduresToDataRef
routine performs data exporting. When executed, that routine makes callbacks to retrieve characteristics, called properties, and media data from each data source. Characteristics for a video data source might include the width, height, and image compression settings to be used; the media data would be the image description and image data corresponding to a particular movie time. A sound data source would have sound-related characteristics and sound sample data.
Follow these basic steps to export data:
Find an exporter for the format that implements
MovieExportFromProceduresToDataRef
.Open that export component.
For each data source, call the export component’s
MovieExportAddDataSource
to provide the property and data callbacks that the exporter should call during export.Call
MovieExportFromProceduresToDataRef
to perform the export.
Determining What Kind of Tracks a Component Supports
The following code sample can be used to determine which track types a given movie export component supports.
Instantiating the Data Export Component
The first step in using a movie data export component to create an AIFF file is instantiating an AIFF data export component. An example of this is shown in Listing 5-1.
Listing 5-1 Instantiating a data export component
QTAtomContainer container = nil; |
ComponentDescription cd; |
long count, i; |
cd.componentType = MovieExportType; |
cd.componentSubType = 0; |
cd.componentManufacturer = 0; |
cd.componentFlags = 0; |
cd.componentFlagsMask = 0; |
GetComponentPublicResourceList(kQTMovieExportTrackInfoResourceType, 1, 0, &cd, nil, nil, &container); |
count = QTCountChildrenOfType(container, kParentAtomIsContainer, OSTypeConst('comp')); |
for (i=1; i<=count; i++) { |
QTAtom compAtom, trkResourceAtom; |
Component c; |
compAtom = QTFindChildByIndex(container, kParentAtomIsContainer, |
OSTypeConst('comp'), i, (long*)&c); |
trkResourceAtom = QTFindChildByID(container, compAtom, |
kQTMovieExportTrackInfoResourceType, 1, nil); |
if (trkResourceAtom) { |
QTMovieExportSourceRecord **trkResource = (QTMovieExportSourceRecord**) |
NewHandle(0); |
long j; |
Boolean wantsSound = false, wantsVideo = false, wantsText = false, |
wantsMIDI = false; |
QTCopyAtomDataToHandle(container, trkResourceAtom, (Handle)trkResource); |
for (j=0; j<(**trkResource).count; j++) { |
OSType mType = (**trkResource).sourceArray[j].mediaType; |
long flags = (**trkResource).sourceArray[j].flags; |
wantsSound = wantsSound || |
((mType == SoundMediaType) && (flags & |
kQTMovieExportSourceInfoIsMediaType)); |
wantsVideo = wantsVideo || |
(((mType == VideoMediaType) && (flags & |
kQTMovieExportSourceInfoIsMediaType)) || |
((mType == VisualMediaCharacteristic) && (flags & |
kQTMovieExportSourceInfoIsMediaCharacteristic))); |
wantsText = wantsText || |
((mType == TextMediaType) && (flags & |
kQTMovieExportSourceInfoIsMediaType)); |
wantsMIDI = wantsMIDI || |
((mType == MusicMediaType) && (flags & |
kQTMovieExportSourceInfoIsMediaType)); |
} |
if (wantsSound || wantsVideo) { |
ComponentDescription cd; |
char cc[5]; |
GetComponentInfo(c, &cd, nil, nil, nil); |
cc[0] = 4; |
*(long *)&cc[1] = cd.componentSubType; |
DebugStr((StringPtr)cc); |
} |
DisposeHandle((Handle)trkResource); |
} |
} |
QTDisposeAtomContainer(container); |
Using a Movie Data Export Component to Export Audio
Listing 5-2 illustrates how to use a movie data export component to export audio data to an AIFF file.
Listing 5-2 Exporting audio data to an AIFF file
ComponentDescription cd; |
MovieExportComponent ci; |
cd.componentType = MovieExportType; |
cd.componentSubType = 'AIFF'; |
cd.componentManufacturer = SoundMediaType; |
cd.componentFlags = canMovieExportFromProcedures; |
cd.componentFlagsMask = canMovieExportFromProcedures; |
ci = OpenComponent(FindNextComponent(nil, &cd)); |
Note that the componentManufacturer
field holds the
SoundMediaType
if the movie contains sampled sound or 'musi'
if it contains MIDI music. If you pass a zero in this field, QuickTime will use the first exporter that can create the desired type of output. This will not always produce the desired result, as a component that creates AIFF output from MIDI requires different input that a component that creates AIFF output from sampled sound.
Note that you use the canMovieExportFromProcedures
flag to limit the search to exporters that support the
MovieExportFromProceduresToDataRef
component call This is important since not all exporters implement this routine.
Configuring the Data Export Component
Once an AIFF movie data export component has been instantiated, it must be configured to open a single output audio stream. Listing 5-3 is an example of creating an output audio stream by calling MovieExportAddDataSource
. In this example, MovieExportAddDataSource
also provides the callback functions for supplying media data.
Listing 5-3 Configuring the audio export component
#define kMySampleRate 22050 |
#define kSoundBufferSize 1024 |
typedef struct |
{ |
Ptr soundData; |
SoundDescriptionHandle soundDescription; |
long trackID; |
} |
MyReferenceRecord; |
MyReferenceRecord myRef; |
SoundDescriptionPtr sdp; |
myRef.soundData = NewPtr(kSoundBufferSize); |
myRef.soundDescription = NewHandleClear(sizeof(SoundDescription)); |
sdp = *myRef.soundDescription; |
sdp->descSize = sizeof(SoundDescription); |
sdp->dataFormat = k8BitOffsetBinaryFormat; |
sdp->numChannels = 1; |
sdp->sampleSize = 8; |
sdp->sampleRate = kMySampleRate << 16; |
MovieExportAddDataSource(ci, SoundMediaType, kMySampleRate, |
&myRef.trackID, getSoundTrackPropertyProc, |
getSoundTrackDataProc, &myRef); |
On the Macintosh, the getSoundTrackPropertyProc and getSoundTrackDataProc routines should be universal procedure pointers (UPPs).
Exporting the Data
The export operation takes place when all of the required output tracks have been created. Typical code is shown in Listing 5-4.
Listing 5-4 Exporting from procedures to a data reference
StandardFileReply reply; |
Handle dataRef; |
// get output file from user |
QTNewAlias(&reply.sfFile, (AliasHandle *)&dataRef, true); |
// make up a data reference |
MovieExportFromProceduresToDataRef(ci, dataRef, rAliasType); |
MovieExportFromProceduresToDataRef
calls the two functions specified in MovieExportAddDataSource
to obtain data to generate the output file. The first function returns information about the output track’s properties, including the sample rate and supported media. If no value is returned for a particular property, the exporter specifies a default value based on the source data format. In the example in Listing 5-5, the output sample rate is set at 32000 Hz, with all other properties left unspecified.
Listing 5-5 Obtaining output track information
pascal OSErr getSoundTrackDataProc(void *refcon, long trackID, |
OSType propertyType, void *propertyValue) |
{ |
OSErr err = noErr; |
switch (propertyType) |
{ |
case scSoundSampleRateType: |
*(Fixed *)propertyValue = 32000L << 16; |
break; |
default: |
err = paramErr; |
break; |
} |
return err; |
} |
The second function provides data to be exported.
Listing 5-6 shows a block of sound data (silence) returned for each export request. The export operation ends when this function returns eofErr
.
Listing 5-6 Providing output track information to the export component
pascal OSErr getSoundTrack(void *refCon, |
MovieExportGetDataParams *params) |
{ |
MyReferenceRecord *myRef = (MyReferenceRecord *)refCon; |
if (params->requestedTime > kMySampleRate * 10) |
return eofErr; // end of data after 10 seconds |
params->dataPtr = myRef->soundData; |
params->dataSize = kSoundBufferSize; |
params->actualTime = params->requestedTime; |
params->sampleCount = kSoundBufferSize; |
params->durationPerSample = 1; |
params->descType = SoundMediaType; |
params->descSeed = 1; |
params->desc = (SampleDescriptionHandle)myRef->soundDescription; |
return noErr; |
} |
Using a Movie Data Export Component to Export Video
Using a movie data export component to create a QuickTime movie file is similar in many respects to creating an AIFF file, as shown in the previous example. Media data is handled differently in each case, however.
Instantiating the Video Export Component
Listing 5-7 illustrates the first step, instantiating the movie data export component for video data.
Listing 5-7 Instantiating a movie data export component
ComponentDescription cd; |
MovieExportComponent ci; |
cd.componentType = MovieExportType; |
cd.componentSubType = 'MooV'; |
cd.componentManufacturer = 'appl'; |
cd.componentFlags = canMovieExportFromProcedures; |
cd.componentFlagsMask = canMovieExportFromProcedures; |
ci = OpenComponent(FindNextComponent(nil, &cd)); |
Configuring the Video Export Component
Listing 5-8 illustrates the next step: configuring the data export component to create a single output video track. The call to MovieExportAddDataSource
provides the callback functions for supplying media data.
Listing 5-8 Configuring the movie data export component
#define kMySampleRate 2997 // 29.97 fps |
#define kMyFrameDuration 100 // one frame at 29.97 fps |
typedef struct |
{ |
GWorldPtr gw; // temporary GWorld we use during export |
ImageDescriptionHandle imageDescription; |
long trackID; |
} |
MyReferenceRecord; |
MyReferenceRecord myRef; |
myRef.gw = nil; |
myRef.imageDescription = nil; |
MovieExportAddDataSource(ci, VideoMediaType, kMySampleRate, |
&myRef.trackID, getVideoPropertyProc, |
getVideoDataProc, &myRef); |
Using the Component
The foregoing process should be repeated for as many video and sound sources as will be exported. However, it’s important to realize that not all exporters supporting export from procedures can export an arbitrary number of sources. For the case where an exporter supports fewer sources than the application needs to export, the application must precomposite the video sources or mix the sound sources before providing them to the exporter. The export code was shown previously in Listing 1-4.
The getVideoPropertyProc
function returns information about the output track’s properties (for example, the compression format). If the function doesn’t return a value for a particular property, the exporter will choose a default value based (usually) on the source data format. In Listing 5-9, dimensions are set to 160x120 and the compression format is set to JPEG. All other properties are unspecified.
Listing 5-9 Setting dimensions and compression format
pascal OSErr getVideoPropertyProc(void *refcon, long trackID, |
OSType propertyType, void *propertyValue) |
{ |
OSErr err = noErr; |
switch (propertyType) { |
case meWidth: |
*(Fixed *)propertyValue = 160L << 16; |
break; |
case meHeight: |
*(Fixed *)propertyValue = 120L << 16; |
break; |
case scSpatialSettingsType: |
{ |
SCSpatialSettings *ss = propertyValue; |
ss->codecType = kJPEGCodecType; |
ss->codec = 0; |
ss->depth = 0; |
ss->spatialQuality = codecNormalQuality; |
} |
break; |
default: |
err = paramErr; |
break; |
} |
return err; |
} |
The videoGetDataProc
function
provides video frames to the export operation. In the example in Listing 5-10, the same blank frame is returned for each request. The export operation ends when this function returns eofErr
. Any data allocated by videoGetDataProc
must
be disposed of after the export operation is complete.
Listing 5-10 Providing video frames for export
pascal OSErr getVideoDataProc(void *refCon, |
MovieExportGetDataParams *params) |
{ |
OSErr err = noErr; |
MyReferenceRecord *myRef = (MyReferenceRecord *)refCon; |
TimeRecord tr; |
if (params->requestedTime > kMySampleRate * 10) |
return eofErr;// end of data after 10 seconds |
if (!myRef->gw) { |
Rect r; |
CGrafPtr savePort; |
GDHandle saveGD; |
SetRect(&r, 0, 0, 320, 240); |
NewGWorld(&myRef->gw, 32, &r, nil, nil, 0); |
LockPixels(myRef ->gw->portPixMap); |
MakeImageDescriptionForPixMap(myRef->gw->portPixMap, |
&myRef->imageDescription); |
GetGWorld(&savePort, &saveGD); |
SetGWorld(myRef->gw, nil); |
EraseRect(&r); |
SetGWorld(savePort, saveGD); |
} |
params->dataPtr = GetPixBaseAddr(myRef->gw->portPixMap); |
params->dataSize = (**myRef->imageDescription).dataSize; |
params->actualTime = params->requestedTime; |
params->descType = VideoMediaType; |
params->descSeed = 1; |
params->desc = (SampleDescriptionHandle) |
myRef->imageDescription; |
params->durationPerSample = kMyFrameDuration; |
params->sampleFlags = 0; |
} |
Determining the Data Sources Supported by an Export Component
The number and kind of data sources supported varies from one exporter to another. In the foregoing examples, AIFF only supports sound data sources while the QuickTime movie exporter accepts both sound and video data sources. Furthermore, an exporter may require at least one data source of some type. In others, it may accept 0 to some maximum number of data sources of a particular type.
Because the kinds and number of data sources supported varies from exporter to exporter, and may change with new versions of a particular exporter, there needs to be a way for a client of an exporter to determine this information. In QuickTime, a procedure-supporting exporter has a public component resource that provides this information.
Copyright © 2005, 2006 Apple Computer, Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2006-01-10