Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Protocol
A protocol declares a programmatic interface that any class may choose to implement. Protocols make it possible for two classes distantly related by inheritance to communicate with each other to accomplish a certain goal. They thus offer an alternative to subclassing. Any class that can provide behavior useful to other classes may declare a programmatic interface for vending that behavior anonymously. Any other class may choose to adopt the protocol and implement one or more of its methods, thereby making use of the behavior. The class that declares a protocol is expected to call the methods in the protocol if they are implemented by the protocol adopter.
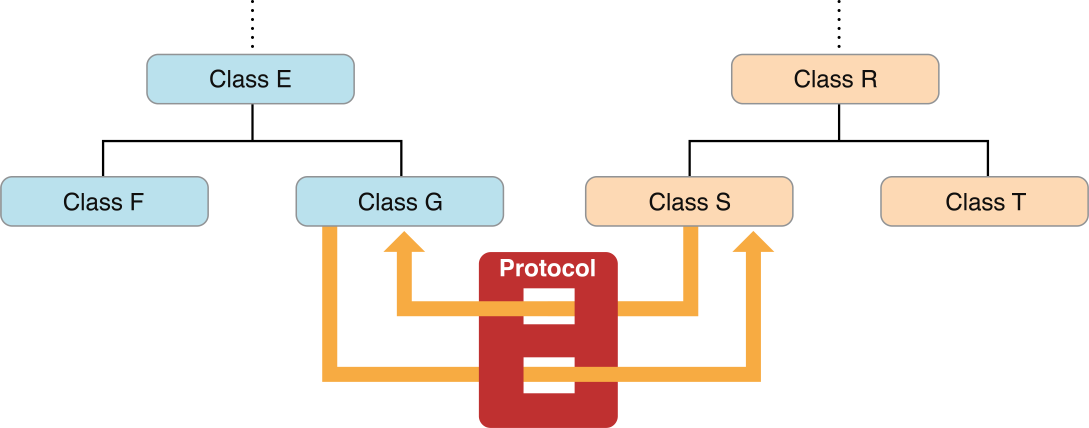
Formal and Informal Protocols
There are two varieties of protocol, formal and informal:
A formal protocol declares a list of methods that client classes are expected to implement. Formal protocols have their own declaration, adoption, and type-checking syntax. You can designate methods whose implementation is required or optional with the
@required
and@optional
keywords. Subclasses inherit formal protocols adopted by their ancestors. A formal protocol can also adopt other protocols.Formal protocols are an extension to the Objective-C language.
An informal protocol is a category on
NSObject
, which implicitly makes almost all objects adopters of the protocol. (A category is a language feature that enables you to add methods to a class without subclassing it.) Implementation of the methods in an informal protocol is optional. Before invoking a method, the calling object checks to see whether the target object implements it. Until optional protocol methods were introduced in Objective-C 2.0, informal protocols were essential to the way Foundation and AppKit classes implemented delegation.
Adopting and Conforming to a Formal Protocol
A class can either declare adoption of a formal protocol or inherit adoption from a superclass. The adoption syntax uses angle brackets in the @interface
declaration of the class. In the following example, the CAAnimation
class declares its superclass to be NSObject
and then formally adopts three protocols.
@interface CAAnimation : NSObject <NSCopying, CAMediaTiming, CAAction> |
A class—and any instance of that class—are said to conform to a formal protocol if the class adopts the protocol or inherits from another class that adopts it. Conformance to a protocol also means that a class implements all the required methods of the protocol. You can determine at runtime whether an object conforms to a protocol by sending it a conformsToProtocol:
message.
Creating Your Own Protocol
You may also declare your own protocol and implement the code that communicates with adopters of that protocol. For a description of how to do this, see the document that definitively describes protocols.
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-04-06