

Create custom classes
Now that you have the project set up, you're ready to begin writing the application. Start by writing the business logic, that is, the custom classes in Registration. The bulk of the work of Registration is done by its custom classes. Registration's components only manage the user interface.Registration has two custom classes: RegistrationManager and Person. RegistrationManager maintains the list of registrants and can add new users to that list. Person defines one user in the list of registrants. Its primary purpose is to validate the data that users enter.
Implement RegistrationManager
- In Project Builder, open Registration's project window.
- Choose File
New in Project.
- Make sure the Classes suitcase is selected, and then type RegistrationManager.java as the name of the class.
- Deselect the Create header option.
- Click OK.
- Declare RegistrationManager. Give it a single instance variable, registrants, which contains the list of registrants. Write a constructor to initialize the variable and an accessor method for the variable:
- Write a method that writes the registrants array to the People.array file:
- Write a method that adds a new person to the registrants array:
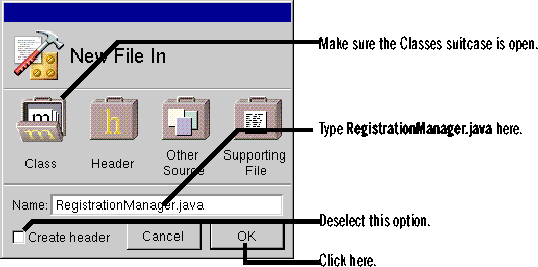
import next.util.*; import next.wo.*; import java.io.*; public class RegistrationManager extends Object { private protected MutableVector registrants; public RegistrationManager() { super(); String path; path = WebApplication.application().pathForResource("People", "array"); if (path != null) { registrants = new MutableVector(new File(path)); } else { registrants = new MutableVector(); } } public ImmutableVector registrants() { return registrants; } }
The list of registants is stored in a file named People.array. The instance variable registrants can be initialized from the file People.array because the file contains data in a property list format. A property list is a compound data type that consists of strings, arrays, dictionaries, and data. Property lists can be represented in an ASCII format, and property list objects such as dictionaries and arrays can consequently be initialized from ASCII files that use this format. The file People.array contains an array of dictionaries (or more explicitly, a MutableVector of ImmutableHashtables).
The constructor accesses the People.array file through the WebApplication method pathForResource. pathForResource takes a path and the file's extension as arguments:
path = WebApplication.application().pathForResource("People", "array");
You can use this method to load different kinds of resources into your application-for example, images, sound files, data files, and so on.
private void writeRegistrantsToFile(String path) { FileOutputStream file; DataOutputStream stream; byte b[]; String output; try { file = new FileOutputStream(path); stream = new DataOutputStream(file); output = registrants.toString(); b = new byte[output.length()]; output.getBytes(0, output.length(), b, 0); stream.write(b, 0, output.length()); stream.flush(); stream.close(); } catch (java.io.IOException e) { WebApplication.application().logString(e.getMessage()); } finally { } }
When a new person is added to the registrants array, RegistrationManager must update the People.array file. It does so with the writeRegistrantsToFile method. writeRegistrantsToFile sends the toString message to registrants, which returns the contents of the array in a property list format.
public ImmutableHashtable registerPerson(Person newPerson) { int i; ImmutableHashtable results; String currentName; String newPersonName = newPerson.name(); String path = WebApplication.application().pathForResource("People", "array"); if (path == null) { /* Create People.array if it doesn't exist. */ path = WebApplication.application().path() + File.separator + "People.array"; } results = newPerson.validate(); if (((String)(results.get("valid"))).compareTo("No") == 0) { return results; } if (registrants.isEmpty() == false) { for (i = registrants.size() - 1; i >= 0; i--) { currentName = ((String)(((ImmutableHashtable) (registrants.elementAt(i))).get("name"))); if (currentName.compareTo(newPersonName) == 0) { registrants.removeElementAt(i); break; } } } registrants.addElement(newPerson.personAsDictionary()); writeRegistrantsToFile(path); return results; }
registerPerson is invoked when the user clicks the Register button on the application's Main page. You'll create the Main page later. registerPerson first uses WebApplication's pathForResource to access the People.array file. If the file does not yet exist, it creates the file. Next, it makes sure that the Person object to be added contains valid information. If so, it adds that Person to the registrants array. If the Person is already in the registrants array, it removes that entry so that the list is effectively updated with the new information for that person. Finally, writeRegistrantsToFile is invoked to update the People.array file.
Table of Contents
Next Section