Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Graphics Exporter Components
This chapter describes the general features of graphics exporter components.
Applications make direct calls to graphic exporter components, so this chapter will be of interest to most QuickTime developers who work with still images or the web. Much of the conceptual information found in Graphics Importer Components also applies to exporters. You should be familiar with that chapter before reading this chapter.
Applications programmers should also be familiar with the Component Manager before working with graphics exporter components.
If you would like to create a new graphics exporter component, refer to this chapter to implement a component that supports the required interface functions.
Exporting Graphics
Graphics exporter components provide a standard interface for exporting graphics to image files in a variety of formats. QuickTime selects a graphic exporter component based on the desired output format, such as GIF or PNG. Different image formats support a wide range of configurable features, such as depth, resolution and compression quality.
QuickTime supports the following export image formats: BMP, JFIF, JPEG, GIF, MacPaint, Photoshop, PNG, QuickDraw PICT, QuickTime Image, Silicon Graphics, Targa, and TIFF. Note that this list is not inclusive; QuickTime supports additional export image formats with each software release.
A graphic image can be exported to a handle, a file, or a data reference.
Third-party developers may also write their own graphics exporters for other image file formats.
Using Graphics Exporter Components
This section describes how to use graphics exporter components. Code samples are included.
To export an image to a file or a data reference using a graphics exporter, you must open a graphics exporter component instance, specify the source of the input image and the destination for the output image file, set the parameters you want, and call GraphicsExportDoExport
.
You select a particular graphics exporter component based on the desired output format, such as GIF or PNG. If you know which file format you want to write, you can use a call such as OpenADefaultComponent
to open the appropriate exporter.
The input image can come from a QuickDraw Picture
, a GWorld
or PixMap
, a QuickTime graphics importer component instance, or a segment of compressed data described by a QuickTime image description. In the last case, the compressed data may be accessed via a pointer, handle, file or other data reference.
The output image for an export operation can be specified as a handle, a file, or a data reference.
When you are done with the graphics exporter component instance, call CloseComponent
.
The example code in Listing 3-1 shows the basic functions you use to export a GWorld
to an image file. Error-checking code has been removed.
Listing 3-1 The basic functions used to export a GWorld to an image file
void writeGWorldToImageFile( GWorldPtr gw, const FSSpec *fileSpec ) |
{ |
GraphicsExportComponent ge = 0; |
OpenADefaultComponent( GraphicsExporterComponentType, |
kQTFileTypePNG, &ge ); |
GraphicsExportSetInputGWorld( ge, gw ); |
GraphicsExportSetOutputFile( ge, fileSpec ); |
GraphicsExportDoExport( ge, nil ); |
CloseComponent( ge ); |
} |
Selecting a Graphics Exporter Component
Graphics exporter components have component type 'grex'
and, by convention, component subtype matching the Mac OS file type for the image file format. For example, the graphics exporter for PNG files has component subtype 'PNGf'
.
If you know which file format you want to write, you can use a call such as OpenADefaultComponent
to open the appropriate exporter.
If you want to display a list of graphics exporter components and let the user select one, you can build a list by using FindNextComponent
to search for graphics exporter components and calling GetComponentInfo
on each to find out its name, as shown in Listing 3-2.
Take care not to offer the base exporter as an option. An easy way to do this is to restrict your search to those graphics exporter components that do not have the graphicsExporterIsBaseExporter
component flag set.
Listing 3-2 Building a list of graphics exporter components
void findGraphicsExporterComponents() |
{ |
ComponentDescription cd; |
Component c = 0; |
cd.componentType = GraphicsExporterComponentType; |
cd.componentSubType = 0; |
cd.componentManufacturer = 0; |
cd.componentFlags = 0; |
cd.componentFlagsMask = graphicsExporterIsBaseExporter; |
while( ( c = FindNextComponent( c, &cd ) ) != 0 ) { |
// add component c to the list. |
} |
} |
Specifying the Source
The input image for an export operation can come from a QuickDraw Picture, GWorld
or PixMap
, or a QuickTime graphics importer instance. To specify such a source, call the respective function:
GraphicsExportSetInputPicture |
GraphicsExportSetInputGWorld |
GraphicsExportSetInputPixMap |
GraphicsExportSetInputGraphicsImporter |
Alternatively, the input image may be a segment of compressed data described by an image description. To specify such a source, call one of these functions:
GraphicsExportSetInputDataReference |
GraphicsExportSetInputFile |
GraphicsExportSetInputHandle |
GraphicsExportSetInputPtr |
In this case, the compressed data may comprise only a segment of the whole data reference. You may limit access to a segment by calling the GraphicsExportSetInputOffsetAndLimit
.
Specifying the Destination
The output image for an export operation can be written to a handle, file, or other data reference. To specify the destination, call the respective function:
GraphicsExportSetOutputDataReference |
GraphicsExportSetOutputFile |
GraphicsExportSetOutputHandle |
By default, the graphics exporter’s suggested file type and creator will be used for any newly created file. To override the suggested file type or creator, call GraphicsExportSetOutputFileTypeAndCreator
.
Specifying Export Settings
A variety of GraphicsExportSet
... and GraphicsExportGet
... functions are available to set and get various settings. Not every graphics exporter supports all of these.
As usual, if you call an unimplemented component function, it will return badComponentSelector
. You can find out whether a particular component function is implemented by a component with the CallComponentCanDo
function.
DontRecompress Flag
Repeated compression of an image with a lossy format (such as JPEG) can cause image degradation.
The DontRecompress
flag is used to request that the original compressed data not be decompressed and recompressed, but be copied (possibly with modifications) through to the output file. This is not always possible; circumstances may still force a graphics exporter to recompress.
In QuickTime, the JPEG, PICT and QuickTime Image graphics exporters support the DontRecompress
flag.
Interlace Styles
Some image formats support interlacing, in which image data is rearranged in some manner. A common use for this is in the PNG and GIF formats, which rearrange data so that low-resolution images can be displayed from incomplete data streams.
In QuickTime, the PNG graphics exporter supports the InterlaceStyle
settings kQTPNGInterlaceNone
and kQTPNGInterlaceAdam7
.
MetaData
Some image file formats can contain supplemental data, such as textual copyright information.
In QuickTime, none of the supplied graphics exporters support setting MetaData
.
Compression Methods
Some image file formats support more than one compression algorithm. In QuickTime, the TIFF graphics exporter supports the CompressionMethod
settings kQTTIFFCompression_None
and kQTTIFFCompression_PackBits
.
Compression Quality
Lossy image compression involves a quality-versus-size tradeoff: the higher the image quality, the greater the compressed data size.
In QuickTime, the JPEG, PICT and QuickTime Image graphics exporters support the CompressionQuality
setting. The PICT and QuickTime image graphics exporters pass it on as a parameter to a compressor.
Target Data Size
An alternative way to view the quality-versus-size tradeoff is to specify a desired maximum data size and ask for a quality that does not exceed that size.
In QuickTime, the JPEG graphics exporter supports the TargetDataSize
setting, which is implemented by compressing the image repeatedly, lowering the quality until the target size (or a maximum attempt limit) is reached.
Resolution
Some image file formats can store the image resolution.
In QuickTime, the BMP, JPEG, Photoshop, PNG, QuickTime Image, and TIFF graphics exporters support the resolution
setting. If you do not set a resolution explicitly, the original image’s resolution is used.
Depth
Some image file formats support more than one pixel depth.
In QuickTime, the BMP, JPEG, Photoshop, PNG, PICT, QuickTime Image, Targa and TIFF graphics exporters support the depth
setting.
Note that the usual QuickDraw conventions for bit depths apply:
24 means “Millions of Colors”
32 means “Millions+,” meaning millions of colors plus an alpha channel
40 means 8-bit grayscale.
ColorSyncProfile
Some image file formats support embedded ColorSync profiles. This allows image files to describe their native colorspace in a self-contained manner. In QuickTime, the JPEG, PNG, PICT, QuickTime Image and TIFF graphics exporters support embedded ColorSync profiles.
Settings Dialogs
You can access all of a graphics exporter’s settings at once with the GraphicsExportSetSettingsFromAtomContainer
and GraphicsExportGetSettingsAsAtomContainer
functions. These functions can also be used to access other settings besides those in the list above.
User Interface
Some graphics exporters can display a dialog to let the user configure format-specific settings. To display such a dialog, call the GraphicsExportRequestSettings
function.
In QuickTime, the BMP, JPEG, Photoshop, PNG, PICT, QuickTime Image and TIFF graphics exporters support settings
dialogs.
You can get a user-readable description of a graphics exporter’s current settings by calling the GraphicsExportGetSettingsAsText
function.
File Type and Creator
To find out the suggested file name extension for a graphics exporter’s format, call GraphicsExportGetDefaultFileNameExtension
.
To find out the suggested Mac OS file type and creator, call GraphicsExportGetDefaultFileTypeAndCreator
. To find out the MIME type, call GraphicsExportGetMIMETypeList
.
How Graphics Exporters Work
QuickTime provides a base graphics export component which provides abstractions that greatly simplify the work of format-specific graphics exporter components, while offering applications a rich interface.
Format-specific graphics exporters, such as the exporters for JPEG, PNG and TIFF, are relatively simple components. When a format-specific exporter is opened, it opens and targets an instance of the base graphics exporter. Subsequently, it delegates most of its calls to the base exporter instance, as shown in Figure 3-1.
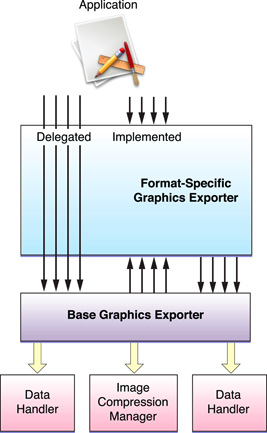
The base exporter communicates with data handler components to write image file data. If necessary, it calls the Image Compression Manager to perform compression operations.
There are three modes that format-specific exporters can operate in:
Transcode. Data is transferred from one image file to another without decompressing and recompressing. (The data may be modified along the way, if appropriate.)
Using a Compressor. The graphics exporter provides an atom container identifying which compressor to use, and any settings to be passed to that compressor.
Standalone Export. The graphics exporter component does all the work itself, without using an image compressor.
How the Base Exporter Chooses a Mode
When an application calls GraphicsExportDoExport
, the base exporter asks the following question:
Can QuickTime transcode?
It calls the format-specific exporter’s GraphicsExportCanTranscode
function. If the answer is yes, it calls the exporter’s GraphicsExportDoTranscode
function.
Otherwise,
Can QuickTime use a compressor?
It calls the format-specific exporter’s GraphicsExportCanUseCompressor
function.
If the answer is yes, it calls the Image Compression Manager to compress using the compressor and parameters specified in the atom container. This is done in the base exporter’s GraphicsExportDoUseCompressor
function. Usually the format-specific exporter does not need to override this function and should simply delegate it. If the format-specific exporter’s format is a container format (such as a PICT or QuickTime Image), it can override and delegate this in order to encapsulate the compressed data in the container format.
Otherwise,
QuickTime must perform a standalone export.
If neither transcoding nor compressing is appropriate, it calls the format-specific exporter’s GraphicsExportDoStandaloneExport
function.
Writing Graphics Export Components
If you’re interesting in writing a component, you should check out the sample code at
http://developer.apple.com/samplecode/ElectricImageComponent/ElectricImageComponent.html |
The sample code demonstrates how to build five QuickTime Components: a graphics importer, graphics exporter, movie importer, movie exporter and image decompressor.
These components all work together to allow QuickTime to use the Electric Image format image files. Sample Electric Image files are included.
Copyright © 2005, 2006 Apple Computer, Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2006-01-10