Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Generating Controllers With the Controller Factory
Much of the magic behind Direct to Java Client applications happens in the controller factory, the class com.webobjects.eogeneration.EOControllerFactory
. The purpose of the class is to produce controllers—windows, dialogs, list controllers, select controllers, controllers for particular tasks, and so on. By learning how to use the controller factory programmatically, you can take greater control of Direct to Java Client applications—you can learn to be the magician.
Selecting Objects in an Entity
Problem: You need a user interface and logic to provide a way for users to select an object or objects from a particular table in the data store.
Solution: Use the controller factory to get a select controller for a particular entity.
If you tackle this task without using the rule system, you could spend an hour or more in Interface Builder building the user interface and connecting it to a custom controller class to get the selected objects and pass them on to the requesting object. But by using the rule system and the controller factory, a single method invocation does all of this for you.
In a client-side view class (such as the CustomFormController class in Extend a Controller Class or a subclass of another core controller class), add the import statement for com.webobjects.eogeneration
. This package contains the controller factory. Then, in the action method that triggers the selection, add this invocation on the controller factory:
EOControllerFactory.sharedControllerFactory().selectWithEntityName("
entityName", true, false);
The method takes three arguments: the entity to select from, a Boolean value determining whether multiple selections are allowed, and a Boolean value representing whether the insertion of new records is allowed (if the dialog provides an action to add new records). When invoked, the method presents a select dialog like that shown in Figure 12-1.
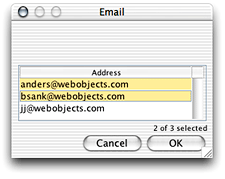
The method returns an array of EOGlobalID objects representing the selected objects. To get enterprise objects from EOGlobalID objects, you can use the method objectForGlobalID
defined in com.webobjects.eocontrol.EOEditingContext
. See the API reference for more information.
Triggering a Task
Problem: You need to provide a custom task to perform some function in the application. You need a way to trigger this task.
Solution: Write a task using a rule and trigger it with an invocation on the controller factory.
Suppose that you have a frozen XML interface in your application. There is no method in the controller factory to simply invoke this frozen interface. But you can easily define a task to do this.
If the frozen XML component is called ImageQueryController, you would define the new task like this:
- Left-Hand Side:
(task ='imageQuery')
- Key:
window
- Value:
"ImageQueryController"
- Priority:
50
In a client-side view class (not a model class or a controller class), add the import statement for com.webobjects.eogeneration
. This package contains the controller factory. Then, in the action method that triggers the selection, add this invocation on the controller factory:
EOControllerFactory.sharedControllerFactory().openWindowForTaskName("imageQuery"); |
Inserting Objects
Problem: You need to provide a form window for a particular task so a user can insert new records into a table.
Solution: Use the controller factory to get a form controller for a particular entity.
If you implement this feature without using the controller factory or the rule system, you could spend an hour or more in Interface Builder building the interface, connecting the controller, and then writing code to invoke the interface. But by using the rule system and the controller factory, a single method invocation does all of this for you.
In a client-side view class (not a model class or a controller class), add the import statement for com.webobjects.eogeneration
. This package contains the controller factory. Then, in the action method that triggers the selection, add this invocation on the controller factory:
EOControllerFactory.sharedControllerFactory().insertWithEntityName("Document"); |
This method simply takes the name of an entity in the enterprise object model group of your application. It results in a form window like that shown in Figure 12-2.
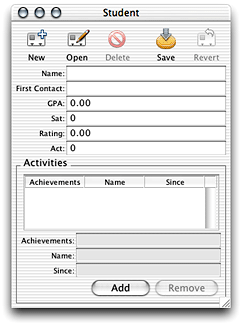
Copyright © 2002, 2005 Apple Computer, Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2005-08-11