Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Web Services Overview
You can think of Web services as distributed applications. Instead of creating an instance of a class and invoking its methods, a Web service consumer locates a Web service and invokes the operations it provides. The Web service provider (the application implementing the Web service) can be on the same Java virtual machine as the one using it, or it can be thousands of miles away. Furthermore, the applications may be written in different languages and running in disparate platforms. Because of this, Web service consumers as well as Web service providers need a way of transferring information that is language and platform independent. This is where SOAP lends a hand.
Web services are based on SOAP (Simple Object Access Protocol). It provides an infrastructure for the exchange of structured data in a distributed environment. SOAP itself is based on XML (Extensible Markup Language). XML is an SGML (Standard Generalized Markup Language)-based language that facilitates the structuring of data in documents. In addition, data elements in XML documents provide information about the data they contain through element names and attributes.
SOAP was created to facilitate the exchange of information by heterogeneous systems. XML provides it with structure through schemas and element scope through namespaces. SOAP is a transport-agnostic protocol: messages can be sent using HTTP, SMTP, and other protocols. For more on XML, including XML Schema and XML Namespaces, see Extensible Markup Language (XML) at http://www.w3.org/XML .
This chapter introduces Web service concepts. If you're familiar with Web service technology, you can go to the next chapter.
The chapter has the following sections:
What Are Web Services?
Web services provide an implementation-independent way for applications to communicate with each other. Currently, many companies use electronic-data-interchange (EDI) systems to communicate with their business partners. EDI, however, requires the use of slow modems and dedicated phone lines. Also, a change in the structure of the data exchanged requires that the systems of all partners involved be updated. Web services, which are based on SOAP messages that wrap XML documents, provide a flexible infrastructure that leverages the ubiquitous HTTP (or HTTPS) over TCP/IP. This means that your organization probably has all the hardware and software infrastructure needed to deploy Web services already. In addition, thanks to XML's structure and flexibility, each partner can extract only the information it needs from a message, which gives participants a great deal of freedom.
But Web services provide more than an information-exchange system. When an application implements some of its functionality using Web services, it becomes more than the sum of its parts. For example, you can create a Web service operation that uses a Web service operation from another provider to give its consumers (also known as service requestors ) information tailored to their needs. Web service operations are akin to the methods of a Java class; a provider is an entity that publishes a Web service, while the entities that use the Web service are called consumers.
Current Web service technology allows an organization to easily integrate its systems, creating an enterprise-wide solution that leverages the work that is performed best by smaller groups within the enterprise. For example, the Payroll system is the one that should deal with an employee's compensation, while the Human Resources system is more appropriate for the management of vacation and sick-leave time. However, an Employee Information system should gather the information that both the Payroll and Human Resources systems contain, but should not duplicate it. The Employee Information system could display a window or Web page that an employee can view to analyze both her salary and accrued vacation time, without having to directly access the data stores used by the other two systems. Payroll and vacation information would be available through Web service operations provided by separate applications tailored to their particular objectives.
Web services can also be deployed over the Internet; however, you should ensure that sensitive information is not compromised. A SOAP message can hop through several computers across a network before reaching its destination, which exposes it to be viewed and modified by entities that you don't know about. There are several standards and specifications that help you protect the messages you send and to make sure that the messages you receive have not been compromised. See Security in Web Services for more information.
What Web services really provide is access to business logic. This business logic can be implemented in any language. Most companies implementing Web services for the first time only add a Web service front end to their existing applications. WebObjects makes this easy.
Web Service Discovery
The Web Services Description Language (WSDL) is an XML-based language used to describe a Web service. This description allows an application to dynamically determine a Web service's capabilities; for example, the operations it provides, their parameters, return values, and so forth. A UDDI (Universal Description, Discovery and Integration) repository is a searchable directory of Web services that Web service requestors can use to search for Web services and obtain their WSDL documents. WSDL documents, however, do not need to be published in a repository for consumers to take advantage of them. You can obtain a WSDL document through a Web page or an email message.
Web Services and SOAP
SOAP is the messaging mechanism that you use when you consume Web service operations or provide Web service operations to your clients.
All Web service communication is done through SOAP messages. These messages have an envelope, represented by the Envelope
element, and a body, enclosed by the Body
element, containing the message's content. In addition, the Envelope
can contain a Header
element enclosing one or more header entries. The header mechanism is what provides SOAP with decentralized extensibility; this is how extensions such as Digital Signature and Web Services Security Core Language (WSS-Core) are implemented. For more information on signatures and security, see Security in Web Services
.
SOAP provides two ways for representing Web service operation invocations: RPC (Remote Procedure Call) messaging and document-style messaging. RPC messaging provides a way of representing method invocations in SOAP messages. Because of this, however, the structure of the messages representing operation invocations is fairly rigid. Document-style messaging, on the other hand, provides greater flexibility; it allows messages to contain arbitrary data elements. However, parsing such messages is more complicated.
Direct to Web Services (a technology that allows you to rapidly create Web services based on a data model) uses RPC because it allows the mapping of entity attributes to operation parameters. However, you can use either RPC messaging or document-style messaging in the Web services that you write. Take into account that document-style messaging requires specialized processing to extract the necessary data from the message. You must also implement error processing in case required data elements are not present in a message.
Ingredients of a SOAP Message
As Figure 1-1
shows, a SOAP message, represented by the Envelope
element, contains a mandatory Body
element and an optional Header
element. The Body
element can contain a number of body entries. The optional Fault
element is present only in messages that report a processing exception.
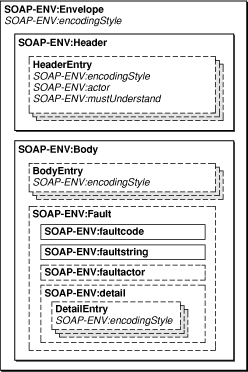
Listing 1-1 shows an RPC SOAP message.
Listing 1-1 Example of an RPC SOAP message
<soapenv:Envelope |
xmlns:soapenv="soap_ns" |
xmlns:xsd="xml_schema_ns" |
xmlns:xsi="type_ns"> |
<soapenv:Body> |
<ns1:getStockPrice |
xmlns:ns1="app_ns" |
soapenv:encodingStyle="encoding_ns"> |
<stockSymbol xsi:type="xsd:string">AAPL</stockSymbol> |
</ns1:getStockPrice> |
</soapenv:Body> |
</soapenv:Envelope> |
Listing 1-2 shows a document-style SOAP message.
Listing 1-2 Example of a document-style SOAP message
<soapenv:Envelope |
xmlns:soapenv="soap_ns" |
xmlns:xsd="xml_schema_ns" |
xmlns:xsi="type_ns"> |
<soapenv:Body> |
<ns1:customerOrder |
soapenv:encodingStyle="encoding_ns" |
xmlns:ns1="app_ns"> |
<order> |
<customer> |
<name>Plastic Pens, Inc.</name> |
<address> |
<street>123 Yukon Drive</street> |
<city>Phoenix</city> |
<state>AZ</state> |
<zip>85021</zip> |
</address> |
</customer> |
<orderInfo> |
<item> |
<partNumber>88</partNumber> |
<description>Blue pen</description> |
<quantity>250</quantity> |
</item> |
<item> |
<partNumber>563</partNumber> |
<description>Red stappler</description> |
<quantity>30</quantity> |
<item> |
</orderInfo> |
</order> |
</ns1:customerOrder> |
</soapenv:Body> |
</soapenv:Envelope> |
Table 1-1 describes the elements of a SOAP message.
Element |
Parent |
Use |
Description |
---|---|---|---|
|
None |
1 |
Root element of the message. |
|
|
? |
Encloses header entries. |
Header entries |
|
* |
Heather entries provide additional information on the message's content. For example, digital signatures, authorization data, and so on. |
|
|
1 |
Encloses the message's body entries. |
Body entries |
|
* |
Body entries make up the content of the message. Their element names depend on the message's content. |
|
|
? |
Body entry used to report a problem. When used, no other body entry can be present. |
|
|
1 |
Indicates the reason for the fault. Intended for application use. |
|
|
1 |
Human-readable version of the fault reason. |
|
|
? |
Indicates which entity along the message path raised the fault. |
|
|
? |
Encloses detail entries. |
Detail entries |
|
* |
Contain application-specific information about the fault. |
All the attributes that the SOAP envelope schema defines are global (they are not associated with a particular element). Also, each element in a SOAP message is free to use any attribute, regardless of where it's defined, either in SOAP's schema or another one, which is one of SOAP's extensibility features. This means that elements are free to use any number of attributes. Table 1-2 describes the attributes that the SOAP specification defines.
Attribute |
Value |
Description |
---|---|---|
|
A URI. |
Specifies the entity that is to process the element. When absent, the actor is the ultimate recipient of the message. This attribute is used mainly to assign header entries to specific entities. |
|
|
Indicates whether the element's actor must process the element. When set to |
|
A list of URIs. |
Indicates the encoding style used for the element's content. |
For more information on SOAP, see Simple Object Access Protocol (SOAP) at http://www.w3.org/TR .
Web Service Description
For a consumer to be able to use a Web service's operations, it must know what operations the Web service provides, the parameters they take, the type of the values they return, and so on. With intimate knowledge of the Web service, you can write a Web service client that takes full advantage of the service. However, the idea behind Web services is to provide a way for an application to dynamically find a Web service that satisfies its requirements and to learn how to use it. One of the building blocks that bring that vision closer to reality is WSDL (Web Services Description Language). Like SOAP, WSDL is an XML-based language. A WSDL document tells a service requestor where a Web service is located and how to use it.
A WSDL document describes Web services in two ways: an abstract description or interface and a concrete implementation. The interface section provides a high-level description of the operations the Web services described by the document provide and their parameter types and return types. The implementation section binds each operation described in the interface section with its implementation (the methods that perform the work).
These are some of the XML elements that WSDL defines to describe Web services:
-
portType
: This element provides the interface to one or more Web services. It describes each operation provided by the services as a set of input (from the consumer) and output (from the provider) messages that can be generated as a result of invoking the operation. Included in the list of possible messages are fault messages, which the Web services way of notifying the occurrence of a processing problem or exception. -
message
: This element describes a SOAP message. It lists the message's elements, which are referred to as parts , and their types. -
types
: This element list all the data types used as parameters or return types used inmessage
elements. Essentially, it's an XML Schema definition. -
binding
: This element specifies the transport used to send messages between the Web services' consumers and their provider and implements aportType
. It also defines the type of encoding used for each message. -
port
: This element defines the URL that the Web service consumers use to access the Web service. It implements abinding
. -
service
: This element encloses one or moreport
elements.
Figure 1-2
shows the relationship between the major elements of a WSDL document. Missing from the figure are the definitions
element, which is the root element of WSDL document and the types
element.
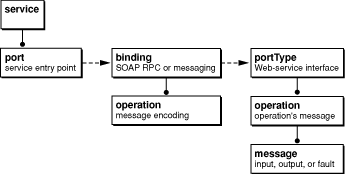
For the most part, you don't have to concern yourself with reading or writing WSDL documents. WebObjects generates the WSDL documents needed to provide Web services and makes available methods to access the information contained in the WSDL documents for Web services you want to consume. For more information on WSDL, see Web Services Description Language (WSDL) at http://www.w3.org/TR .
SOAP Engine
A SOAP engine (or processor) aids both consumers of Web services and their providers to accomplish their task without having to worry about the intricacies of SOAP message handling. As far as the consumer is concerned, it invokes an operation in a similar way a remote procedure call is invoked. The Web service provider needs to implement only the logic required by the business problem it solves. The consumer's SOAP processor converts the method invocation into a SOAP message. This message is transmitted through a transport, such as HTTP or SMTP, to the service provider's SOAP processor, which parses the message into a method invocation. The provider then executes the appropriate logic and gives the result to its SOAP processor, which parses the information into a SOAP response message. The message is transmitted through a transport to the consumer. It's SOAP processor parses the response message into a result object that it returns to the invoking entity.
Axis is the third generation of Apache SOAP (an implementation of SOAP from the Apache Software Foundation). Axis is a SOAP engine as well as a code generator and WSDL processing tool. WebObjects uses Axis to both provide and consume Web services.
The idea behind Axis is to serve as a bridge between your time-tested code and the world of Web services. By using Axis as its SOAP engine, WebObjects allows you to leverage the business logic you have already created and use it as the backbone of your Web services strategy.
Axis processes SOAP messages using a series of handlers , which are classes responsible for processing a message or part of a message in a certain way. In fact, you are free to add your own handlers to customize message processing. For more information on Axis, visit http://xml.apache.org/axis .
The Axis SOAP Engine
WebObjects uses the Axis framework to both serve and consume Web services. Axis is an interface between your business logic and the Web services world.
The Axis Web service processing model is shown in Figure 1-3 .
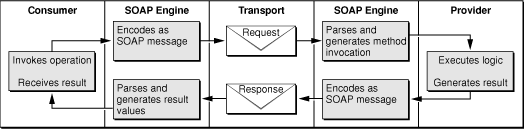
Axis implements a very extensible message processing model. It uses handlers and handler chains to allow its functionality to be tailored to a wide variety of situations and requirements. A handler is an atomic component that acts on a specific part of a SOAP message; for example, a handler can be in charge of performing authentication on the message's sender before allowing it to be processed by the provider. A special handler, the pivot handler (another name for the service's provider), is in charge of executing the Web service's logic. It's called pivot handler because it is where the message's processing cycle changes from request processing to response processing.
A handler chain is a group of handlers that can be viewed as a unit. An important concept to grasp is that handlers and handler chains are not Web service–specific. For example, you can develop handlers that process SOAP messages from transports other than HTTP or SMTP to increase security without having to change the Web service implementation. If you start now, you may be able to sell those handlers to others for a nice profit.
Handlers are simply Java classes that act on an org.apache.axis.MessageContext
object. A MessageContext contains several useful objects, but the most important are the requestMessage
and the responseMessage
. Handlers processing incoming messages normally access the requestMessage
object, while those processing the outgoing messages access the responseMessage
object. However, Axis provides no restrictions; a handler can access and modify whatever it pleases. This is helpful if you need a handler to act both on incoming and outgoing messages.
Figure 1-4 shows the relationship between handlers and chains in Axis from the point of view of a Web service provider, while Figure 1-5 does the same from the perspective of a consumer.
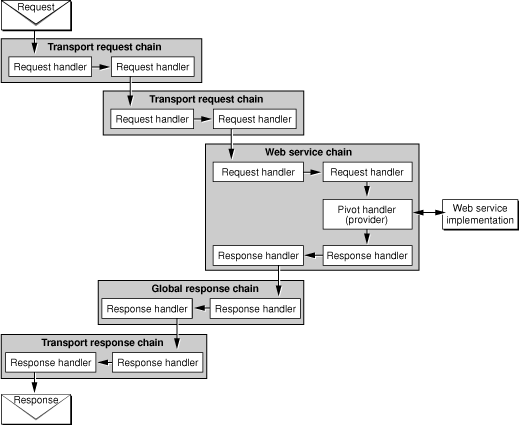
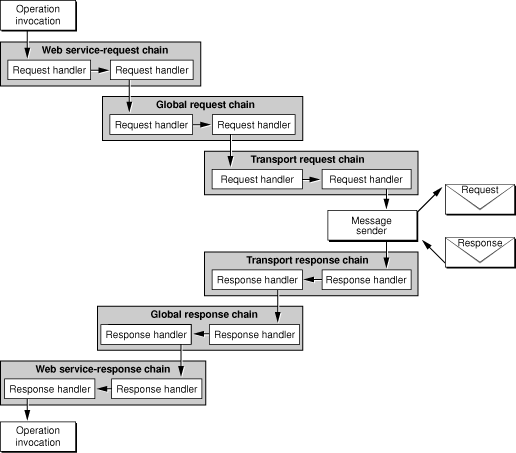
Notice that there are three types of chains: transport, global, and Web service. A transport chain can deal with issues specific to the transport used to send and receive SOAP messages. A global chain is one that processes every SOAP message, regardless of the transport used or the target Web service. Finally, a Web service chain is one tailored for a specific Web service. For more information on handlers and chains, see Axis's documentation at http://xml.apache.org/axis .
Serialization and Deserialization of Objects
Complex classes require a custom serialization and deserialization strategy. WebObjects provides serializers and deserializers for some of its classes, as shown in Table 1-3 .
Class |
Serializer |
Deserializer |
---|---|---|
|
x |
x |
|
x |
x |
|
x |
|
|
x |
x |
|
x |
|
|
x |
x |
|
x |
x |
|
x |
|
|
x |
|
|
x |
x |
|
x |
x |
|
x |
If you have special classes that require a special serializer and deserializer, you have to create them. Writing serializer and deserializer classes is a simple process, but requires knowledge of SAX (Simple API for XML). To learn how to process SAX callbacks in your serializers and deserializers, see Java & XML (O'Reilly).
Copyright © 2002, 2007 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2007-07-11