About Document-Based Applications in iOS
The UIKit framework offers support for applications that manage multiple documents, with each document containing a unique set of data that is stored in a file located either in the application sandbox or in iCloud.
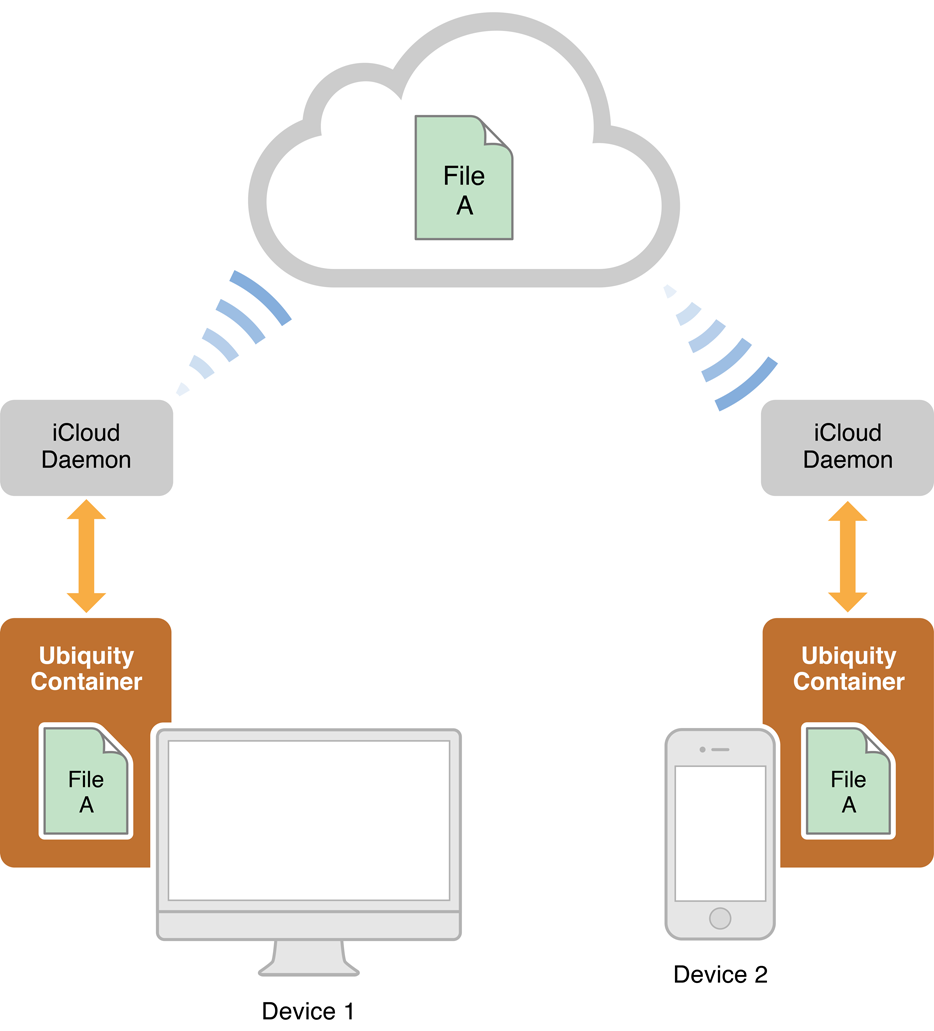
Central to this support is the UIDocument
class, introduced in iOS 5.0. A document-based application must create a subclass of UIDocument
that loads document data into its in-memory data structures and supplies UIDocument
with the data to write to the document file. UIDocument
takes care of many details related to document management for you. Besides its integration with iCloud, UIDocument
reads and writes document data in the background so that your application’s user interface does not become unresponsive during these operations. It also saves document data automatically and periodically, freeing your users from the need to explicitly save.
At a Glance
Although a document-based application is responsible for a range of behaviors, making an application document-based is usually not a difficult task.
Document Objects Are Model Controllers
In the Model-View-Controller design pattern, document objects—that is, instances of subclasses of UIDocument
—are model controllers. A document object manages the data associated with a document, specifically the model objects that internally represent what the user is viewing and editing. A document object, in turn, is typically managed by a view controller that presents a document to users.
Relevant Chapter: Designing a Document-Based Application
When Designing an Application, Consider Document-Data Format and Other Issues
Before you write a line of code you should consider aspects of design specific to document-based applications. Most importantly, what is the best format of document data for your application, and how can you make that format work for your application in iOS and Mac OS X? What is the most appropriate document type?
You also need to plan for the view controllers (and views) managing such tasks as opening documents, indicating errors, and moving selected documents to and from iCloud storage.
Relevant Chapters: Designing a Document-Based Application, Document-Based Application Preflight
Creating a Subclass of UIDocument Requires Two Method Overrides
The primary role of a document object is to be the “conduit” of data between a document file and the model objects that internally represent document data. It gives the UIDocument
class the data to write to the document file and, after the document file is read, it initializes its model objects with the data that UIDocument
gives it. To fulfill this role, your subclass of UIDocument
must override the contentsForType:error:
method and the loadFromContents:ofType:error:
method, respectively.
Relevant Chapter: Creating a Custom Document Object
An Application Manages a Document Through Its Life Cycle
An application is responsible for managing the following events during a document’s lifetime:
Creation of the document
Opening and closing the document
Monitoring changes in document state and responding to errors or version conflicts
Moving documents to iCloud storage (and removing them from iCloud storage)
Deletion of the document
Relevant Chapter: Managing the Life Cycle of a Document
An Application Stores Document Files in iCloud Upon User Request
Applications give their users the option for putting all document files in iCloud storage or all document files in the local sandbox. To move document files to iCloud, they compose a file URL locating the document in an iCloud container directory of the application and then call a specific method of the NSFileManager
class, passing in the file URL. Moving document files from iCloud storage to the application sandbox follows a similar procedure.
Relevant Chapter: Managing the Life Cycle of a Document
An Application Ensures That Document Data is Saved Automatically
UIDocument
follows the saveless model and automatically saves a document’s data at specific intervals. A user usually never has to save a document explicitly. However, your application must play its part in order for the saveless model to work, either by implementing undo and redo or by tracking changes to the document.
Relevant Chapter: Change Tracking and Undo Operations
An Application Resolves Conflicts Between Different Document Versions
When documents are stored in iCloud, conflicts between versions of a document can occur. When a conflict occurs, UIKit informs the application about it. The application must attempt to resolve the conflict itself or invite the user to pick the version he or she prefers.
Relevant Chapter: Resolving Document Version Conflicts
How to Use This Document
Before you start writing any code for your document-based application, you should at least read the first two chapters, Designing a Document-Based Application and Document-Based Application Preflight. These chapters talk about design and configuration issues, and give you an overview of the tasks required for well-designed document-based applications
Prerequisites
Before you read Document-Based Application Programming Guide for iOS you should become familiar with the information presented in App Programming Guide for iOS.
See Also
The following documents are related in some way to Document-Based Application Programming Guide for iOS:
Uniform Type Identifiers Overview and the related reference discuss Uniform Type Identifiers (UTIs), which are the primary identifiers of document types.
File Metadata Search Programming Guide describes how to conduct searches using the
NSMetadataQuery
class and related classes. You use metadata queries to locate an application’s documents stored in iCloud.iCloud Design Guide provides an introduction to iCloud document support.
Copyright © 2012 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2012-09-19