Core Bluetooth Overview
The Core Bluetooth framework lets your iOS and Mac apps communicate with Bluetooth low energy devices. For example, your app can discover, explore, and interact with low energy peripheral devices, such as heart rate monitors, digital thermostats, and even other iOS devices.
The framework is an abstraction of the Bluetooth 4.0 specification for use with low energy devices. That said, it hides many of the low-level details of the specification from you, the developer, making it much easier for you to develop apps that interact with Bluetooth low energy devices. Because the framework is based on the specification, some concepts and terminology from the specification have been adopted. This chapter introduces you to the key terms and concepts that you need to know to begin developing great apps using the Core Bluetooth framework.
Central and Peripheral Devices and Their Roles in Bluetooth Communication
There are two major players involved in all Bluetooth low energy communication: the central and the peripheral. Based on a somewhat traditional client-server architecture, a peripheral typically has data that is needed by other devices. A central typically uses the information served up by peripherals to accomplish some particular task. As Figure 1-1 shows, for example, a heart rate monitor may have useful information that your Mac or iOS app may need in order to display the user’s heart rate in a user-friendly way.
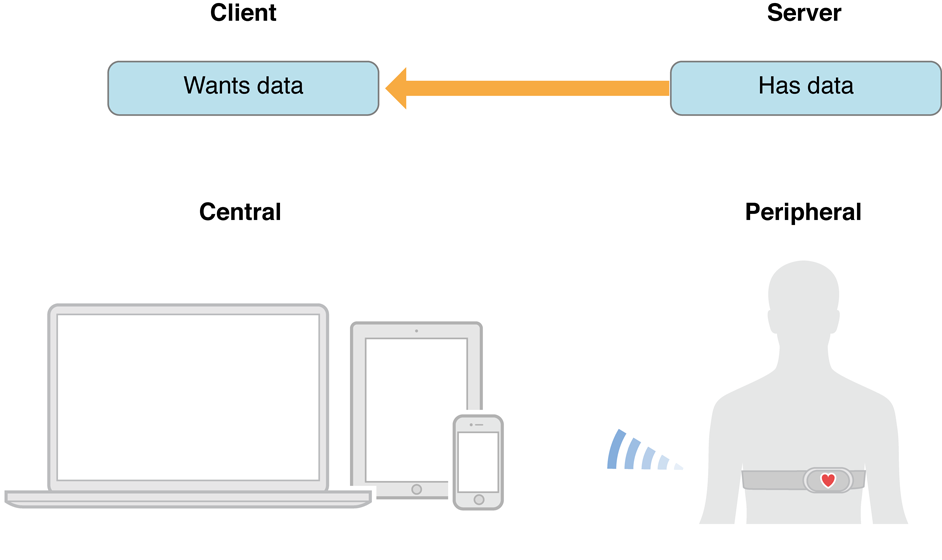
Centrals Discover and Connect to Peripherals That Are Advertising
Peripherals broadcast some of the data they have in the form of advertising packets. An advertising packet is a relatively small bundle of data that may contain useful information about what a peripheral has to offer, such as the peripheral’s name and primary functionality. For instance, a digital thermostat may advertise that it provides the current temperature of a room. In Bluetooth low energy, advertising is the primary way that peripherals make their presence known.
A central, on the other hand, can scan and listen for any peripheral device that is advertising information that it’s interested in, as shown in Figure 1-2. A central can ask to connect to any peripheral that it has discovered advertising.

How the Data of a Peripheral Is Structured
The purpose of connecting to a peripheral is to begin exploring and interacting with the data it has to offer. Before you can do this, however, it helps to understand how the data of a peripheral is structured.
Peripherals may contain one or more services or provide useful information about their connected signal strength. A service is a collection of data and associated behaviors for accomplishing a function or feature of a device (or portions of that device). For example, one service of a heart rate monitor may be to expose heart rate data from the monitor’s heart rate sensor.
Services themselves are made up of either characteristics or included services (that is, references to other services). A characteristic provides further details about a peripheral’s service. For example, the heart rate service just described may contain one characteristic that describes the intended body location of the device’s heart rate sensor and another characteristic that transmits heart rate measurement data. Figure 1-3 illustrates one possible structure of a heart rate monitor’s service and characteristics.
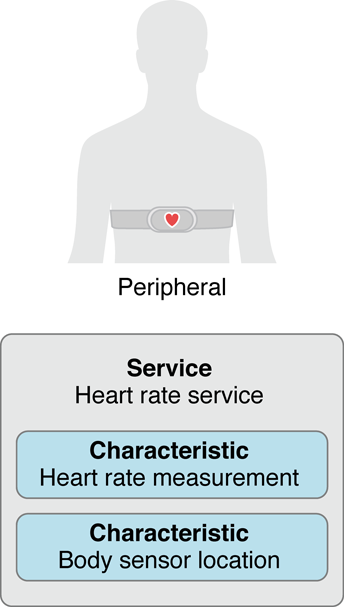
Centrals Explore and Interact with the Data on a Peripheral
After a central has successfully established a connection to a peripheral, it can discover the full range of services and characteristics the peripheral has to offer (advertising data might contain only a fraction of the available services).
A central can also interact with a peripheral’s service by reading or writing the value of that service’s characteristic. For example, your app may request the current room temperature from a digital thermostat, or it may provide the thermostat with a value at which to set the room’s temperature.
How Centrals, Peripherals, and Peripheral Data Are Represented
The major players and data involved in Bluetooth low energy communication are mapped onto the Core Bluetooth framework in a simple, straightforward way.
Objects on the Central Side
When you are using a local central to interact with a remote peripheral, you are performing actions on the central side of Bluetooth low energy communication. Unless you are setting up a local peripheral device—and using it to respond to requests by a central—most of your Bluetooth transactions will take place on the central side.
For information about how to implement the central role in your app, see Performing Common Central Role Tasks and Best Practices for Interacting with a Remote Peripheral Device
Local Centrals and Remote Peripherals
On the central side, a local central device is represented by a CBCentralManager
object. These objects are used to manage discovered or connected remote peripheral devices (represented by CBPeripheral
objects), including scanning for, discovering, and connecting to advertising peripherals. Figure 1-4 shows how local centrals and remote peripherals are represented in the Core Bluetooth framework.
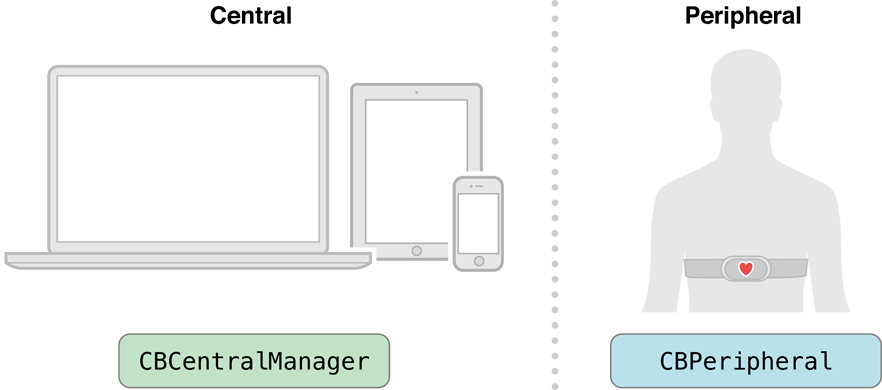
A Remote Peripheral’s Data Are Represented by CBService and CBCharacteristic Objects
When you are interacting with the data on a remote peripheral (represented by a CBPeripheral
object), you are dealing with its services and characteristics. In the Core Bluetooth framework, the services of a remote peripheral are represented by CBService
objects. Similarly, the characteristics of a remote peripheral’s service are represented by CBCharacteristic
objects. Figure 1-5 illustrates the basic structure of a remote peripheral’s services and characteristics.
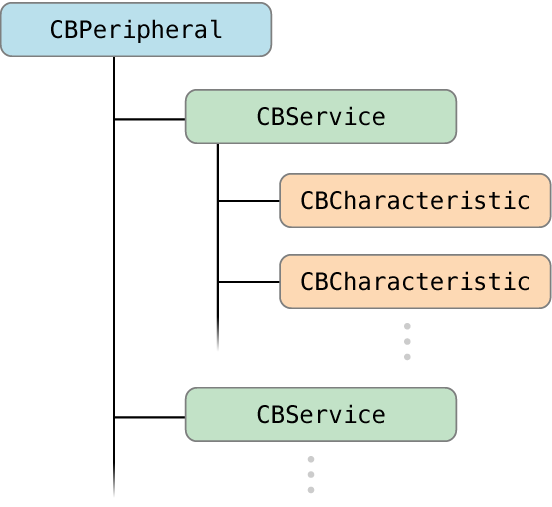
Objects on the Peripheral Side
As of macOS 10.9 and iOS 6, Mac and iOS devices can function as Bluetooth low energy peripherals, serving data to other devices, including other Mac, iPhone, and iPad devices. When setting up your device to implement the peripheral role, you are performing actions on the peripheral side of Bluetooth low energy communication.
Local Peripherals and Remote Centrals
On the peripheral side, a local peripheral device is represented by a CBPeripheralManager
object. These objects are used to manage published services within the local peripheral device’s database of services and characteristics and to advertise these services to remote central devices (represented by CBCentral
objects). Peripheral manager objects are also used to respond to read and write requests from these remote centrals. Figure 1-6 shows how local peripherals and remote centrals are represented in the Core Bluetooth framework.
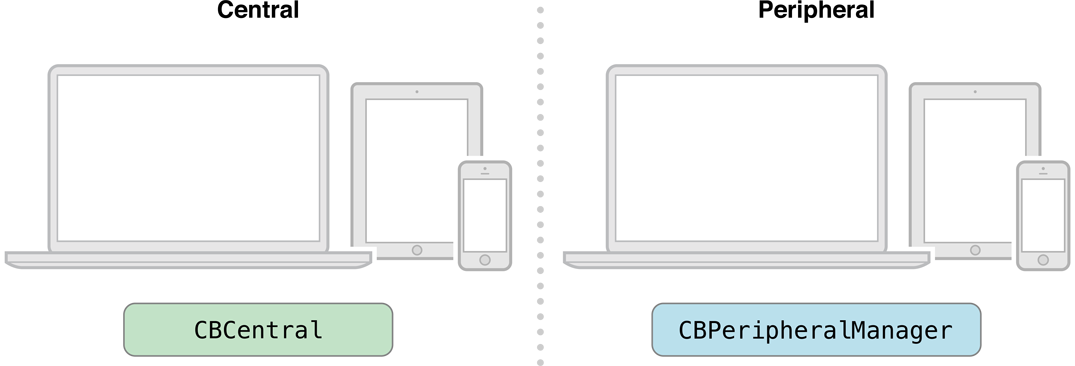
A Local Peripheral’s Data Are Represented by CBMutableService and CBMutableCharacteristic Objects
When you are setting up and interacting with the data on a local peripheral (represented by a CBPeripheralManager
object), you are dealing with mutable versions of its services and characteristics. In the Core Bluetooth framework, the services of a local peripheral are represented by CBMutableService
objects. Similarly, the characteristics of a local peripheral’s service are represented by CBMutableCharacteristic
objects. Figure 1-7 illustrates the basic structure of a local peripheral’s services and characteristics.
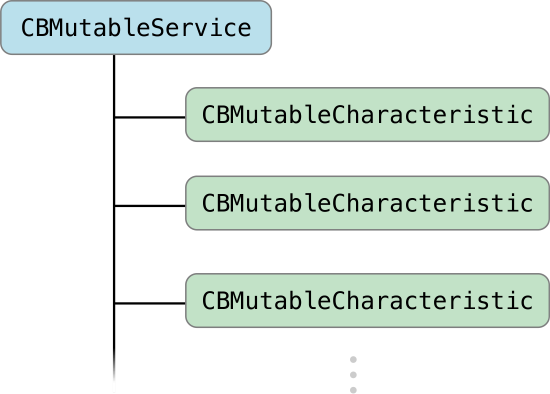
For more information about how to set up your local device to implement the peripheral role, see Performing Common Peripheral Role Tasks and Best Practices for Setting Up Your Local Device as a Peripheral.
Copyright © 2013 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2013-09-18