Safari 9.0
The following new features have been added in Safari 9.0.
Application Launch Changes
In Safari on iOS 9, subframes and timer events can not launch applications via URLs. To launch an application, a navigation action must be triggered by a user navigation gesture on the main document frame. You can provide a navigation response from the main document by setting the window.location
property, as shown below:
window.location = "com.yourdomain.applink://..." |
Subframes can navigate the main document by setting the window.top.location
property.
window.top.location = "myApp://..." |
Safari presents a confirmation prompt to open the application from custom protocol URLs.
Secure Extension Distribution
Secure Extensions Distribution introduces improved security for Safari on OS X. All extensions in the Safari Extensions Gallery are now hosted and signed by Apple. Users can trust that the Safari Extension they install is the one you submitted.
Only Safari Extensions installed from the Safari Extensions Gallery can be updated automatically. Add the following two lines to each extension's dictionary entry within your Update Manifest to allow existing users to update automatically to the latest version.
<key>Update From Gallery</key> |
<true/> |
You may still sign your Safari Extensions with your developer certificate for distribution outside of the Safari Extensions Gallery, but it will not be a candidate for automatic updating.
Force Touch Trackpad Mouse Events
Safari’s new mouse event property, webkitForce
, provides events and force information from Force Touch Trackpads. See Responding to Force Touch Events from JavaScript for an introduction to Force Touch Operations.
Use event.webkitForce
to create interactivity with the following events and constants.
webkitmouseforcewillbegin
webkitmouseforcedown
webkitmouseforceup
webkitmouseforcechanged
Constants:
MouseEvent.WEBKIT_FORCE_AT_MOUSE_DOWN
MouseEvent.WEBKIT_FORCE_AT_FORCE_MOUSE_DOWN
Viewport Changes
Viewport meta
tags using "width=device-width"
cause the page to scale down to fit content that overflows the viewport bounds. You can override this behavior by adding "shrink-to-fit=no"
to your meta
tag as shown below. The added value will prevent the page from scaling to fit the viewport.
<meta name="viewport" content="width=device-width, initial-scale=1.0, shrink-to-fit=no"> |
Content Blocking Safari Extensions
iOS
The new Safari release brings Content Blocking Safari Extensions to iOS. Content Blocking gives your extensions a fast and efficient way to block cookies, images, resources, pop-ups, and other content.
Your app extension is responsible for supplying a JSON file to Safari. The JSON consists of an array of rules (triggers and actions) for blocking specified content. Safari converts the JSON to bytecode, which it applies efficiently to all resource loads without leaking information about the user’s browsing back to the app extension.
Xcode includes a Content Blocker App Extension template that contains code to send your JSON file to Safari. Just edit the JSON file in the template to provide your own triggers and actions. The sample JSON file below contains triggers and actions that block images on webkit.org
.
Listing 1 Actions and triggers example
[ |
{ |
"action": { |
"type": "block" |
}, |
"trigger": { |
"url-filter": "webkit.org/images/icon-gold.png" |
} |
}, |
{ |
"action": { |
"selector": "a[href^=\"http://nightly.webkit.org/\"]", |
"type": "css-display-none" |
}, |
"trigger": { |
"url-filter": ".*" |
} |
} |
] |
OS X
Content Blocking is now available on OS X through Safari. Add your JSON file in the new Content Blocker section of the Safari Extensions Builder, shown in Figure 1, or use the new setContentBlocker
API available on the Safari Extension object.
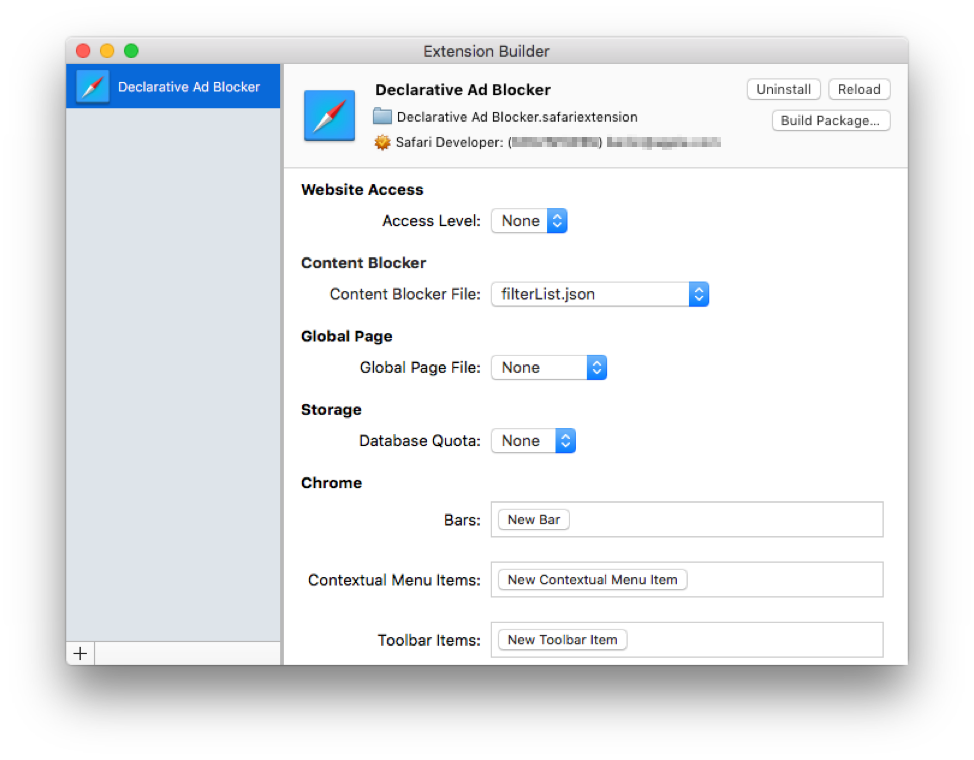
Shared Links
Safari on both iOS and OS X now allow App Extensions that send items to Safari's Shared Links sidebar. Xcode offers templates for both iOS and OS X Shared Links app extensions. The sample in Listing 2 uses the template for OS X.
Listing 2 Creating Shared Links in OS X
- (void)beginRequestWithExtensionContext:(NSExtensionContext *)context { |
NSExtensionItem *extensionItem = [[NSExtensionItem alloc] init]; |
// The keys of the user info dictionary match what data Safari expects for each Shared Links item. |
// For the date, use the publish date of the content being linked |
extensionItem.userInfo = @{ |
@"uniqueIdentifier": @"uniqueIdentifierForSampleItem", |
@"urlString": @"http://apple.com", |
@"date": [NSDate date] }; |
extensionItem.attributedTitle = [[NSAttributedString alloc] initWithString:@"Sample title"]; |
extensionItem.attributedContentText = [[NSAttributedString alloc] initWithString:@"Sample description text"]; |
// You can use the NSExtensionItem's attachments property to supply a custom image for your link. |
// extensionItem.attachments = @[ [[NSItemProvider alloc] initWithContentsOfURL:[[NSBundle mainBundle] URLForResource:@"customLinkImage" withExtension:@"png"]] ]; |
[context completeRequestReturningItems:@[ extensionItem ] completionHandler:nil]; |
} |
HTML5 Media
AirPlay
Safari 9.0 allows you to create custom controls for HTML5 media with JavaScript AirPlay support. Use Safari's WebKitPlaybackTargetAvailabilityEvent
to detect Airplay availability and then add your own controls for streaming audio and video to AirPlay devices. For more on custom controls, see AirPlay API
.
The JavaScript sample below shows an implementation of custom player controls for HTML5 sites.
Listing 3 Custom controls for Airplay
if (window.WebKitPlaybackTargetAvailabilityEvent) { |
video.addEventListener('webkitplaybacktargetavailabilitychanged', |
function(event) { |
switch (event.availability) { |
case "available": /* turn stuff on */ |
break; |
case "not-available": /* turn AirPlay button off */ |
break; |
} |
}) |
} |
Theme-specific Wireless Playback Icon
Safari 9.0 lets you use CSS to match the wireless playback icon with the theme of your custom HTML5 video controls.
.normal { |
background-image: -webkit-named-image(wireless-playback); |
background-size: 100% 100%; |
background-repeat: no-repeat; |
} |
.active { |
-webkit-mask-image: -webkit-named-image(wireless-playback); |
-webkit-mask-size: 100% 100%; |
background-color: dodgerblue; |
} |
Picture in Picture Support
Picture in Picture (PiP) maintains user engagement, allowing your video to remain in view in a floating video overlay while users interact with other apps. With Safari 9.0, when using the built-in video controls for HTML5 videos, picture-in-picture controls are made available automatically depending on device support.
You can use the JavaScript presentation mode API to add customized picture-in-picture controls for HTML5 videos. The presentation mode API for JavaScript allows switching the video playback mode from inline, to fullscreen or the new picture-in-picture mode.
Listing 4 Activating PiP when clicked
if (video.webkitSupportsPresentationMode && typeof video.webkitSetPresentationMode === "function") { |
// Toggle PiP when the user clicks the button. |
pipButtonElement.addEventListener("click", function(event) { |
video.webkitSetPresentationMode(video.webkitPresentationMode === "picture-in-picture" ? "inline" : "picture-in-picture"); |
}); |
} else { |
pipButtonElement.disabled = true; |
} |
For more information on PiP, see Picture in Picture Quick Start in Adopting Multitasking on iPad.
SFSafariViewController for iOS
You can use SFSafariViewController
to display web content within your app. The Safari View Controller shares cookies and other website data with Safari, and has many of Safari's features, like Safari AutoFill and Safari Reader. Unlike Safari itself, the Safari View Controller UI is tailored for displaying a single page, featuring a Done button that'll take users right back where they were in your app.
Consider replacing your WKWebView
or UIWebView
-based browsers with SFSafariViewController
if your app displays web content but does not customize that content.
Icons for Pinned Tabs
Pinned sites allow your users to keep their favorite websites open, running, and easily accessible.
You can set the pinned tab icon for your site by providing a vector image. Use an SVG image with a transparent background and 100% black for all vectors. Add the following markup to all your pinnable webpages, replacing "website_icon"
with your own file's name:
<link rel="mask-icon" href="website_icon.svg" color="red"> |
In the example, the color
attribute sets the display color of the image. That attribute can specify a single color with a hexadecimal value (#990000
), an RGB value (rgb(153, 0, 0)
), or a recognized color-keyword, such as: red
, lime
, or navy
.
CSS Scroll Snapping
Safari’s WebKit now provides CSS scroll snapping to keep the focal point of your content in view when scrolling momentum stops. The following properties are available to customize your page.
-webkit-scroll-snap-type:
mandatory;The viewport comes to rest on a snap point at the end of a scroll operation.
-webkit-scroll-snap-points-y:
-webkit-scroll-snap-points-x:
elements; snap to the element
repeat( Nunits ); every N-units (pixels, view height/width, percentage, calculated value)
-webkit-scroll-snap-destination:
50%
50%
;defaults to
0
(left or top depending on scroll orientation)use
50%
50%
for center coordinate of an element
-webkit-scroll-snap-coordinate:
0%
0%
;for example:
50%
50%
; aligns snap to center of the element
Backdrop Filters
Backdrop filters allow you to add advanced image filters to the backdrops of your elements. Achieve modern iOS and OS X material effects in your web content layouts using these filters. Figure 2 shows a backdrop filter with a blur effect applied to the overlay.
Safari provides for the following functions for the backdrop-filter
property:
blur()
brightness()
contrast()
drop-shadow()
grayscale()
hue-rotate()
invert()
opacity()
saturate()
sepia()
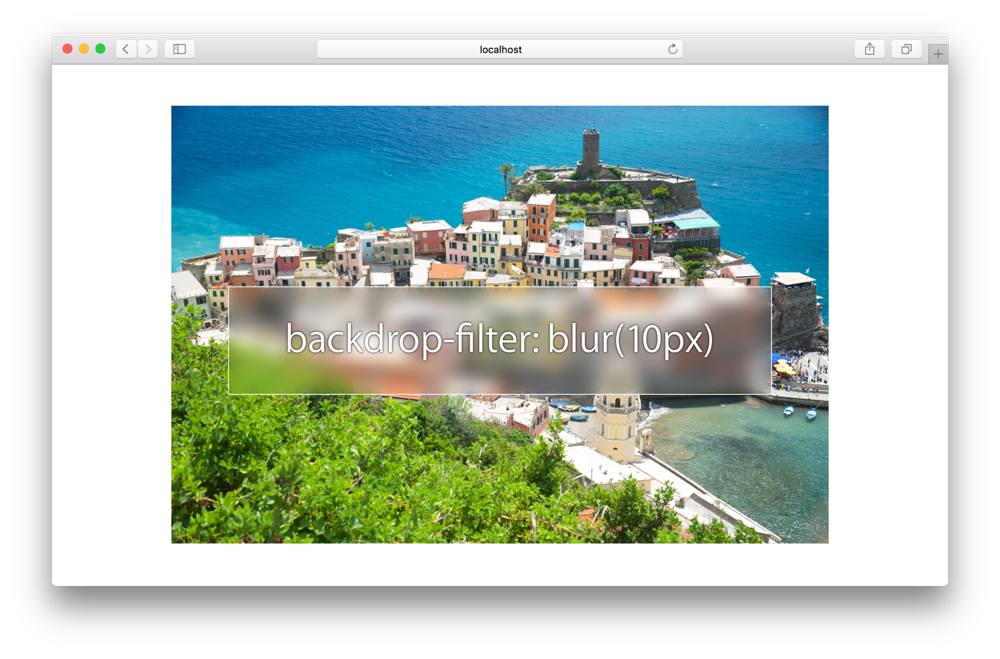
W3C offers more information on filter effects at http://dev.w3.org/fxtf/filters/#typedef-filter-function-list.
Responsive Design Mode
The Responsive Design Mode provides a fast-switching layout preview of your webpage across a spectrum of viewport sizes. This viewport quick-look tool helps you quickly spot layout issues on a webpage across many screen sizes. It includes several preset sizes for Apple devices, shown in Figure 3, and allows custom viewport sizes to be defined.
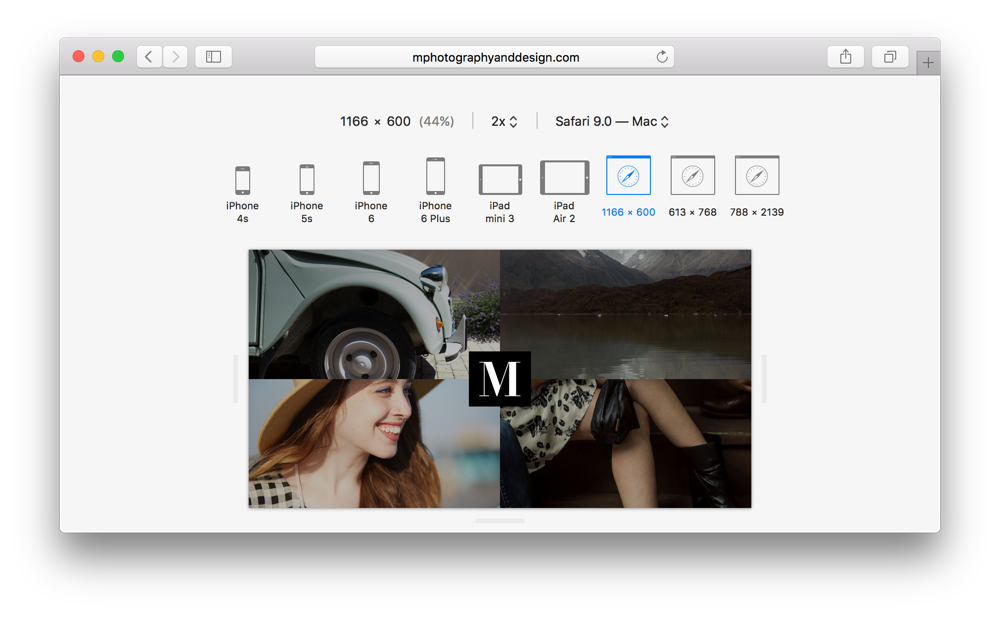
Web Inspector Redesign
The new Web Inspector design provides a more intuitive user experience. The use of tabbed view panels keeps the user oriented and provides quick navigate between development tasks.
A new frame rendering timeline, shown in Figure 4, makes it easy to see performance with respect to the frames painted to the page.
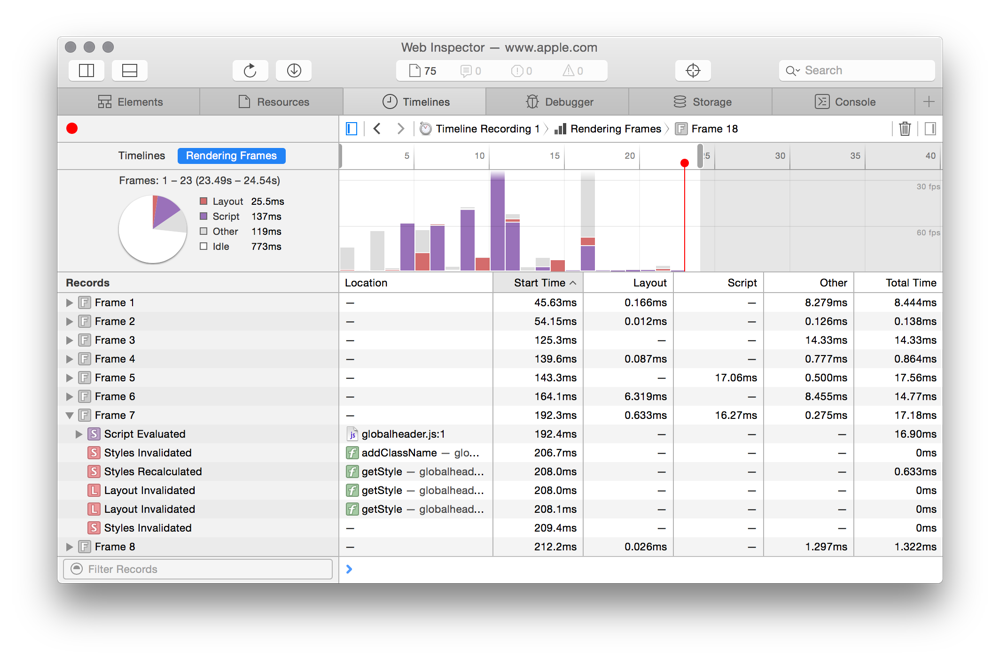
ECMAScript 6 Enhancements
The following ECMAScript 6 content is now supported by Safari:
Classes
Computed Properties
Weak Set
Number Object
Octal and Binary Literals
Symbol Objects
Template Literals
System Font Support
Apple operating systems automatically switch between two optical, size-specific font families to accommodate the new system font, "San Francisco". You can use the following CSS rule to intelligently switch web fonts to match the operating system’s typeface.
font-family: -apple-system, sans-serif;
In addition to the font, iOS lets you specify the size and weight of the system text styles. Use the CSS font property with the newly supported text-style keywords below.
font: -apple-system-body;
font: -apple-system-headline;
font: -apple-system-subheadline;
font: -apple-system-caption1;
font: -apple-system-caption2;
font: -apple-system-footnote;
font: -apple-system-short-body;
font: -apple-system-short-headline;
font: -apple-system-short-subheadline;
font: -apple-system-short-caption1;
font: -apple-system-short-footnote;
font: -apple-system-tall-body;
Initial Letter Support
You can create a drop cap—including dropped, raised, or sunken initial letter effects—using Safari 9.0's support of the initial-letter
CSS property:
initial-letter: 3 3;
The first number defines number of text lines the letter will span. The second number defines the number of lines the letter should sink.
For more on the initial-letter
property, see http://dev.w3.org/csswg/css-inline/#initial-letter-styling.
CSS Enhancements
CSS3 Feature Queries
The CSS3 enhancement, Feature Queries, use the @supports
rule. See http://www.w3.org/TR/css3-conditional/.
CSS4 Selectors
The following CSS4 selectors are now fully supported by Safari.
Case-insensitive attribute selectors, http://www.w3.org/TR/selectors4/#attribute-case
:any-link
, http://www.w3.org/TR/selectors4/#the-any-link-pseudo:matches
, http://www.w3.org/TR/selectors4/#matches:nth-child
, http://www.w3.org/TR/selectors4/#the-nth-child-pseudo:placeholder-shown
, http://www.w3.org/TR/selectors4/#placeholder
Unprefixed CSS Properties
The following properties are now fully supported by Safari, without the -webkit-
prefix.
transition
transition-delay
transition-duration
transition-property
transition-timing-function
animation
cursor: zoom-in
cursor: zoom-out
perspective
perspective-origin
transform
transform-origin
transform-style
@keyframes
animation-name
animation-duration
animation-timing-function
animation-iteration-count
animation-direction
animation-play-state
animation-delay
animation-fill-mode
order
align-content
align-items
align-self
justify-content
flex
flex-basis
flex-direction
flex-flow
flex-grow
flex-shrink
flex-wrap
break-after
break-before
break-inside
columns
column-count
column-fill
column-gap
column-rule
column-rule-color
column-rule-style
column-rule-width
column-span
column-width
alt
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-02-22