Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Notification
A notification is a message sent to one or more observing objects to inform them of an event in a program. The notification mechanism of Cocoa follows a broadcast model. It is a way for an object that initiates or handles a program event to communicate with any number of objects that want to know about that event. These recipients of the notification, known as observers, can adjust their own appearance, behavior, and state in response to the event. The object sending (or posting) the notification doesn’t have to know what those observers are. Notification is thus a powerful mechanism for attaining coordination and cohesion in a program. It reduces the need for strong dependencies between objects in a program (such dependencies would reduce the reusability of those objects). Many classes of the Foundation, AppKit, and other Objective-C frameworks define notifications that your program can register to observe.
The centerpiece of the notification mechanism is a per-process singleton object known as the notification center (NSNotificationCenter
). When an object posts a notification, it goes to the notification center, which acts as a kind of clearing house and broadcast center for notifications. Objects that need to know about an event elsewhere in the application register with the notification center to let it know they want to be notified when that event happens. Although the notification center delivers a notification to its observers synchronously, you can post notifications asynchronously using a notification queue (NSNotificationQueue
).
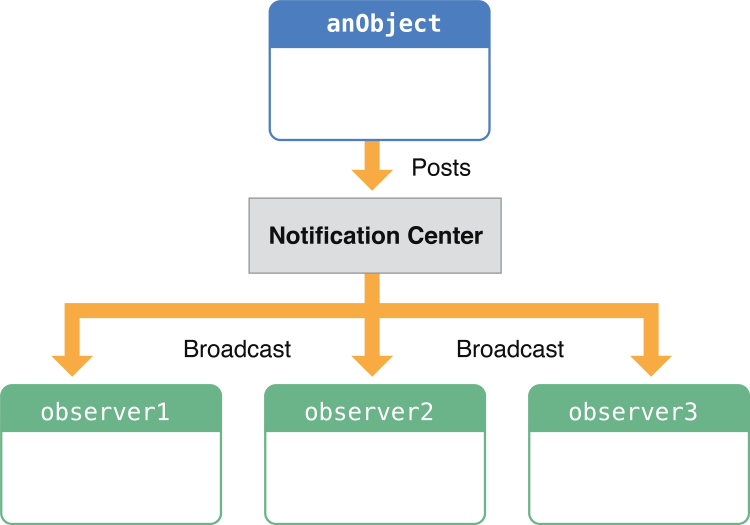
The Notification Object
A notification is represented by an instance of the NSNotification
class. A notification object contains several bits of state: a unique name, the posting object, and (optionally) a dictionary of supplementary information, called the userInfo
dictionary. When a notification is delivered to an interested observer, the notification object is passed in as an argument of the method handling the notification.
Observing a Notification
To observe a notification, obtain the singleton NSNotificationCenter
instance and send it an addObserver:selector:name:object:
message. Typically, this registration step is done shortly after your application launches. The second parameter of the addObserver:selector:name:object:
method is a selector that identifies the method that you implement to handle the notification. The method must have the following signature:
- (void)myNotificationHandler:(NSNotification *)notif; |
In this handling method, you can extract information from the notification to help you in your response, especially data in the userInfo
dictionary (if one exists).
Posting a Notification
Before posting a notification, you should define a unique global string constant as the name of your notification. A convention is to use a two- or three-letter application-specific prefix for the name, for example:
NSString *AMMyNotification = @"AMMyNotication"; |
To post the notification, send a postNotificationName:object:userInfo:
(or similar) message to the singleton NSNotificationCenter
object. This method creates the notification object before it sends the notification to the notification center.
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-04-06