Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Object comparison
Object comparison refers to the ability of an object to determine whether it is essentially the same as another object. You evaluate whether one object is equal to another by sending one of the objects an isEqual:
message and passing in the other object. If the objects are equal, you receive back YES
; if they are not equal, you receive NO
. Each class determines equality for its instances by implementing class-specific comparison logic. The root class, NSObject
, measures equality by simple pointer comparison; at the other extreme, the determinants of equality for a custom class might be class membership plus all encapsulated values.
Some classes of the Foundation framework implement comparison methods of the form isEqualTo
Type:
—for example, isEqualToString:
and isEqualToArray:
. These methods perform comparisons specific to the given class type.
The comparison methods are indispensable coding tools that can help you decide at runtime what to do with an object. The collection classes such as NSArray
and NSDictionary
use them extensively.
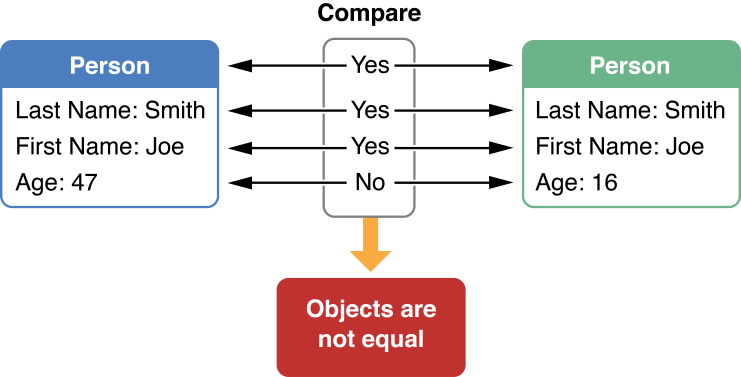
Implementing Comparison Logic
If you expect instances of your custom subclass to be compared, override the isEqual:
method and add comparison logic that is specific to your subclass. Your class, for example, might accept the superclass’s determination of equality but then add further tests. Your class may have one or more instance variables whose values should be equal before two instances of your class can be considered equal. The following implementation of isEqual:
performs a series of checks, ending with one that is class-specific (the name
property).
- (BOOL)isEqual:(id)other { |
if (other == self) |
return YES; |
if (![super isEqual:other]) |
return NO; |
return [[self name] isEqualToString:[other name]]; // class-specific |
} |
If you override isEqual:
, you should also implement the hash
method to generate and return an integer that can be used as a table address in a hash table structure. If isEqual:
determines that two objects are equal, they must have the same hash value.
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-04-06