Page View Controllers
You use a page view controller to present content in a page-by-page manner. A page view controller manages a self-contained view hierarchy. The parent view of this hierarchy is managed by the page view controller, and the child views are managed by the content view controllers that you provide.
Anatomy of a Page View Controller Interface
A page view controller has a single view in which it hosts your content. The UI provided by a page view controller is visible when the user is turning the page. The page view controller applies a page curl to its view controler’s view, providing the visual appearance of a page being turned.
The navigation provided by a page view controller is a linear series of pages. It is well suited for presenting content that is accessed in a linear fashion, such as the text of a novel, and for presenting content that has natural page breaks, such as a calendar. For content that the user needs to access in a nonlinear fashion, such as a reference book, you are responsible for providing the navigation logic and UI.
Figure 3-1 shows the page view interface implemented by a sample application. The outermost brown view is associated with the parent view controller, not the page view controller itself. The page view controller has no UI of its own; however, it does add a page curl effect to its children while the user is turning the page. The custom content is provided by the view controllers that are children of the page view controller.

The Objects of a Page View Controller Interface
A page view interface consists of the following objects:
An optional delegate
An optional data source
An array of the current view controllers
An array of gesture recognizers
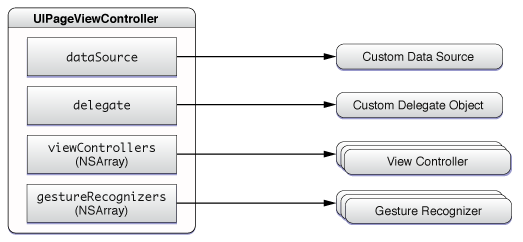
The data source provides view controllers on demand.
The delegate provides methods that are called in response to gesture-based navigation and orientation changes.
The array of view controllers contains the content view controllers that are currently being displayed. The number of items in this array varies depending on the options passed to the page view controller.
The array of gesture recognizers is populated only if a data source is set. These gesture recognizers let the user turn pages by tapping, flicking, or dragging.
Creating a Page View Controller Interface
A page view controller’s view can be resized and embedded in a view hierarchy. This means that, unlike a navigation controller or tab bar controller, a page view controller can be used in a wide variety of settings, not just a few specific cases.
Creating a Page View Controller Interface Using a Storyboard
The Page-Based Application Xcode template creates a new project with a page view controller as the initial scene.
To add a page view controller to an existing storyboard, do the following:
Drag a page view controller out of the library. Add a page view controller scene to your storyboard.
In the Attributes inspector, set up the appropriate options.
Optionally, set a delegate, a data source, or both by connecting the corresponding outlets.
Display it as the first view controller by selecting the option Is Initial View Controller in the Attributes inspector (or present the view controller in your user interface in another way.)
Creating a Page View Controller Interface Programmatically
To create a page view controller programmatically:
Allocate and initialize a page view controller using the
initWithTransitionStyle:navigationOrientation:options:
method. For initialization-time customization, see Customizing Behavior at Initialization.Optionally, set a delegate, a data source, or both.
Set the initial content view controllers.
Present the page view controller’s view on the screen.
Setting an Initial View Controller
Whether you create a page view controller in Interface Builder or programmatically, you need to set its initial view controllers before displaying it on the screen.
To set the initial view controller, call the setViewControllers:direction:animated:completion:
method with an array containing the appropriate number of view controllers.
Customizing Behavior at Initialization
You pass parameter values and options to the initWithTransitionStyle:navigationOrientation:options:
method to customize a page view controller when initializing it. They are accessible as read-only properties after initialization. You can customize:
The direction that navigation occurs: horizontally or vertically
The location of the spine: at either edge or in the center
The transition style: a page curl or scrolling
For example, Listing 3-1 initializes a page view controller with horizontal navigation, the spine in the center, and a page curl transition:
Listing 3-1 Customizing a page view controller
NSDictionary * options = [NSDictionary dictionaryWithObject: |
[NSNumber numberWithInt:UIPageViewControllerSpineLocationMid] |
forKey:UIPageViewControllerOptionSpineLocationKey]; |
UIPageViewController *pageViewController = [[UIPageViewController alloc] |
initWithTransitionStyle:UIPageViewControllerTransitionStylePageCurl |
navigationOrientation:UIPageViewControllerNavigationOrientationHorizontal |
options:options]; |
Customizing Behavior at Run Time with a Delegate
The page view controller’s delegate implements the UIPageViewControllerDelegate
protocol. It can perform actions when the device orientation changes and when the user navigates to a new page, and it can update the spine location in response to a change in the interface orientation.
Supplying Content by Providing a Data Source
Supplying a data source enables gesture-driven navigation. Without a data source, you must provide your own UI for navigation and supply content as described in Supplying Content by Setting the Current View Controller. The data source you provide must implement the UIPageViewControllerDataSource
protocol.
The methods of the data source are called with the currently displayed view controller, and return the view controller that come before or after them. To simplify the process of finding the previous or next view controller, you can store additional information in your view controllers, such as a page number.
If a data source is supplied, the page view controller associates gesture recognizers to its view. These gesture recognizers let the user turn the pages by tapping, flicking, and dragging; they are accessible as the gestureRecognizers
property.
To move the gesture recognizers to another view, pass the value of the gestureRecognizers
property to the addGestureRecognizer:
method of the target view. Moving the gesture recognizers to another view is particularly useful if you embed the page view controller into a larger view hierarchy.
For example, if the page view controller doesn’t fill the entire bounds of the screen, placing the gesture recognizers on a larger superview can make it easier for users to initiate page turns. Moving the gesture recognizers lets the users start their gestures anywhere within the superview’s bounds, not just within the bounds of the page view controller’s view.
Supplying Content by Setting the Current View Controller
To directly control what content is being displayed, call the setViewControllers:direction:animated:completion:
method, passing an array of content view controllers to display. The number of view controllers to pass varies; see the method’s reference documentation for specific details.
This approach is how you would let the user jump to a specific location in the content, such as the first page or an overview: You set the view controllers directly, in response to the user interacting with the UI.
If you do not provide a data source, you need to provide UI for moving between pages, such as forward and backward buttons. Gesture-driven navigation is available only when a you provide a datasource.
Special Consideration for Right-to-Left and Bottom-to-Top Content
The page view controller understands content to the left and to the top as being before the current page, and it understands content to the right and to the bottom as being after the current page. This matches the usage for left-to-right and top-to-bottom content.
To use a page view controller to display right-to-left or bottom-to-top content from a data source, just reverse the behavior of two methods:
In your data source, implement
pageViewController:viewControllerBeforeViewController:
to return the view controller after the given view controller.In your data source, implement
pageViewController:viewControllerAfterViewController:
to return the view controller before the given view controller.
For right-to-left or top-to-bottom content, you typically want to set the spine location to UIPageViewControllerSpineLocationMax
.
Copyright © 2014 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2014-11-15