Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Object ownership
Objective-C uses reference counting to manage the memory of its objects through the concept of object ownership. When compiling code with the ARC feature (enabled by default), the compiler takes the references you create and automatically inserts calls to the underlying memory management mechanism. A reference to an object is any object pointer or property that lets you reach the object.
There are two types of object reference:
Strong references, which keep an object “alive” in memory.
Weak references, which have no effect on the lifetime of a referenced object.
Most references in your code are strong references—this is the default behavior when you declare an object reference using the form:
NSString *someReferenceToAString; |
A strong reference ensures that the referenced object remains in memory (that is, it does not get deallocated) for as long as the reference is valid. When there are no remaining strong references to an object, the object gets deallocated.
Strong references are not always the right choice. For example, if two objects need to refer to each other, making both references strong would create a strong reference cycle, preventing either object from ever being deallocated. In these cases, you use weak references.
A weak reference has no effect on the lifecycle of the object it refers to: when no strong references to the object remain, even if there are still weak references, the object gets deallocated, and any weak references to the object are set to nil
. Because of this nil
behavior, weak references are sometimes called zeroing weak references.
To create a weak reference, use the __weak
qualifier as follows:
NSString * __weak someWeakReferenceToAString; |
To decide whether a reference should be strong or weak, consider whether it expresses ownership, depencence, or containment. For example, a superview maintains strong references to its subviews because it has ownership of them; an app delegate maintains strong references to its data models, controllers, and views because it depends on their continued existence; an array maintains strong references to the objects it contains. However, a subview maintains a weak reference to its parent view because the reference is one of convenience: a view might not even have a parent view in some circumstances. Similarly, a view that presents data maintains weak references to its delegate and data source; the view is owned by some other object, usually a view controller, which commonly acts as the delegate and data source.
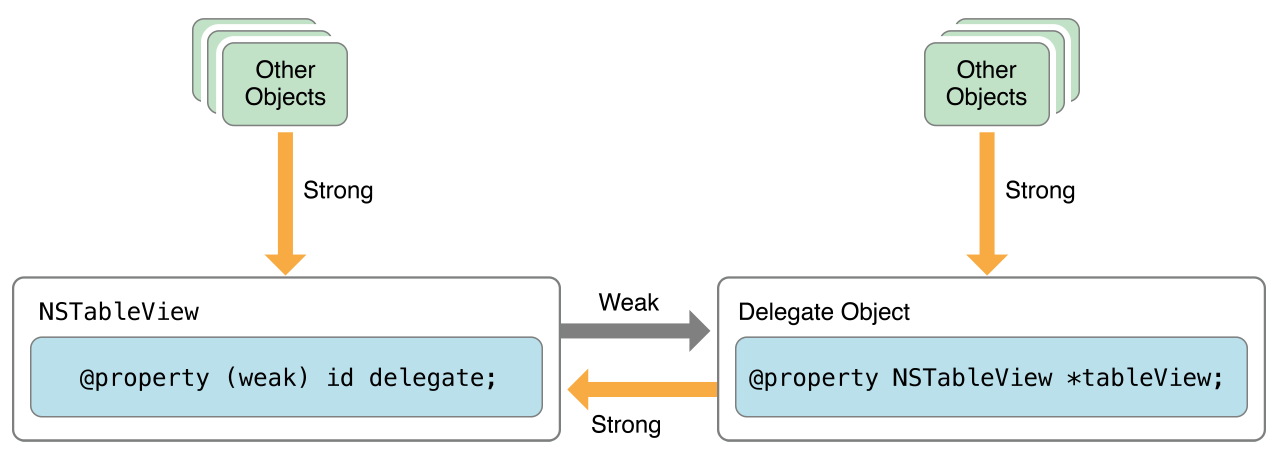
In effect, all objects in memory form a hierchy that begins with the application itself and continues down through the strong references it maintains: the application keeps a strong reference to its app delegate, which keeps strong references to the top-level MVC objects, which keep strong references to the objects they need to perform their individual tasks. When lower level objects refer to objects higher up in the memory tree, they use weak references. These lower level objects don’t own the higher level objects, and keeping a strong reference to them would create a strong reference cycle.
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-04-06