Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Memory management
Memory management is the programming discipline of managing the life cycles of objects and freeing them when they are no longer needed. Managing object memory is a matter of performance; if an application doesn’t free unneeded objects, its memory footprint grows and performance suffers. Memory management in a Cocoa application that doesn’t use garbage collection is based on a reference counting model. When you create or copy an object, its retain count is 1. Thereafter other objects may express an ownership interest in your object, which increments its retain count. The owners of an object may also relinquish their ownership interest in it, which decrements the retain count. When the retain count becomes zero, the object is deallocated (destroyed).
To assist you in memory management, Objective-C gives you methods and mechanisms that you must use in conformance with a set of rules.
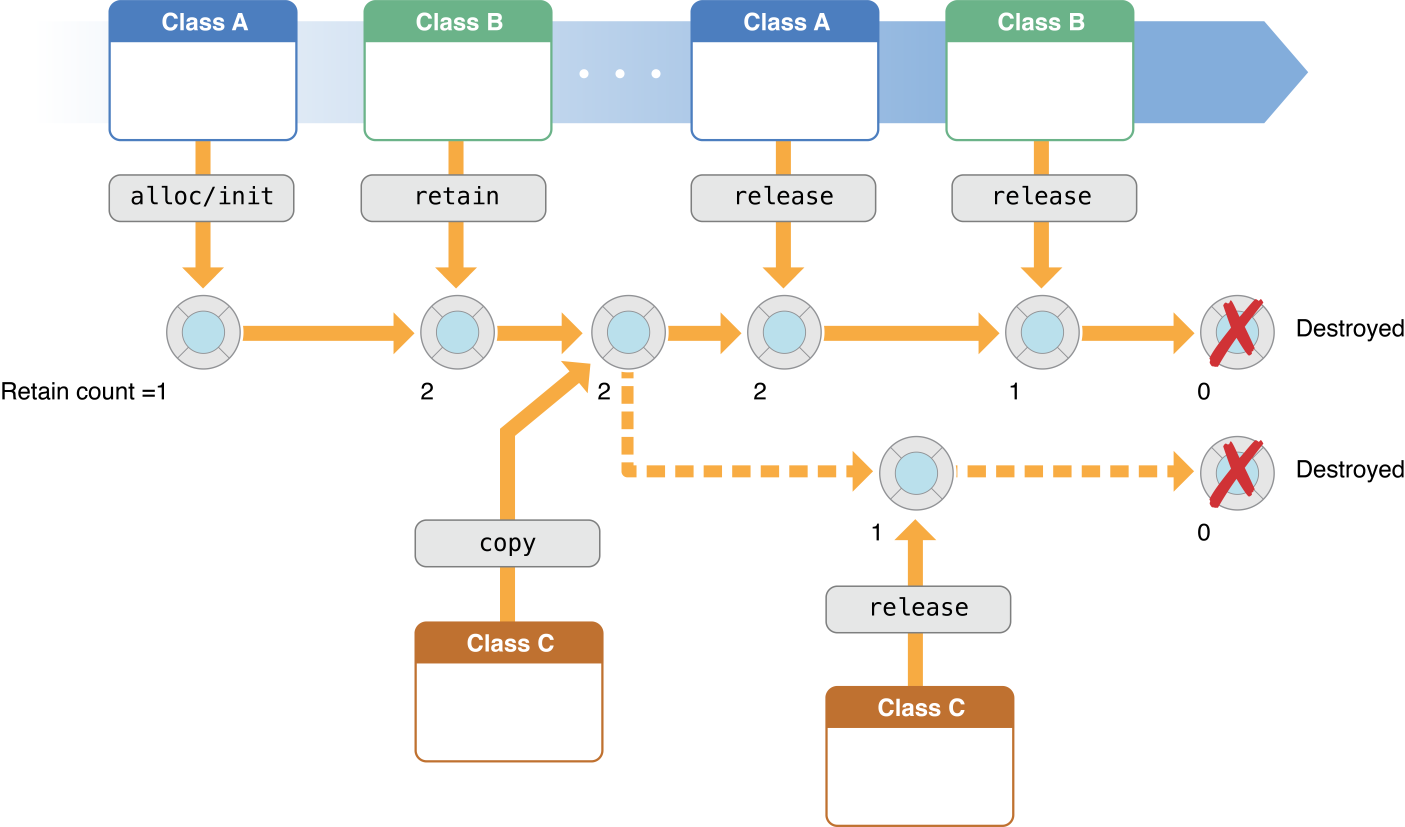
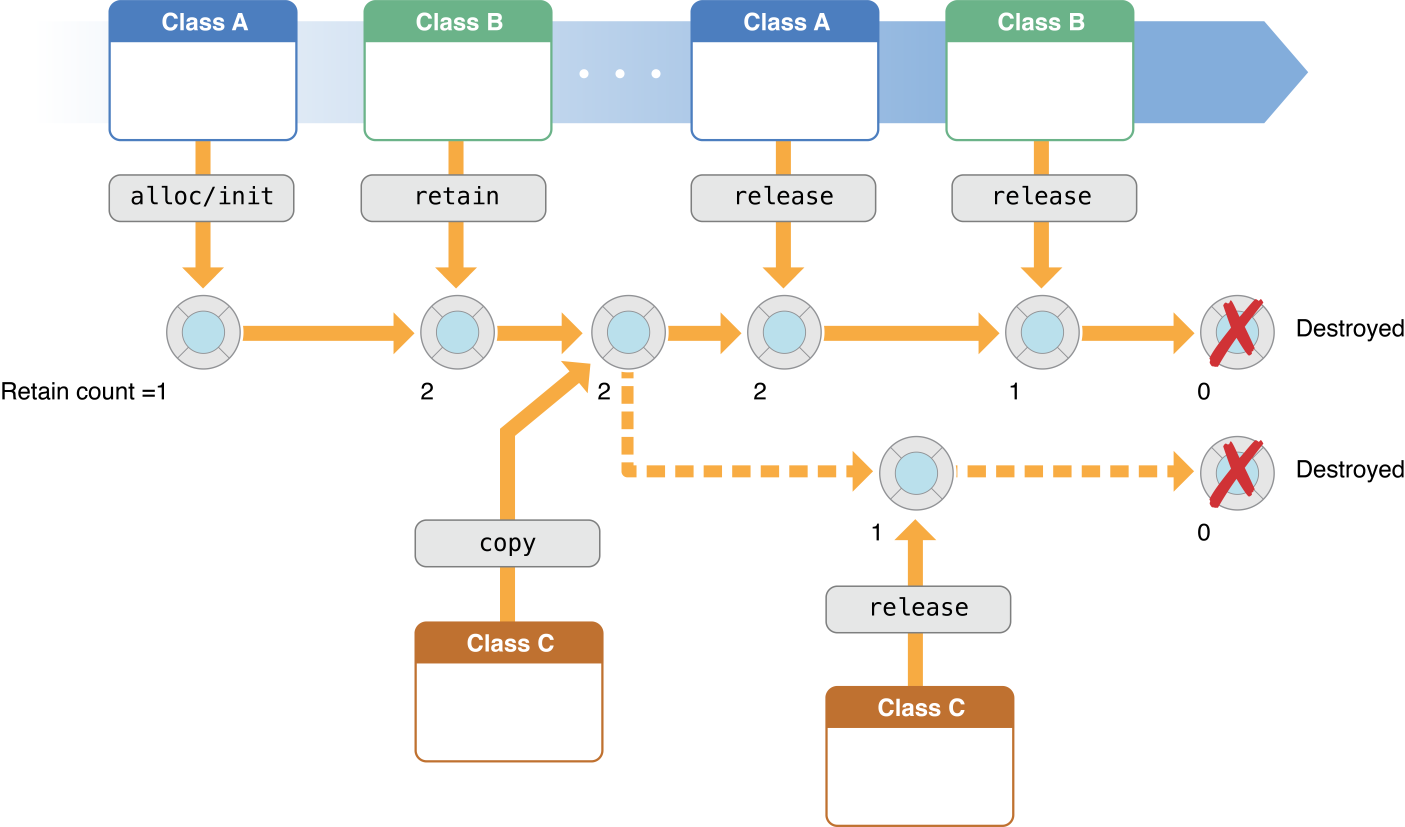
Memory-Management Rules
Memory-management rules, sometimes referred to as the ownership policy, help you to explicitly manage memory in Objective-C code.
You own any object you create by allocating memory for it or copying it.
Related methods:
alloc
,allocWithZone:
,copy
,copyWithZone:
,mutableCopy
,mutableCopyWithZone:
If you are not the creator of an object, but want to ensure it stays in memory for you to use, you can express an ownership interest in it.
Related method:
retain
If you own an object, either by creating it or expressing an ownership interest, you are responsible for releasing it when you no longer need it.
Related methods:
release
,autorelease
Conversely, if you are not the creator of an object and have not expressed an ownership interest, you must not release it.
If you receive an object from elsewhere in your program, it is normally guaranteed to remain valid within the method or function it was received in. If you want it to remain valid beyond that scope, you should retain or copy it. If you try to release an object that has already been deallocated, your program crashes.
Aspects of Memory Management
The following concepts are essential to understanding and properly managing object memory:
Autorelease pools. Sending
autorelease
to an object marks the object for later release, which is useful when you want the released object to persist beyond the current scope. Autoreleasing an object puts it in an autorelease pool (an instance ofNSAutoreleasePool
), which is created for an arbitrary program scope. When program execution exits that scope, the objects in the pool are released.Deallocation. When an object’s retain count drops to zero, the runtime calls the
dealloc
method of the object’s class just before it destroys the object. A class implements this method to free any resources the object holds, including objects pointed to by its instance variables.Factory methods. Many framework classes define class methods that, as a convenience, create objects of the class for you. These returned objects are not guaranteed to be valid beyond the receiving method’s scope.
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-04-06