Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Movie Time and Space
This chapter describes the functions of the movie toolbox that your application will use to manipulate the timebase and spatial characteristics of movies, tracks, and media.
Working With Movie Spatial Characteristics
The Movie Toolbox provides a number of functions that allow your application to determine and change the spatial characteristics of movies and tracks. Before using any of them, you should be familiar with the way in which the Movie Toolbox displays movies. Here are some tips for using these functions:
You can use the
SetMovieGWorld
andGetMovieGWorld
functions to work with a movie’s graphics world.Your application can work with a movie’s matrix by calling the
GetMovieMatrix
andSetMovieMatrix
functions, and it can work with a track’s matrix with theGetTrackMatrix
andSetTrackMatrix
functions. Then you can perform operations on matrices with the Movie Toolbox’s matrix functions described in Matrix Functions.Certain functions affect the displayed movie and its tracks in the final display coordinate system. The
SetMovieGWorld
andGetMovieGWorld
functions let you work with a movie’s display destination. TheGetMovieBox
andSetMovieBox
functions allow you to work with a movie’s boundary rectangle and its associated transformations. Alternatively, you can use theGetMovieMatrix
andSetMovieMatrix
functions to work directly with a movie’s transformation matrix. TheGetMovieDisplayBoundsRgn
function determines a movie’s boundary region at the current movie time. On the other hand, theGetMovieSegmentDisplayBoundsRgn
function determines a movie’s boundary region over a specified time segment. You can use theGetMovieDisplayClipRgn
andSetMovieDisplayClipRgn
functions to work with a movie’s display clipping region.The
GetTrackDisplayBoundsRgn
andGetTrackSegmentDisplayBoundsRgn
functions determine a track’s final boundary region. You can use theGetTrackLayer
andSetTrackLayer
functions to control the drawing order of tracks within a movie.A number of functions affect a movie’s display boundaries before any display transformations. These functions operate in the movie’s display coordinate system. You can use the
GetMovieClipRgn
andSetMovieClipRgn
functions to work with a movie’s clipping region; that is, the clipping region that is applied before the movie display transformation. Use theGetMovieBoundsRgn
function to determine a movie’s boundary region at the current movie time.Use the
GetTrackMovieBoundsRgn
function to work with a track’s boundary region after matrix transformations have placed the track into the movie’s display system. TheSetTrackMatrix
andGetTrackMatrix
functions let you define a track’s matrix transformations.The Movie Toolbox provides several functions that affect a track’s display boundaries; these functions operate in the track’s display coordinate system before any other display transformations are applied. The
GetTrackDimensions
andSetTrackDimensions
functions allow you to establish a track’s coordinate system and to establish a track’s source rectangle.You can use the
GetTrackBoundsRgn
function to determine a track’s boundary region. TheGetTrackClipRgn
andSetTrackClipRgn
functions let you work with a track’s clipping region. You can use theGetTrackMatte
andSetTrackMatte
functions to establish a track’s matte. TheDisposeMatte
function allows you to dispose of a matte once you are finished with it.
Matrix Functions
The Movie Toolbox provides a number of functions that allow you to work with transformation matrices. This section describes those functions. For descriptions of fixed-point and fixed-rectangle structures, see The Fixed-Point and Fixed-Rectangle Structures.
The Fixed-Point and Fixed-Rectangle Structures
The Movie Toolbox matrix functions provide two mechanisms for specifying points and rectangles. Some of the functions work with standard QuickDraw points and rectangles, which use integer values to identify coordinates. Others, such as the TransformFixedRect
function, work with points and rectangles whose coordinates are expressed as fixed-point numbers. By using fixed-point numbers in these points and rectangles, the Movie Toolbox can support a greater degree of precision when defining graphic objects.
The FixedPoint
data type defines a fixed point. The FixedRect
data type defines a fixed rectangle. Note that both of these structures define the x coordinate before the y coordinate. This is different from the standard QuickDraw structures.
struct FixedPoint |
{ |
Fixed x; /* point's x coordinate as fixed-point number */ |
Fixed y; /* point's y coordinate as fixed-point number */ |
}; |
typedef struct FixedPoint FixedPoint; |
Field |
Description |
---|---|
|
Defines the point's x coordinate as a fixed-point number. |
|
Defines the point's y coordinate as a fixed-point number. |
struct FixedRect |
{ |
Fixed left; /* x coordinate of upper-left corner */ |
Fixed top; /* y coordinate of upper-left corner */ |
Fixed right; /* x coordinate of lower-right corner */ |
Fixed bottom; /* y coordinate of lower-right corner */ |
}; |
typedef struct FixedRect FixedRect; |
Field |
Description |
---|---|
|
Defines the x coordinate of the upper-left corner of the rectangle as a fixed-point number. |
|
Defines the y coordinate of the upper-left corner of the rectangle as a fixed-point number. |
|
Defines the x coordinate of the lower-right corner of the rectangle as a fixed-point number. |
|
Defines the y coordinate of the lower-right corner of the rectangle as a fixed-point number. |
Creating a Track Matte
Listing 1-1 provides an example of how to create a track matte. The CreateTrackMatte
function adds an uninitialized, 8-bit-deep, grayscale matte to a track. The UpdateTrackMatte
function draws a gray ramp rectangle around the edge of the matte and fills the center of the matte with black. (A ramp rectangle shades gradually from light to dark in smooth increments.)
Listing 1-1 Creating a track matte
void CreateTrackMatte (Track theTrack) |
{ |
QDErr err; |
GWorldPtr aGW; |
Rect trackBox; |
Fixed trackHeight; |
Fixed trackWidth; |
CTabHandle grayCTab; |
GetTrackDimensions (theTrack, &trackWidth, &trackHeight); |
SetRect (&trackBox, 0, 0, FixRound (trackWidth), |
FixRound (trackHeight)); |
grayCTab = GetCTable(40); /* 8 bit + 32 = 8 bit gray */ |
err = NewGWorld (&aGW, 8, &trackBox, grayCTab, |
(GDHandle) nil, 0); |
DisposeCTable (grayCTab); |
if (!err && (aGW != nil)) |
{ |
SetTrackMatte (theTrack, aGW->portPixMap); |
DisposeGWorld (aGW); |
} |
} |
void UpdateTrackMatte (Track theTrack) |
{ |
OSErr err; |
PixMapHandle trackMatte; |
PixMapHandle savePortPix; |
Movie theMovie; |
GWorldPtr tempGW; |
CGrafPtr savePort; |
GDHandle saveGDevice; |
Rect matteBox; |
short i; |
theMovie = GetTrackMovie (theTrack); |
trackMatte = GetTrackMatte (theTrack); |
if (trackMatte == nil) |
{ |
/* track doesn't have a matte, so give it one */ |
CreateTrackMatte (theTrack); |
trackMatte = GetTrackMatte (theTrack); |
if (trackMatte == nil) |
return; |
} |
GetGWorld (&savePort, &saveGDevice); |
matteBox = (**trackMatte).bounds; |
err = NewGWorld(&tempGW, |
(**trackMatte).pixelSize, &matteBox, |
(**trackMatte).pmTable, (GDHandle) nil, 0); |
if (err || (tempGW == nil)) return; |
SetGWorld (tempGW, nil); |
savePortPix = tempGW->portPixMap; |
LockPixels (trackMatte); |
SetPortPix (trackMatte); |
/* draw a gray ramp rectangle around the edge of the matte */ |
for (i = 0; i < 35; i++) |
{ |
RGBColor aColor; |
long tempLong; |
tempLong = 65536 - ((65536 / 35) * (long)i); |
aColor.red = aColor.green = aColor.blue = tempLong; |
RGBForeColor(&aColor); |
FrameRect (&matteBox); |
InsetRect (&matteBox, 1, 1); |
} |
/* fill the center of the matte with black */ |
ForeColor (blackColor); |
PaintRect (&matteBox); |
SetPortPix (savePortPix); |
SetGWorld (savePort, saveGDevice); |
DisposeGWorld (tempGW); |
UnlockPixels (trackMatte); |
SetTrackMatte (theTrack, trackMatte); |
DisposeMatte (trackMatte); |
} |
Working With Movie Time
Every QuickTime movie has its own time base. A movie’s time base allows all the tracks that make up the movie to be synchronized when the movie is played. The Movie Toolbox provides a number of functions that allow your application to determine and establish the time parameters of a movie:
You can use the
GetMovieTimeBase
function to retrieve the time base for a movie.You can work with a movie’s current time by calling the
GetMovieTime
,SetMovieTime
, andSetMovieTimeValue
functions.You can work with a movie’s time scale by calling the
GetMovieTimeScale
andSetMovieTimeScale
functions.The Movie Toolbox can calculate the total duration of a movie. You can use the
GetMovieDuration
function to retrieve a movie’s duration.Your application can call the
GetMovieRate
andSetMovieRate
to work with a movie’s playback rate.
The next sections discuss those functions. Later sections in this chapter discuss the Movie Toolbox functions that allow you to work with the time parameters of tracks and media structures. For information about more functions that work with time, see Time Base Functions.
About Movie Time
At the most basic level, the Movie Toolbox allows you to process time based data. As such, the Movie Toolbox must provide a description of the time basis of that data as well as a definition of the context for evaluating that time basis. In QuickTime, a movie’s time basis is referred to as its time base. Geometrically, you can think of the time base as a vector that defines the direction and velocity of time for a movie. The context for a time base is called its time coordinate system. Essentially, the time coordinate system defines the axis on which the time base vector is plotted. The smallest single unit of time marked on that axis is defined by the time scale as the units per absolute second.
Time Coordinate Systems
A movie’s time coordinate system provides the context for evaluating the passage of time in the movie. If you think of the time coordinate system as defining an axis for measuring time, it is only natural that this axis would be marked with a scale that defines a basic unit of measurement. In QuickTime, that measurement system is called a time scale.
A QuickTime time scale defines the number of time units that pass each second in a given time coordinate system. A time coordinate system that has a time scale of 1 measures time in seconds. Similarly, a time coordinate system that has a time scale of 60 measures sixtieths of a second. In general, each time unit in a time coordinate system is equal to (1/time scale) seconds. Some common time scales are listed in Table 1-1.
Time scale |
Absolute time measured |
---|---|
1 |
Seconds |
60 |
Sixtieths of a second (Macintosh ticks) |
1000 |
Milliseconds |
22254.54 |
Sound sampled at 22 kHz |
Figure 1-1 shows a duration of two seconds in absolute time and equivalent durations in the common time scales listed in Table 1-1.
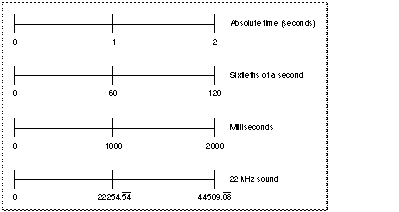
A particular point in time in a time coordinate system is represented using a time value. A time value is expressed in terms of the time scale of its time coordinate system. Without an appropriate time scale, a time value is meaningless. For example, in a time coordinate system with a time scale of 60, a time value of 180 translates to 3 seconds. Because all time coordinate systems tie back to absolute time (that is, time as we measure it in seconds), the Movie Toolbox can translate time values from one time coordinate system into another.
Time coordinate systems have a finite maximum duration that defines the maximum time value for a time coordinate system (the minimum time value is always 0). Note that as a QuickTime movie is edited, the duration changes.
As the value of the time scale increases (as the time unit for a coordinate system gets smaller in terms of absolute time), the maximum absolute time that can be represented in a time coordinate system decreases. For example, if a time value were represented as an unsigned 16-bit integer, its maximum value would be 65,535. In a time coordinate system with a time scale of 1, the maximum time value would represent 65,535 seconds. However, in a time coordinate system with a time scale of 5, the maximum time value would correspond to 13,107 seconds. Hence, a time coordinate system’s duration is limited by its time scale. QuickTime uses 32-bit and 64-bit quantities to represent time values, so you only need to worry about attaining a maximum absolute time in situations where a time coordinate system’s duration is very long or its time scale is very large.
Time Bases
A movie’s time base defines its current time value and the rate at which time passes for the movie. The rate specifies the speed and direction in which time travels in a movie. Negative rate values cause you to move backward through a movie’s data; positive values move forward. The time base also contains a reference to the clock that provides timing for the time base. QuickTime clocks are implemented as components that are managed by the Component Manager.
Time bases exist independently of any specific time coordinate system. However, time values extracted from a time base are meaningless without a time scale. Therefore, whenever you obtain a time value from a time base, you must specify the time scale of the time value result. The Movie Toolbox translates the time base’s time value into a value that is sensible in the specified time scale.
Figure 1-2 represents a time coordinate system and a time base geometrically. The time coordinate system is represented by a coordinate axis. In this example, the time coordinate system has a time scale of 2; that is, there are two time units in each second. The duration of this time coordinate system is 2 seconds, which is equivalent to 4 time units. An object’s time base is depicted by the large arrow under the axis that represents the time coordinate system. This time base has a current time value of 3 and a rate of 1. The starting time is a time value, expressed in the units of the time coordinate system.
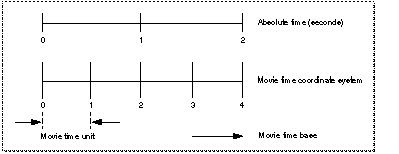
Working With Track Time
The Movie Toolbox provides several functions that allow your application to determine and establish a track’s time parameters. A track uses the time base of the movie that contains the track; therefore there are no functions that work with a track’s time base or time scale. However, you can determine a track’s duration and its offset from the start of a movie.
All of the tracks in a movie use the movie’s time coordinate system. That is, the movie’s time scale defines the basic time unit for each of the movie’s tracks. Each track begins at the beginning of the movie, but the track’s data might not begin until some time value other than 0. This intervening time is represented by blank space. In an audio track the blank space translates to silence; in a video track the blank space generates no visual image. This blank space is the track offset. Each track has its own duration. This duration need not correspond to the duration of the movie. A movie duration always equals the maximum track duration. These functions help you work with track time:
You can use the
GetTrackDuration
function to determine a track’s duration.The
SetTrackOffset
andGetTrackOffset
functions enable you to work with a track’s offset from the start of the movie that contains it.The
TrackTimeToMediaTime
function lets you translate a track’s time to the corresponding time value of a media in the track.
Working With Media Time
The Movie Toolbox provides functions that allow your application to work with the time parameters of a media:
You can use the
GetMediaDuration
function to determine a media’s duration.The
GetMediaTimeScale
andSetMediaTimeScale
let you determine or establish a media’s time scale.
Time Base Functions
The Movie Toolbox provides a number of functions that allow you to work with time bases. A QuickTime time base defines the time coordinate system of a movie. However, you can also use QuickTime time bases to provide general timing services. This section describes the functions that allow your application to work with time bases.
This section has been divided into the following topics:
Creating and Disposing of Time Bases describes how to create and dispose of time bases and how to assign a time base to a movie
Working With Time Base Values discusses functions that allow your application to work with the contents of a time base
Time Structures describes a number of functions that allow you to convert times between time bases and to perform simple arithmetic on time values
Time Base Callback Functions describes the functions your application may use to condition a time base to invoke functions your application provides
Creating and Disposing of Time Bases
The following Movie Toolbox functions help your application create and dispose of time bases:
The
NewTimeBase
function lets you create a new time base. You can use theDisposeTimeBase
function to dispose of a time base once you are finished with it.Time bases rely on either a clock component or another time base for their time source. You can use the
SetTimeBaseMasterTimeBase
function to cause one time base to be based on another time base. TheGetTimeBaseMasterTimeBase
allows you to determine the master time base of a given time base.You can assign a clock component to a time base; that clock then acts as the master clock for the time base. You can use the
SetTimeBaseMasterClock
function to assign a clock component to a time base. TheGetTimeBaseMasterClock
function enables you to determine the clock component that is assigned to a time base. You can change the offset between a time base and its time source by calling theSetTimeBaseZero
function.You can set the time source of a movie by calling the
SetMovieMasterTimeBase
andSetMovieMasterClock
functions.
Working With Time Base Values
Every time base contains a rate, a start time, a stop time, a current time, and some status information. The Movie Toolbox provides a number of functions that allow your application to work with the contents of a time base. You can use these functions:
The
GetTimeBaseTime
function lets you retrieve the current time value of a time base. You can set the current time value by calling theSetTimeBaseTime
function; this function requires you to provide a time structure. Alternatively, you can set the current time based on a time value by calling theSetTimeBaseValue
function.You can determine the rate of a time base by calling the
GetTimeBaseRate
function. You can set the rate of a time base by calling theSetTimeBaseRate
function. You can determine the effective rate of a specified time base (relative to the master time base to which it is subordinate) by calling theGetTimeBaseEffectiveRate
function.You can retrieve the start time of a time base by calling the
GetTimeBaseStartTime
function. You can set the start time of a time base by calling theSetTimeBaseStartTime
function. Similarly, you can use theGetTimeBaseStopTime
andSetTimeBaseStopTime
functions to work with the stop time of a time base.
The Movie Toolbox also provides functions that allow you to work with the status information of a time base:
The
GetTimeBaseStatus
function allows you to read the current status of a time base.The
GetTimeBaseFlags
function helps you obtain the control flags of a time base. You can set these flags by calling theSetTimeBaseFlags
function.
Time Structures
The Movie Toolbox provides a number of functions that allow you to work with time structures:
You can use the
ConvertTime
function to convert a time you obtain from one time base into a time that is relative to another time base. Similarly, you can use theConvertTimeScale
function to convert a time from one time scale to another.You can add two times by calling the
AddTime
function; you can subtract two times with theSubtractTime
function.
All of these functions work with time structures. You can use time structures to represent either time values or durations. Time values specify a point in time, relative to a given time base. Durations specify a span of time, relative to a given time scale. Durations are represented by time structures that have the time base set to 0 (that is, the base
field in the time structure is set to nil
).
The TimeRecord Structure
Many time management functions require that you place a time specification in a data structure called a TimeRecord
.
struct TimeRecord |
{ |
CompTimeValue value; /* time value (duration or absolute) */ |
TimeScale scale; /* units per second */ |
TimeBase base; /* reference to the time base */ |
}; |
typedef struct TimeRecord TimeRecord; |
Field |
Description |
---|---|
|
Contains the time value. The time value defines either a duration or an absolute time by specifying the corresponding number of units of time. For durations, this is the number of time units in the period. For an absolute time, this is the number of time units since the beginning of the time coordinate system. The unit for this value is defined by the scale field. The time value is expressed as a |
|
Contains the time scale. This field specifies the number of units of time that pass each second. If you specify a value of 0, the time base uses its natural time scale. |
|
Contains a reference to the time base. You obtain a time base by calling the Movie Toolbox's |
Large Time Values
You can specify the time value in a time structure as a 64-bit integer value as follows:
typedef Int64 CompTimeValue; |
The Movie Toolbox uses this format so that extremely large time values can be represented. The Int64
data type defines the format of these signed 64-bit integers.
struct Int64 |
{ |
long hi; /* high-order 32 bits-value field in time structure */ |
long lo; /* low-order 32 bits-value field in time structure */ |
}; |
typedef struct Int64 Int64; |
Field |
Description |
---|---|
|
Contains the high-order 32 bits of the value. The high-order bit represents the sign of the 64-bit integer. |
|
Contains the low-order 32 bits of the value. |
Time Base Callback Functions
If your application uses QuickTime time bases, it may define callback functions that are associated with a specific time base. Your application can then use these callback functions to perform activities that are triggered by temporal events, such as a certain time being reached or a specified rate being achieved. The time base functions of the Movie Toolbox interact with clock components to schedule the invocation of these callback functions; clock components are responsible for invoking the callback function at its scheduled time. Your application can use the functions described in this section to establish your own callback function and to schedule callback events.
You can define three types of callback events. These types are distinguished by the nature of the temporal event that triggers the Movie Toolbox to call your function. The three types are
events that are triggered at a specified time
events that are triggered when the rate reaches a specified value
events that are triggered when the time value of a time base changes by an amount different from the time base’s rate.
Use these functions to work with time base callbacks:
You specify a callback event’s type when you define the callback event, using the
NewCallBack
function.You specify whether your event can occur at interrupt time when you define the callback event, using the
NewCallBack
function. Your function is called closer to the triggering event at interrupt time, but it is subject to all the restrictions of interrupt functions (for example, your callback function cannot cause memory to be moved). If your function is not called at interrupt time, you are free of these restrictions; but your function may be called later, because the invocation is delayed to avoid interrupt time.The
NewCallBack
function allocates the memory to support a callback event. When you are done with the callback event, you dispose of it by calling theDisposeCallBack
function.You schedule a callback event by calling the
CallMeWhen
function. CallCancelCallBack
function to unschedule a callback event.You can retrieve the time base of a callback event by calling the
GetCallBackTimeBase
function.You can obtain the type of a callback event by calling the
GetCallBackType
function.
Time and Space Function Summary
Here is a summary of the Movie Toolbox functions you can use to manage movie time and spatial characteristics.
Managing Movie Space
The Movie Toolbox contains a number of functions that your application can use to determine or change the spatial display characteristics of movies and tracks. These functions affect the movie’s graphics world, its matrix, the final display coordinate system, and the display boundaries. They include
SetTrackGWorld
SetMovieGWorld
GetMovieGWorld
SetMovieBox
GetMovieBox
GetMovieDisplayBoundsRgn
GetMovieSegmentDisplayBoundsRgn
SetMovieDisplayClipRgn
GetMovieDisplayClipRgn
GetTrackSegmentDisplayBoundsRgn
SetTrackLayer
GetTrackLayer
SetMovieMatrix
GetMovieMatrix
GetMovieBoundsRgn
GetTrackMovieBoundsRgn
GetMovieClipRgn
SetTrackMatrix
GetTrackMatrix
GetTrackBoundsRgn
SetTrackDimensions
GetTrackDimensions
SetTrackClipRgn
GetTrackClipRgn
SetTrackMatte
GetTrackMatte
DisposeMatte
SetMovieColorTable
GetMovieColorTable
Working With Matrices
Several Movie Toolbox functions allow you to manipulate transformation matrices.
SetIdentityMatrix
GetMatrixType
CopyMatrix
EqualMatrix
TranslateMatrix
ScaleMatrix
RotateMatrix
SkewMatrix
ConcatMatrix
InverseMatrix
TransformPoints
TransformFixedPoints
TransformRect
TransformFixedRect
TransformRgn
RectMatrix
MapMatrix
Managing Movie Time
Several functions are used to work with a movie’s time parameters. Included are functions for retrieving the time base, working with the current movie time, working with the time scale, calculating the movie’s duration, and getting and setting the playback rate.
GetMovieDuration
SetMovieTimeValue
SetMovieTime
GetMovieTime
SetMovieRate
GetMovieRate
SetMovieTimeScale
GetMovieTimeScale
GetMovieTimeBase
Managing Track Time
Several functions work with a track’s time parameters. All tracks share the movie’s time base, but each track contains its own offset and duration. A function is also provided to translate track time into a value appropriate to the track’s media.
GetTrackDuration
SetTrackOffset
GetTrackOffset
TrackTimeToMediaTime
Managing Media Time
Three functions work with a media’s time parameters. Each media has its own time scale and duration.
GetMediaDuration
SetMediaTimeScale
GetMediaTimeScale
Finding Interesting Times
You can call Movie Toolbox functions to search a movie, track or media for a particular sample, such as a keyframe. The functions return the time and duration of the next sample that meets the search criteria.
GetMovieNextInterestingTime
GetTrackNextInterestingTime
GetMediaNextInterestingTime
Time Base Management
Various Movie Toolbox functions work with time bases. A QuickTime time base defines the time coordinate system for a movie. It can also be used to provide general timing services.
Creating and Disposing of Time Bases
NewTimeBase
DisposeTimeBase
SetMovieMasterClock
SetMovieMasterTimeBase
SetTimeBaseMasterClock
GetTimeBaseMasterClock
SetTimeBaseMasterTimeBase
GetTimeBaseMasterTimeBase
SetTimeBaseZero
-
SetTimeBaseTime
SetTimeBaseValue
SetTimeBaseRate
GetTimeBaseRate
GetTimeBaseEffectiveRate
SetTimeBaseStartTime
GetTimeBaseStartTime
SetTimeBaseStopTime
GetTimeBaseStopTime
SetTimeBaseFlags
GetTimeBaseFlags
GetTimeBaseStatus
-
AddTime
SubtractTime
ConvertTime
ConvertTimeScale
-
NewCallBack
CallMeWhen
CancelCallBack
DisposeCallBack
GetCallBackTimeBase
GetCallBackType
Most of these functions are discussed in the preceding sections of this chapter.
Copyright © 2005, 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-06-04