Retired Document
Important: This document may not represent best practices for current development. Links to downloads and other resources may no longer be valid.
Target-Action
Target-action is a design pattern in which an object holds the information necessary to send a message to another object when an event occurs. The stored information consists of two items of data: an action selector, which identifies the method to be invoked, and a target, which is the object to receive the message. The message sent when the event occurs is called an action message. Although the target can be any object, even a framework object, it is typically a custom controller that handles the action message in an application-specific way.
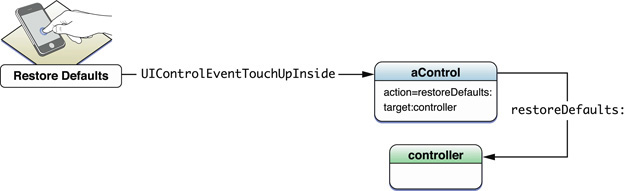
The event triggering an action message can be anything, just as the object sending the message can be any object. For example, a gesture-recognizer object might send an action message to another object when it recognizes its gesture. However, the target-action paradigm is most commonly found with controls such as buttons and sliders. When a user manipulates a control object, it sends the message to the specified object. The control object is an instance of a subclass of UIControl
(iOS) or NSControl
(OS X). Both action selector and target object are properties of a control object or, in the AppKit framework, properties of the control’s cell object.
An Action Method Must Have a Certain Form
Action methods must have a conventional signature. The UIKit framework permits some variation of signature, but both platforms accept action methods with a signature similar to the following:
- (IBAction)doSomething:(id)sender; |
The type qualifier IBAction
, which is used in place of the void
return type, flags the declared method as an action so that Interface Builder is aware of it. For an action method to appear in Interface Builder, you first must declare it in a header file of the class whose instance is to receive the action message.
The sender
parameter is the control object sending the action message. When responding to an action message, you may query sender
to get more information about the context of the event triggering the action message.
You Can Set Target and Action in Code or Using the Tools
You can set the action and target of a control (or cell) object programmatically or in Interface Builder. Setting these properties effectively connects the control and its target via the action. If you connect a control and its target in Interface builder, the connection is archived in a nib file. When an application later loads the nib file, the connection is restored.
You may set the target of an action message to nil
. In this case, the application determines the target at runtime; it sends an action message first to the first responder and from there it goes up the responder chain until it is handled (if at all).
Target-Action is Different in iOS and OS X
Although conceptually the target-action design pattern is the same for both frameworks, UIKit and AppKit implement it differently:
In UIKit, a control maps a target and action to one or more multitouch events that can occur on the control.
UIKit allows several different signatures for action methods. For example, the following signature is permissible:
- (IBAction)action:(id)sender forEvent:(UIEvent *)event
AppKit uses the control-cell architecture for implementing target-action for most (but not all) controls. In this architecture, a control “owns” one or more lighter-weight cell objects, and the cell holds the target and action properties for its control. When the user clicks or otherwise activates a control, it extracts this information from its cell and sends the action message.
Copyright © 2018 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2018-04-06