Scrolling Using Paging Mode
The UIScrollView
class supports a paging mode, which restricts a user initiated scroll action to scrolling a single screens worth of content at a time. This mode is used when displaying sequential content, such as an eBook or a series of instructions.
Configuring Paging Mode
Configuring a scroll view to support paging mode requires that code be implemented in the scroll view’s controller class.
Aside from the standard scroll view initialization described in Creating and Configuring Scroll Views, you must also set the pagingMode
property to YES
.
The contentSize
property of a paging scroll view is set so that it fills the height of the screen and that the width is a multiple of the width of the device screen multiplied by the number of pages to be displayed.
Additionally, the scroll indicators should be disabled, because the relative location as the user is touching the screen is irrelevant, or is shown using a UIPageControl
.
Figure 5-1 shows an example of a scroll view configured in paging mode. The implementation of the shown application is available in the PageControl: Using a Paginated UIScrollView sample code.
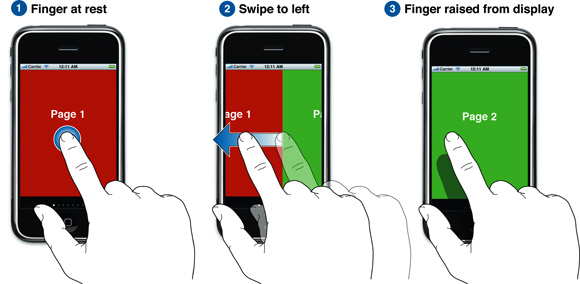
Configuring Subviews of a Paging Scroll View
The subviews of a paging scroll view can be configured in one of two ways. If the content is small, you could draw the entire contents at once, in a single view that is the size of the scroll view’s contentSize
. While this is the easiest to implement, it is not efficient when dealing with large content areas, or page content that takes time to draw.
When your application needs to display a large number of pages or drawing the page content can take some time, your application should use multiple views to display the content, one view for each page. This is more complicated, but can greatly increase performance and allows your application to support much larger display sets. The PageControl: Using a Paginated UIScrollView example uses this multiple view technique. By examining the sample code, you can see exactly how this technique can be implemented.
Supporting a large number of pages in a paging scroll view can be accomplished using only three view instances, each the size of the device screen: one view displays current page, another displays the previous page, and third displays the next page. The views are reused as the user scrolls through the pages.
When the scroll view controller is initialized, all three views are created and initialized. Typically the views are a custom subclass of UIView, although an application could use instances of UIImageView
if appropriate. The views are then positioned relative to each so that when the user scrolls, the next or previous page is always in place and the content is ready for display. The controller is responsible for keeping track of which page is the current page.
To determine when the pages need to be reconfigured because the user is scrolling the content, the scroll view requires a delegate that implements the scrollViewDidScroll:
method. The implementation of this method should track the contentOffset
of the scroll view, and when it passes the mid point of the current view’s width, the views should be reconfigured, moving the view that is no longer visible on the screen to the position that represents the next and previous page (depending on the direction the user scrolled). The delegate should then inform the view that it should draw the content appropriate for the new location it the represents.
By using this technique, you can display a large amount of content using a minimum of resources.
If drawing the page content is time consuming, your application could add additional views to the view pool, positioning those as pages on either side of the next and previous pages as scrolling occurs, and then draw the page content of those additional pages when the current content scrolls.
Copyright © 2011 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2011-06-06