User Interaction: Prompting for and Displaying Data
The Address Book UI framework provides three view controllers and one navigation controller for common tasks related to working with the Address Book database and contact information. By using these controllers rather than creating your own, you reduce the amount of work you have to do and provide your users with a more consistent experience.
This chapter includes some short code listings you can use as a starting point. For a fully worked example, see QuickContacts.
What’s Available
The Address Book UI framework provides four controllers:
ABPeoplePickerNavigationController
prompts the user to select a person record from their address book.ABPersonViewController
displays a person record to the user and optionally allows editing.ABNewPersonViewController
prompts the user create a new person record.ABUnknownPersonViewController
prompts the user to complete a partial person record, optionally allows them to add it to the address book.
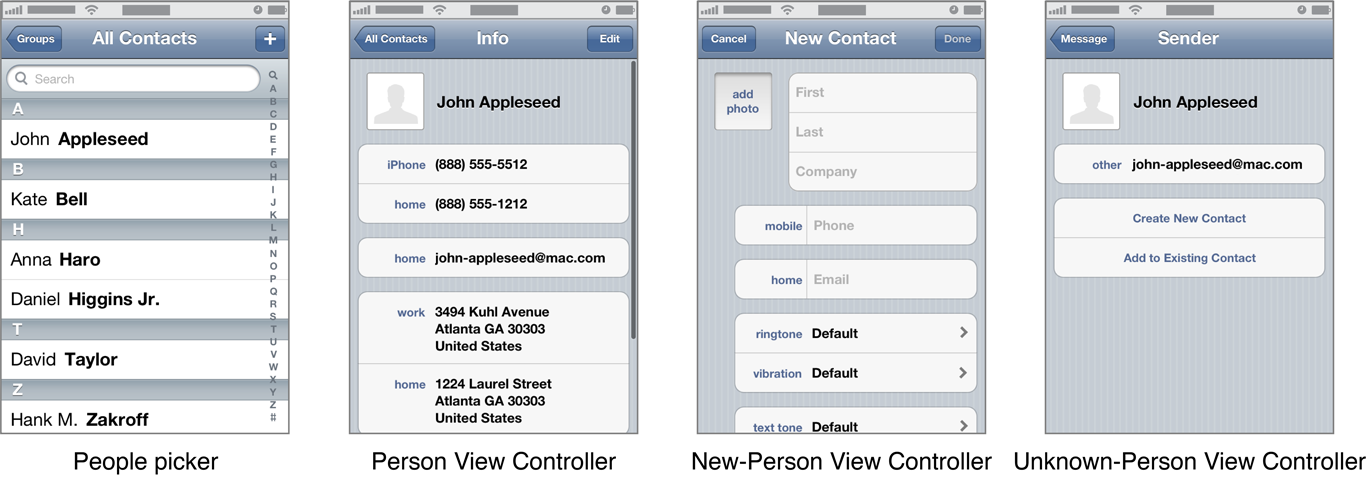
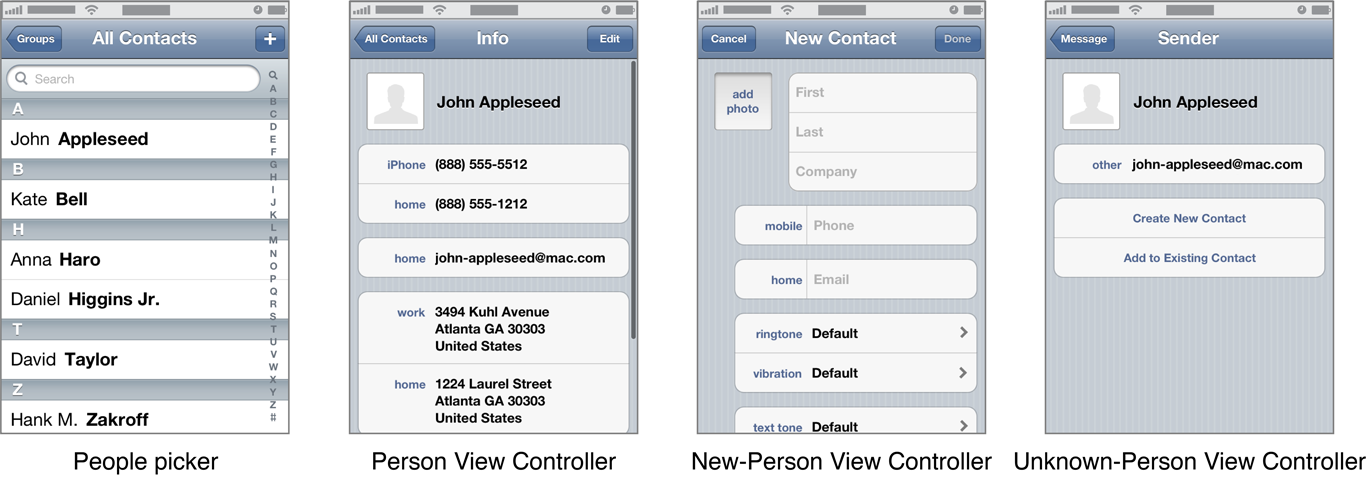
To use these controllers, you must set a delegate for them which implements the appropriate delegate protocol. You should not need to subclass these controllers; the expected way to modify their behavior is by your implementation of their delegate. In this chapter, you will learn more about these controllers and how to use them.
Prompting the User to Choose a Person Record
The ABPeoplePickerNavigationController
class allows users to browse their list of contacts and select a person and, at your option, one of that person’s properties. To use a people picker, do the following:
Create and initialize an instance of the class.
Set the delegate, which must adopt the
ABPeoplePickerNavigationControllerDelegate
protocol.Optionally, set
displayedProperties
to the array of properties you want displayed. The relevant constants are defined as integers; wrap them in anNSNumber
object using thenumberWithInt:
method to get an object that can be put in an array.Present the people picker as a modal view controller using the
presentModalViewController:animated:
method. It is recommended that you present it using animation.
The following code listing shows how a view controller which implements the ABPeoplePickerNavigationControllerDelegate
protocol can present a people picker:
ABPeoplePickerNavigationController *picker = |
[[ABPeoplePickerNavigationController alloc] init]; |
picker.peoplePickerDelegate = self; |
[self presentModalViewController:picker animated:YES]; |
The people picker calls one of its delegate’s methods depending on the user’s action:
If the user cancels, the people picker calls the method
peoplePickerNavigationControllerDidCancel:
of the delegate, which should dismiss the people picker.If the user selects a person, the people picker calls the method
peoplePickerNavigationController:shouldContinueAfterSelectingPerson:
of the delegate to determine if the people picker should continue. To prompt the user to choose a specific property of the selected person, returnYES
. Otherwise returnNO
and dismiss the picker.If the user selects a property, the people picker calls the method
peoplePickerNavigationController:shouldContinueAfterSelectingPerson:property:identifier:
of the delegate to determine if it should continue. To perform the default action (dialing a phone number, starting a new email, etc.) for the selected property, returnYES
. Otherwise returnNO
and dismiss the picker using thedismissModalViewControllerAnimated:
method. It is recommended that you dismiss it using animation..
Displaying and Editing a Person Record
The ABPersonViewController
class displays a record to the user. To use this controller, do the following:
Create and initialize an instance of the class.
Set the delegate, which must adopt the
ABPersonViewControllerDelegate
protocol. To allow the user to edit the record, setallowsEditing
toYES
.Set the
displayedPerson
property to the person record you want to display.Optionally, set
displayedProperties
to the array of properties you want displayed. The relevant constants are defined as integers; wrap them in anNSNumber
object using thenumberWithInt:
method to get an object that can be put in an array.Display the person view controller using the
pushViewController:animated:
method of the current navigation controller. It is recommended that you present it using animation.
The following code listing shows how a navigation controller can present a person view controller:
ABPersonViewController *view = [[ABPersonViewController alloc] init]; |
view.personViewDelegate = self; |
view.displayedPerson = person; // Assume person is already defined. |
[self.navigationController pushViewController:view animated:YES]; |
If the user taps on a property in the view, the person view controller calls the personViewController:shouldPerformDefaultActionForPerson:property:identifier:
method of the delegate to determine if the default action for that property should be taken. To perform the default action for the selected property, such as dialing a phone number or composing a new email, return YES
; otherwise return NO
.
Prompting the User to Create a New Person Record
The ABNewPersonViewController
class allows users to create a new person. To use it, do the following:
Create and initialize an instance of the class.
Set the delegate, which must adopt the
ABNewPersonViewControllerDelegate
protocol. To populate fields, set the value ofdisplayedPerson
. To put the new person in a particular group, setparentGroup
.Create and initialize a new navigation controller, and set its root view controller to the new-person view controller
Present the navigation controller as a modal view controller using the
presentModalViewController:animated:
method. It is recommended that you present it using animation.
The following code listing shows how a navigation controller can present a new person view controller:
ABNewPersonViewController *view = [[ABNewPersonViewController alloc] init]; |
view.newPersonViewDelegate = self; |
UINavigationController *newNavigationController = [[UINavigationController alloc] |
initWithRootViewController:view]; |
[self presentModalViewController:newNavigationController |
animated:YES]; |
When the user taps the Save or Cancel button, the new-person view controller calls the method newPersonViewController:didCompleteWithNewPerson:
of the delegate, with the resulting person record. If the user saved, the new record is first added to the address book. If the user cancelled, the value of person
is NULL
. The delegate must dismiss the new-person view controller using the navigation controller’s dismissModalViewControllerAnimated:
method. It is recommended that you dismiss it using animation.
Prompting the User to Create a New Person Record from Existing Data
The ABUnknownPersonViewController
class allows the user to add data to an existing person record or to create a new person record for the data. To use it, do the following:
Create and initialize an instance of the class.
Create a new person record and populate the properties to be displayed.
Set
displayedPerson
to the new person record you created in the previous step.Set the delegate, which must adopt the
ABUnknownPersonViewControllerDelegate
protocol.To allow the user to add the information displayed by the unknown-person view controller to an existing contact or to create a new contact with them, set
allowsAddingToAddressBook
toYES
.Display the unknown-person view controller using the
pushViewController:animated:
method of the navigation controller. It is recommended that you present it using animation.
The following code listing shows how you can present an unknown-person view controller:
ABUnknownPersonViewController *view = [[ABUnknownPersonViewController alloc] init]; |
view.unknownPersonViewDelegate = self; |
view.displayedPerson = person; // Assume person is already defined. |
view.allowsAddingToAddressBook = YES; |
[self.navigationController pushViewController:view animated:YES]; |
When the user finishes creating a new contact or adding the properties to an existing contact, the unknown-person view controller calls the method unknownPersonViewController:didResolveToPerson:
of the delegate with the resulting person record. If the user canceled, the value of person
is NULL
.
Copyright © 2013 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2013-08-08