Retired Document
Important: This document is retired. Use the information in View Controller Programming Guide for iOS instead.
View Controller Basics
View controllers provide the fundamental infrastructure you need to implement iOS applications. This chapter provides an overview of the role view controllers play in your application and how you use them to implement different types of user interfaces.
What Is a View Controller?
In the Model-View-Controller (MVC) design pattern, a controller object provides the custom logic needed to bridge the application’s data to the views and other visual entities used to present that data to the user. In iOS applications, a view controller is a specific type of controller object that you use to present and manage a set of views. View controller objects are descendants of the UIViewController
class, which is defined in the UIKit framework.
View controllers play a very important role in the design and implementation of iOS applications. Applications running on iOS–based devices have a limited amount of screen space for displaying content and therefore must be creative in how they present information to the user. Applications that have lots of content may have to distribute that content across multiple screens or show and hide different parts of content at different times. View controller objects provide the infrastructure for managing your content-related views and for coordinating the showing and hiding of them.
There are many reasons to use view controllers in your application and very few reasons to avoid them. View controllers make it easier for you to implement many of the standard interface behaviors found in iOS applications. They provide default behavior that you can use as is or customize when needed. They also provide a convenient way to organize your application’s user interface and content.
Figure 1-1 shows an example of three different (but related) screens from an iPhone application that manages recipes. The first screen lists the recipes that the application manages. Tapping one of the recipes displays the second screen, which shows the details of the recipe. Tapping the recipe’s picture in this detail view displays the third screen, which displays a picture of the resulting dish that fills the screen. Managing each of these screens is a distinct view controller object whose job is to present the appropriate view objects, populate those views with data, and respond to interactions with that view.
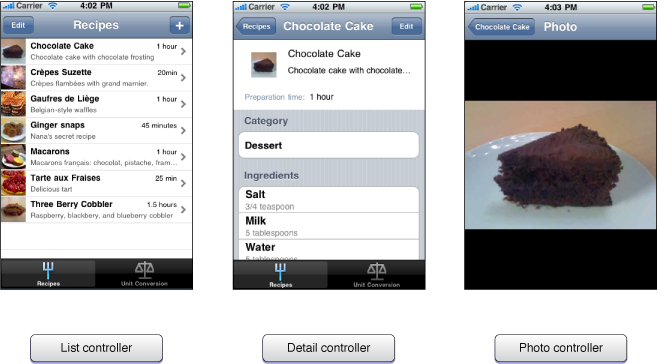
In addition to displaying and managing views, you can also use view controllers to manage the navigation from screen to screen. In iOS, there are several standard techniques for presenting new screens. All of these techniques are implemented using view controllers and are discussed at different points throughout this document.
Types of View Controllers
Most iOS applications have at least one view controller and some have several. Broadly speaking, view controllers are divided into three general categories that reflect the role the view controller plays in your application.
A custom view controller is a controller object that you define for the express purpose of presenting some content on the screen. Most iOS applications present data using several distinct sets of views, each of which handles the presentation of your data in a specific way. For example, you might have a set of views that presents a list of items in a table and another set that displays the details for a single item in that list. The corresponding architecture for such an application would involve the creation of separate view controllers to manage the marshaling and display of each set of views.
A container view controller is a specific type of view controller object that manages other view controllers and defines the navigational relationships among them. Navigation, tab bar, and split view controllers are all examples of container view controllers. You do not define container view controllers yourself. Instead, you use the container view controllers provided by the system as is.
A modal view controller is a view controller (container or custom) that is presented in a specific way by another view controller. Modal view controllers define a specific navigational relationship in your application. The most common reason to present a view controller modally is so that you can prompt the user to input some data. For example, you might present a view controller modally to have the user fill in a form or select an option from a picker interface. However, there are other uses for modal view controllers that are described in more detail in Modal View Controllers.
Figure 1-2 shows the view controller classes available in the UIKit framework along with some of the key classes used in conjunction with view controllers. These additional classes are often used internally by view controller objects to implement special types of interfaces. For example, the UITabBarController
object manages a UITabBar
object, which actually displays the tabs associated with the tab bar interface. Other frameworks may also define additional view controller objects in order to present specific types of interfaces.
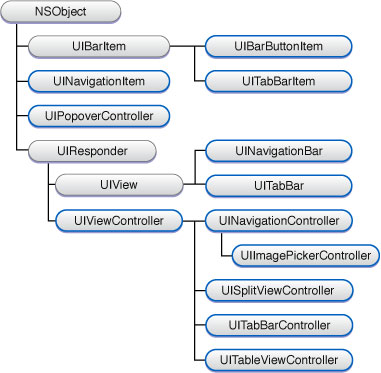
The sections that follow provide more detail about the types of view controllers you use to organize and present your application’s content.
About Custom View Controllers
Custom view controllers are the primary coordinating objects for your application’s content. Nearly every application has at least one custom view controller, and a complex application might have many of them. A custom view controller contains the logic and glue code needed to facilitate interactions between a portion of your application’s data and the views used to present that data. The view controller may also interact with other controller objects in your application, including the application delegate and other view controllers.
Each custom view controller object you create is responsible for managing all of the views in a single view hierarchy. In iPhone applications, the views in a view hierarchy traditionally cover the entire screen, but in iPad applications they may cover only a portion of the screen. The one-to-one correspondence between a view controller and the views in its view hierarchy is the key design consideration. You should not use multiple custom view controllers to manage different portions of the same view hierarchy. Similarly, you should not use a single custom view controller object to manage multiple screens worth of content.
You create a custom view controller by subclassing UIViewController
directly and adding custom code to your subclass. The declaration of a typical UIViewController
subclass includes things such as the following:
Member variables pointing to the objects containing the data to be displayed by the corresponding views
Member variables (or outlets) pointing to key view objects with which your view controller must interact
Action methods that perform tasks associated with buttons and other controls in the view hierarchy
Any additional methods needed to implement your view controller’s custom behavior
Because you use it to manage your custom content, most of the code in this type of view controller is going to be specific to your application. However, there are also some common behaviors that all view controllers can support. For these common behaviors, the UIViewController
class defines methods that you can override and use to implement the desired behavior. Some of the common behaviors include view management, interface rotation management, and low-memory warning support.
Figure 1-3 shows an example of a custom view controller in the sample project BubbleLevel. This application defines the LevelViewController
class, which is a direct descendant of UIViewController
. This class monitors the accelerometer data for changes in the pitch of the device and uses that data to update its associated view object. The view
property of the view controller provides a reference to the actual view object presenting the content.
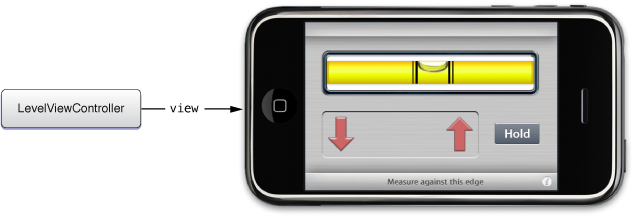
For information about managing the standard behaviors required of all view controllers, see Custom View Controllers.
About Table View Controllers
The UITableViewController
class is another type of custom view controller designed specifically for managing tabular data. Although it is certainly possible to manage tables without a table view controller, the class adds automatic support for many standard table-related behaviors such as selection management, row editing, table configuration, and others. This additional support is there to minimize the amount of code you have to write to create and initialize your table-based interface. You can use a table view controller in the same places you would use a custom view controller. You can also subclass it and implement additional custom behaviors. Of course, any view hierarchy managed by such a view controller should include a table view object.
Figure 1-4 shows an example of the configuration of a table view controller. Because it is a subclass of UIViewController
, the table view controller still has a pointer to the root view of the interface (through its view
property) but it also has a separate pointer to the table view displayed in that interface.
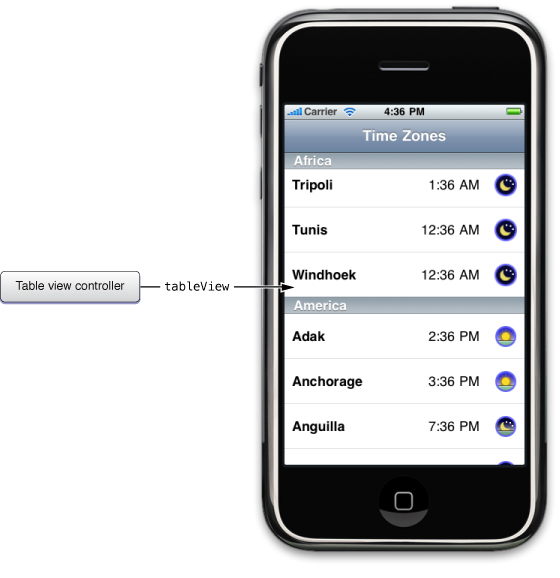
This document covers only the behaviors that are common to all view controllers and does not cover any information specific to table view controllers. For specific information about the table-related behaviors of a table view controller, see UITableViewController Class Reference. For more information about managing table views in general, see Table View Programming Guide for iOS.
About Navigation Controllers
A navigation controller is a container view controller that you use to present data that is organized hierarchically. A navigation controller is an instance of the UINavigationController
class, which is a class you use as is and do not subclass. The methods of this class provide support for managing a stack-based collection of custom view controllers. This stack represents the path taken by the user through the hierarchical data, with the bottom of the stack reflecting the starting point and the top of the stack reflecting the user’s current position in the data.
Although the navigation controller’s primary job is to act as a manager of other view controllers, it also manages a few views. Specifically, it manages a navigation bar that displays information about the user’s current location in the data hierarchy, a back button for navigating to previous screens, and any custom controls the current view controller needs. The navigation controller also manages an optional toolbar, which can be used to display commands related to the current screen. You do not modify these views directly in most cases but configure them through support found in the UIViewController
class.
Figure 1-5 shows some screens from the Contacts application, which uses a navigation controller to present contact information to the user. Each screen displayed to the user is managed by a custom view controller object, which presents information at that specific level of the data hierarchy. For example, the root view controller and list view controllers manage the presentation of tabular contact information in different ways. The detail view controller displays the information for a specific contact using an entirely different type of screen. As the user interacts with controls in the interface, those controls tell the navigation controller to display the next view controller in the sequence or dismiss the current view controller.
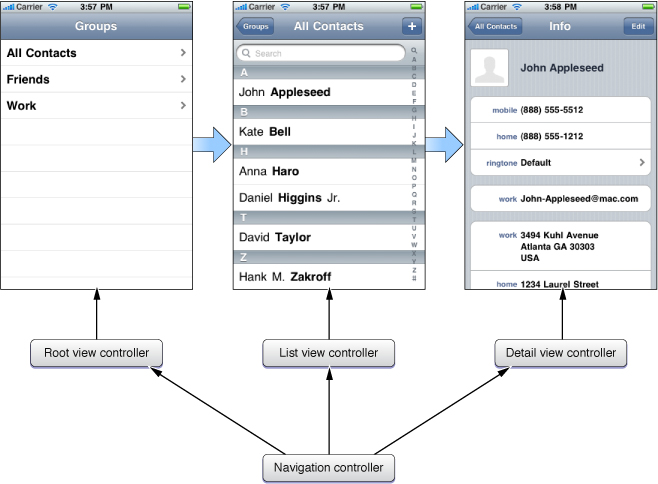
For information about how to configure and use navigation controller objects, see Navigation Controllers.
About Tab Bar Controllers
A tab bar controller is a container view controller that you use to divide your application into two or more distinct modes of operation. A tab bar controller is an instance of the UITabBarController
class, which is a class you use as is and do not subclass. The modes of the tab bar controller are presented using a tab bar view, which displays a tab for each supported mode. Selecting a tab causes an associated view controller to present its interface on the screen.
You use tab bar controllers in situations where your application either presents different types of data or presents the same data in significantly different ways. The tab bar controller facilitates the automatic switching of modes in response to user taps on the tab bar view. If there are more modes than there is space for tabs, the tab bar controller also manages the selection of tabs that are not normally visible and the customization of the tabs that are visible.
Figure 1-6 shows several modes of the Clock application along with the relationships between the corresponding view controllers. Each mode has a root view controller to manage the main content area. In the case of the Clock application, the Clock and Alarm view controllers both display a navigation-style interface to accommodate some additional controls along the top of the screen. The other modes use custom view controllers to present a single screen.
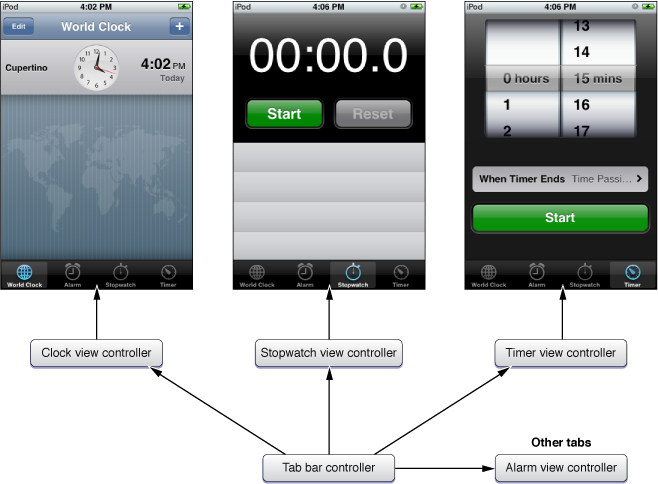
For information about how to configure and use a tab bar controller, see Tab Bar Controllers.
About Split View Controllers
A split-view controller is a container view controller that is typically used to implement master-detail interfaces. A split view controller is an instance of the UISplitViewController
class, which is a class you use as is and do not subclass. The contents of a split view interface are derived from two view controllers that you provide. In landscape orientations, a split view controller displays the contents of two other view controllers side-by-side. In portrait orientations, it displays only one of the view controllers directly and makes the other one available from a popover.
Figure 1-7 shows a split view interface from the MultipleDetailViews sample application. The landscape version of the interface displays the list view and detail view side-by-side. In portrait mode, only the detail view is displayed and the list view is made available using a popover. Both the list view and detail view are managed by a custom view controller.
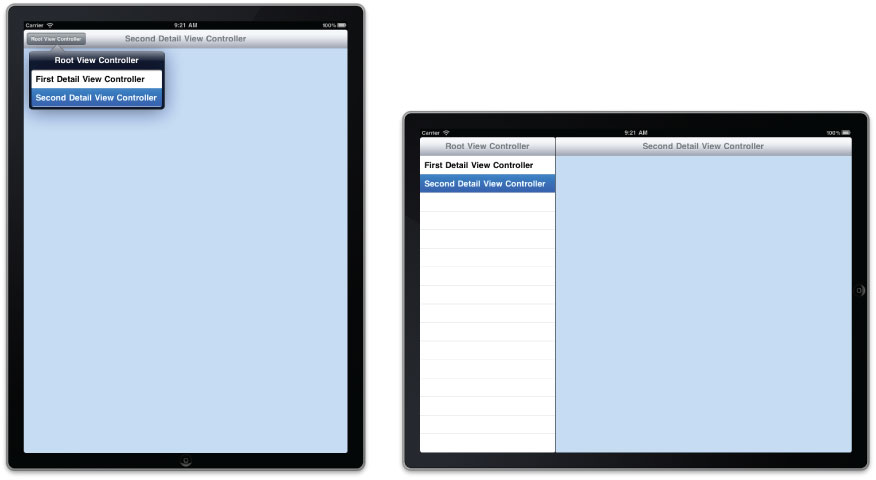
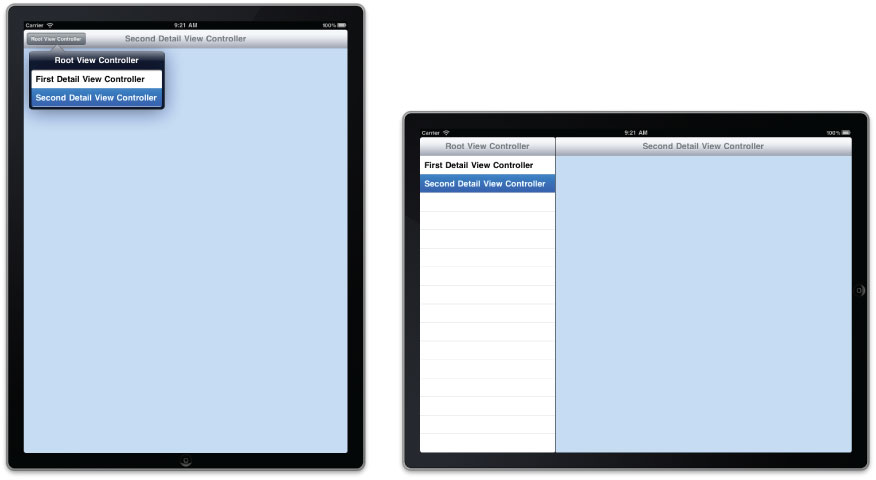
For information about how to configure and use a split view controller, see Split View Controller.
About Modal View Controllers
A modal view controller is not a specific view controller class but is a way of presenting any view controller to the user. Although container view controllers define specific relationships between the managed view controllers, modal view controllers let you define the relationship. Any view controller object can present any other view controller object modally. Most of the time, you present view controllers modally in order to gather information from the user or capture the user’s attention for some specific purpose. Once that purpose is completed, you dismiss the modal view controller and allow the user to continue navigating through your application.
Figure 1-8 shows an example from the Contacts application. When the user clicks the plus button to add a new contact, the Contacts view controller presents the New Contact view controller modally. This creates a parent-child relationship between the two view controllers. The New Contact screen remains visible until the user cancels the operation or provides enough information about the contact that it can be saved to the contacts database, at which point the Contacts view controller dismisses its child.
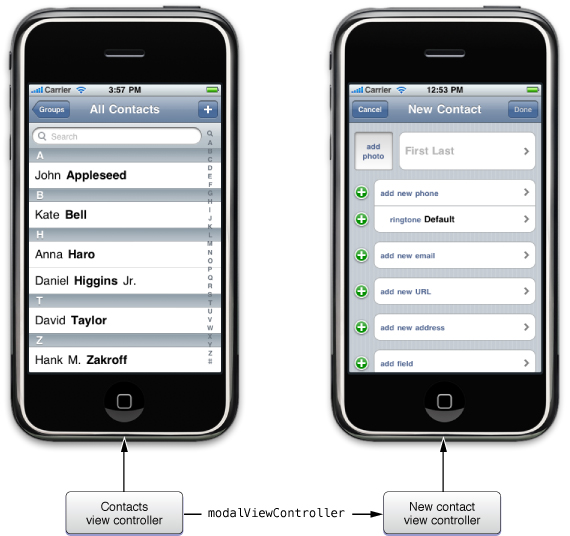
It is worth noting that a view controller presented modally can itself present another view controller modally. This ability to chain modal view controllers can be useful in situations where you might need to perform several modal actions sequentially. For example, if the user taps the Add Photo button in the New Contact screen in the preceding figure and wants to choose an existing image, the New Contact view controller presents an image picker interface modally. The user must dismiss the image picker screen and then dismiss the New Contact screen separately in order to return to the list of contacts.
For more information about the uses for modal view controllers and how to present them in your application, see Modal View Controllers.
Getting Started with the iOS Application Templates in Xcode
Most of the Xcode project templates for iOS applications provide you with at least one view controller class initially, and some may provide you with multiple view controllers. These initial classes provide you with the code found in a typical view controller and are intended to help you start writing your application quickly. It is important to remember though that the templates are just a starting point.
The goal of the template applications is to show you the best way to get started with specific types of applications. It is always easiest to start with the template that most closely matches the interface you are trying to create. For example, if you are creating an application similar to the Stocks or Weather applications, you would start with the Utility Application template. On the other hand, if you plan to use a tab bar to divide your application into different modes, you should start with the Tab Bar Application template.
If you want to explore basic view controller behaviors, the View-based Application template is a good place to start. This type of application uses a single custom view controller to display the contents of the application. You can expand on this basic behavior by presenting additional view controllers modally.
If you want to build your application’s user interface from scratch, start with the Window-based Application template. This template provides a minimally configured project that you can modify to include the view controllers you need.
For more information about creating projects in Xcode, see Xcode Project Management Guide.
Copyright © 2013 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2013-09-18