Adding In-App Purchase to Your Applications
In-App Purchase allows you to sell additional features and functionality from within your iOS, macOS, and tvOS apps. If you wish to offer in-app purchases in your applications, you must complete several steps before you can do it. This document provides step-by-step instructions for setting up and testing in-app purchase. It also answers common questions about in-app purchase. The "Agreements, Tax, and Banking Information" section describes all the financial documents that must be completed. The "Certificates, Identifiers & Profiles" and "iTunes Connect" sections indicate the steps to be respectively done in the Certificates, Identifiers & Profiles section of Account and iTunes Connect. The "What's Next" section shows how to test in-app purchase. The "Update Your App for Ask to Buy" section describes how to support the Ask to Buy feature.
This document does not cover how to implement in-app purchase in your applications. Read the In-App Purchase Programming Guide for detailed information about implementing in-app purchase in your applications.
Agreements, Tax, and Banking Information
You must complete the following steps before you can support in-app purchase in your applications:
Agree to the latest Developer Program License Agreement.
Your team agent must agree to the latest Apple Developer Program License Agreement in Account before you are allowed to create in-app purchases.
Complete your contract, tax, and banking Information.
You must have a Paid Applications contract in effect with Apple and have provided your tax and banking information in iTunes Connect as seen in Figure 1. Read Manage agreements, tax, and banking for more information.


Certificates, Identifiers & Profiles
The Certificates, Identifiers & Profiles section of Account is used to configure your App ID and Provisioning Profiles for in-app purchase. You must complete the following step in that section:
Register an explicit App ID for your application.
Explicit App IDs are App IDs whose Bundle Identifier portion is a string without the wildcard ("*") character. Furthermore, they are automatically registered for in-app purchase and Game Center as shown in Figure 2. Using an explicit App ID ensures that your in-app purchases are only associated with your application. For example, use
com.example.dinner
rather thancom.example.*
.Your team agent or admin should navigate to the App IDs section of Certificates, Identifiers & Profiles to create App IDs for your applications. Read Registering App IDs to find out how to create App IDs.
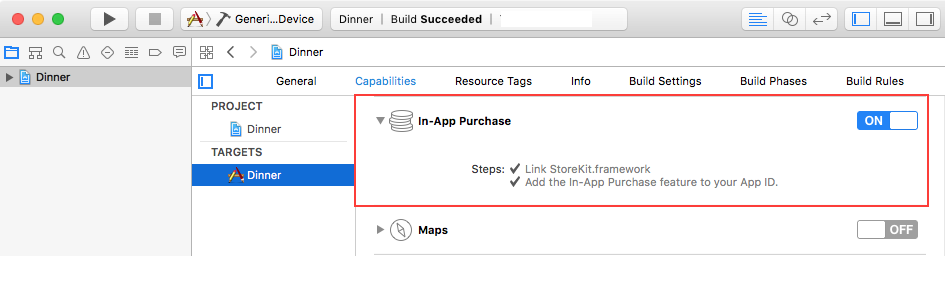
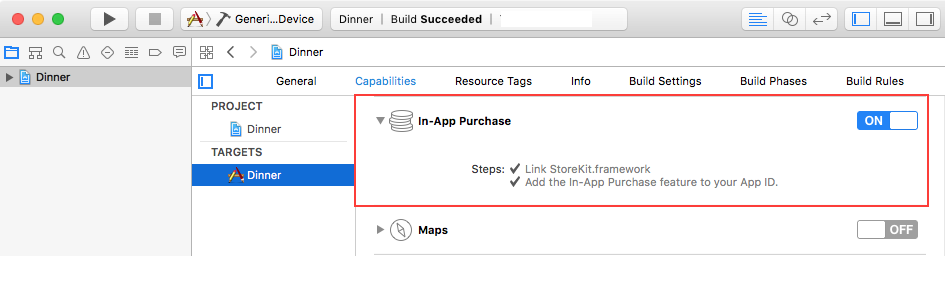
iTunes Connect
To test in-app purchase, you need to create products to purchase and test accounts to make the purchases. iTunes Connect allows you to create and manage in-app purchases and test user accounts. You must complete the following steps in iTunes Connect:
Create test user accounts.
Apple provides a testing environment, called the sandbox, which allows you to test your in-app purchases without incurring any financial charges. The sandbox environment uses special test user accounts rather than your regular iTunes Connect accounts to test in-app purchase. See Create a sandbox tester account for more information about creating test user accounts.
Create In-App Purchase products.
Creating in-app purchase products is available via the In-App Purchases feature for your app in iTunes Connect. This feature is only visible to users with admin or technical role in iTunes Connect. See Creating In-App Purchase Products for more information.
Fill out the In-App Purchases form.
The In-App Purchase form contains the Product ID field, which specifies a unique identifier for each of your in-app purchase products. See Technical Q&A QA1329, 'In-App Purchase Product Identifiers' for more information about product identifiers.
Leave the state of your product as Missing Metadata as shown in Figure 3.
Clear your product for sale.
The In-App Purchases form contains a Cleared for Sale checkbox, which determines whether your in-app purchase product will be available for purchase from within your application. Check that box to make sure your product is available for sale.
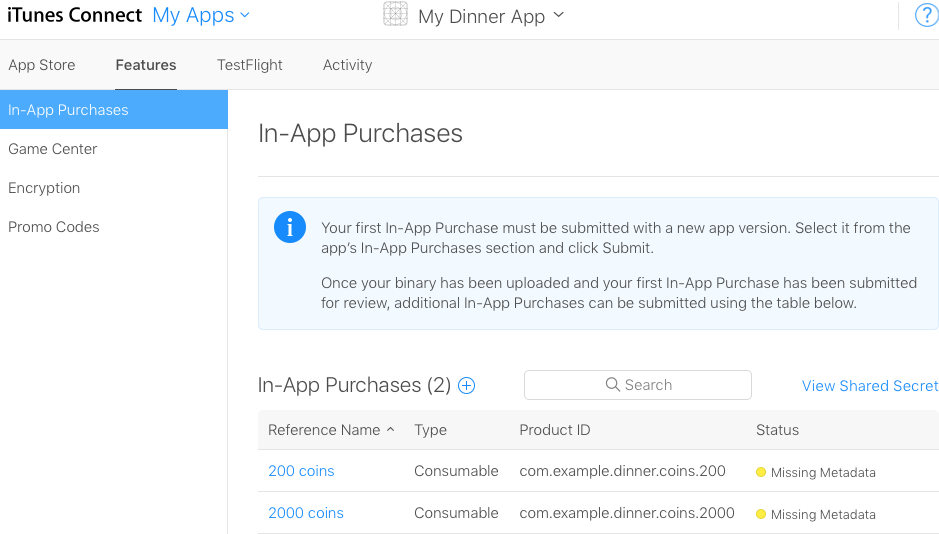
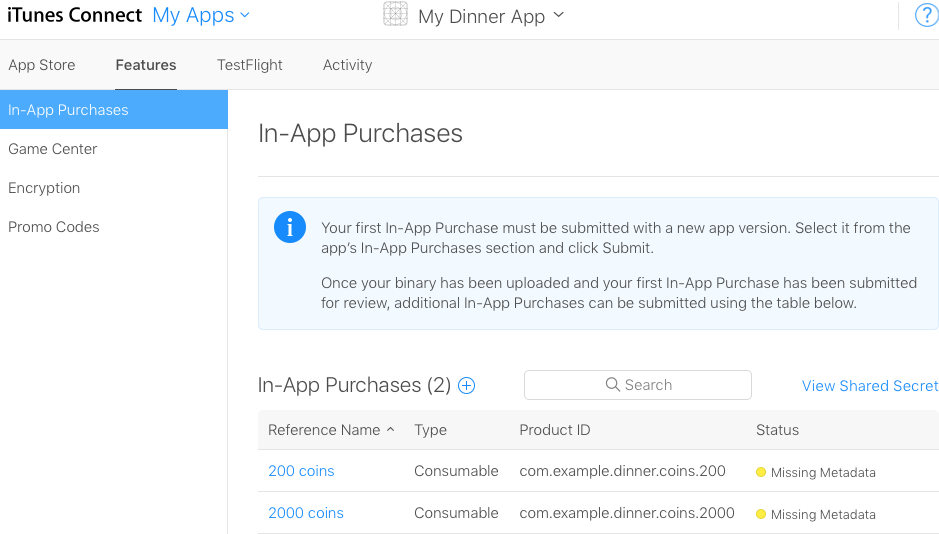
What's Next?
You have successfully set up in-app purchase for your application, let's implement and test it:
Launch or create your project in Xcode.
Enter the Bundle Identifier portion of your App ID in the Bundle Identifier field of your Target's Info pane in Xcode.
Enter a version number (CFBundleVersion) and a build number (CFBuildNumber) in the Version and Build fields of your Target's General Pane in Xcode, respectively as seen in Figure 4.
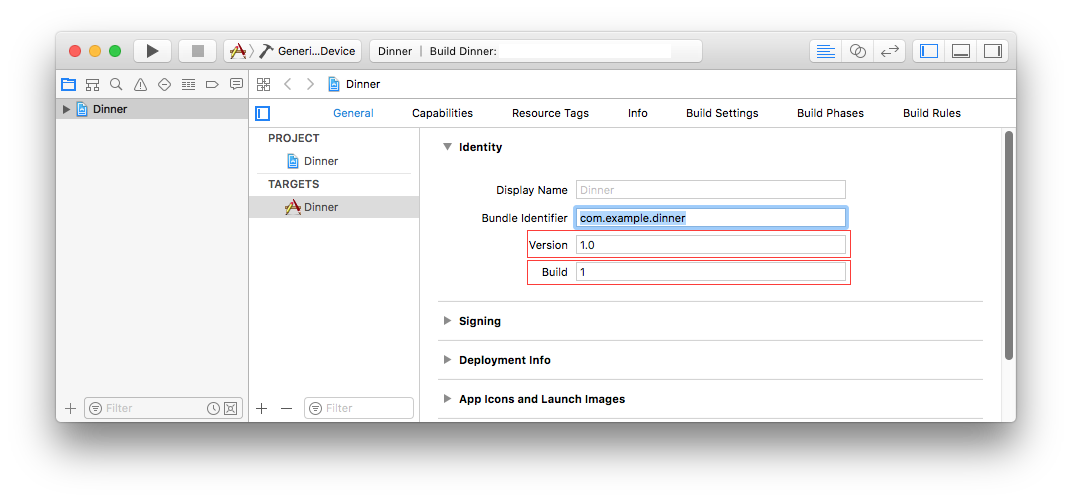
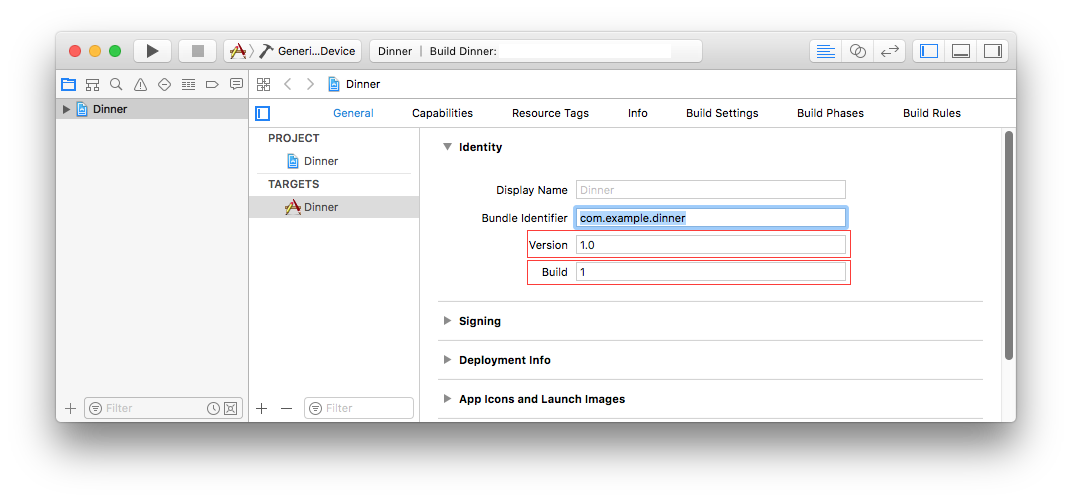
Set up your project to use automatic provisioning. Read Technical Q&A, QA1814, Setting up Xcode to automatically manage your provisioning profiles for more information.
Add the in-app purchase capability to your app as seen in Figure 5.
Xcode will automatically create a development provisioning profile enabled for in-app purchase. Read Enabling In-App Purchase (iOS, tvOS, Mac) for more information.
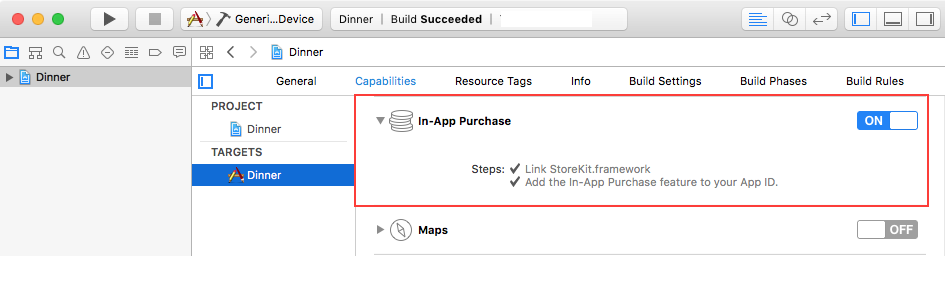
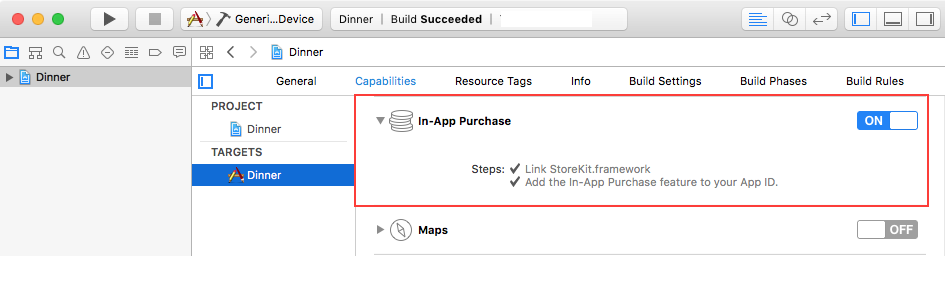
Write code for your application.
Read the In-App Purchase Programming Guide and Receipt Validation Programming Guide for detailed information about implementing in-app purchase in your applications and receipt validation, respectively.
macOS applications should perform receipt validation immediately after launch. The apps should call
exit
with a status of173
if validation fails as shown in Listing 1.Listing 1 Receipt validation
func applicationDidFinishLaunching(_ aNotification: Notification) {
// Test whether the app's receipt exists.
if let url = Bundle.main.appStoreReceiptURL, let _ = try? Data(contentsOf: url) {
// The receipt exists. Do something.
}
else {
// Validation fails. The receipt does not exist.
exit(173)
}
}
Test your application in the sandbox environment.
iOS and tvOS developers must complete the following steps:
Sign out of the Store in the Settings application on your testing device.
Set the run destination of your application to an iOS or tvOS Device in Xcode.
Build and run your application from Xcode.
macOS developers must complete the following steps:
Build your application in Xcode.
Run your application.
You must launch your application from the Finder rather than from Xcode the first time in order to obtain a receipt. Click on your application in the Finder to launch it. macOS displays a "Sign in to download from the App Store." dialog. Enter your test user account and password as requested. The sandbox provides you with a new receipt upon successful authentication.
StoreKit connects to the sandbox environment when you launch your application from Xcode, from your test device (iOS and tvOS), or from the Finder (macOS). It connects to a production environment for applications that were downloaded from the App Store. You must not use your test user account to sign into the production environment. This will result in your test user account becoming invalid. Invalid test accounts cannot be used to test in-app purchase again.
Submit your In-App Purchase products for review.
Log in to iTunes Connect to submit your in-app purchase products for review by Apple, after you are done thoroughly testing them in the sandbox environment.
Update Your App for Ask to Buy
iOS 8 introduces Ask to Buy, which lets parents approve any purchases initiated by children, including apps or in-app purchases on the App Store. When a child requests to make a purchase, Ask to Buy will indicate that the app is awaiting the parent’s approval for this purchase by sending the Deferred
state to the paymentQueue(_:updatedTransactions:)
method on your transaction queue observer as shown in Listing 2. You should update your UI to reflect this deferred state, and expect paymentQueue(_:updatedTransactions:)
to be called again with a new transaction state reflecting the parent’s decision or after the transaction times out. Avoid blocking your UI or gameplay while waiting for the transaction to be updated. Furthermore, be sure to follow the Add a transaction queue observer at application launch best practice.
Listing 2 Responding to transaction statuses
func paymentQueue(_ queue: SKPaymentQueue, updatedTransactions transactions: [SKPaymentTransaction]) { |
for transaction in transactions { |
switch transaction.transactionState { |
// Call the appropriate custom method for the transaction state. |
case .purchasing: break |
// Do not block your UI. Allow the user to continue using your app. |
case .deferred: deferredTransaction(transaction) |
case .purchased: completeTransaction(transaction) |
case .restored: restoreTransaction(transaction) |
case .failed: failedTransaction(transaction) |
} |
} |
} |
If the child makes multiple Ask to Buy requests, only the most recent request is presented to the parent. Each new request restarts the 24 hour clock for processing the purchase request.
References
Document Revision History
Date | Notes |
---|---|
2017-06-29 | Updated screenshots and urls. |
Updated screenshots and urls. | |
2017-06-28 | Updated screenshots and urls. |
Updated screenshots and urls. | |
2016-11-09 | Updated screenshots, listing code, and links. |
2016-08-10 | Editorial update. |
2015-06-24 | Editorial update. Moved the FAQ section to TN2413, In-App Purchase FAQ. |
2014-09-12 | Added the Update Your App for Ask to Buy section. Updated FAQ 9. |
2014-08-06 | Editorial update. |
2014-01-30 | Updated for iOS 7. |
2013-02-21 | Fixed typos and updated the FAQ section. |
2012-08-29 | Updated the FAQ section and fixed typos. |
2012-02-22 | Added screenshots. Updated the "What's Next?" and FAQ sections. |
2011-08-03 | Added information about In App Purchase in Mac OS X 10.7. Updated the FAQ section. |
2011-05-23 | Updated the "What's Next?" section. |
2011-05-05 | Updated the FAQ section. |
2010-10-20 | Removed the Enable your App ID for In App Purchase section. Updated the FAQ section. |
2010-03-03 | New document that describes how to set up and test in-app purchase in your iOS, macOS, and tvOS applications. |
Copyright © 2017 Apple Inc. All Rights Reserved. Terms of Use | Privacy Policy | Updated: 2017-06-29