Navigating a Scripting Dictionary
A scripting dictionary window in Script Editor contains three primary areas. See Figure 12-1.
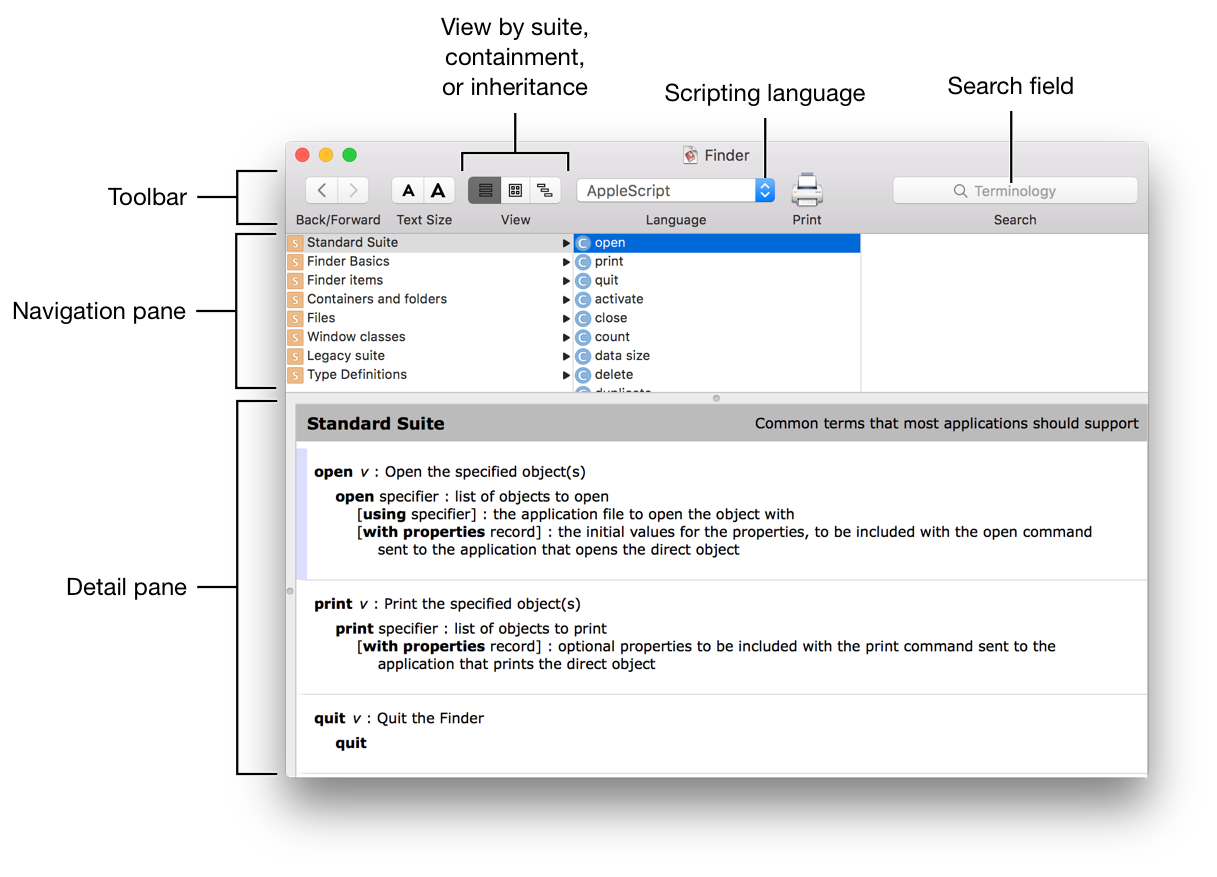
Toolbar. Options for toggling between terminology views, setting the scripting language, entering search terms, and more.
Navigation pane. Columns of scripting terminology.
Detail pane. Definitions for any terminology selected in the navigation pane.
Types of Terminology
The navigation pane of a dictionary includes the types of terms described in Table 12-1.
Type |
Icon |
Description |
---|---|---|
Suite |
|
A suite is a grouping of related commands and classes. Apps often have a Standard Suite, which includes terminology supported by most scriptable apps, such as an |
Command |
|
A command is an instruction that can be sent to an app or object in order to initiate some action. For example, |
Class |
|
A class is an object within an app, or an app itself. Mail, for example, has an |
Property |
|
A property is an attribute of a class. For example, the |
Key Concepts
It’s important to understand the concepts of inheritance and containment when navigating a scripting dictionary.
Inheritance
In a scriptable app, different classes often implement the same properties. For example, in Finder, the file
and folder
classes both have creation date
, modification date
, and name
properties. Rather than defining these same properties multiple times throughout the scripting dictionary, Finder implements a generic item
class. Since files and folders are considered types of Finder items, these classes inherit the properties of the item
class. In other words, any properties of the item
class also apply to the file
and folder
classes. There are many other items in Finder that also inherit these same properties, including the disk
, package
, and alias file
classes.
A class that inherits the properties of other classes can also implement its own properties. In Finder, the file
class implements a number of file-specific properties, including file type
and version
. The alias file
class implements an original item
property.
In some cases, a class inherits properties from multiple classes. In Finder, an alias is a type of file, which is a type of item. Therefore, the alias file
class inherits the properties of both the file
and item
classes, as shown in Figure 12-2.
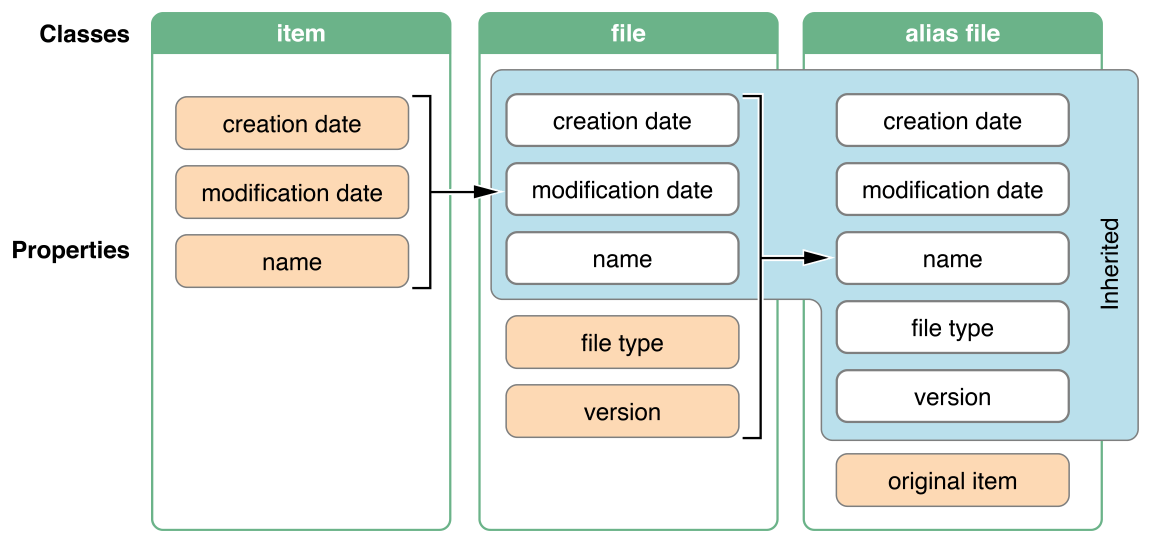
Containment
Classes of a scriptable app reside within a certain containment hierarchy. The application is at the top level, with other classes nested beneath. Finder, for example, contains disks, folders, files, and other objects. While files don’t contain elements, folders and disks can contain other folders and files. See Figure 12-3. Mail is another example—the application contains accounts, which can contain mailboxes, which can contain other mailboxes and messages.
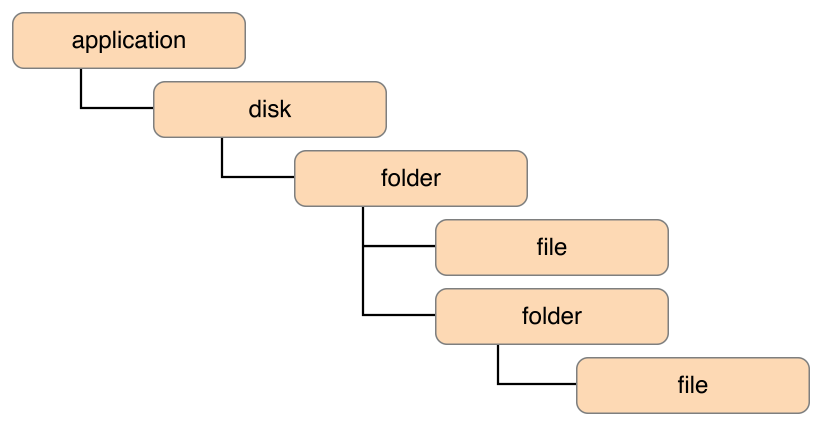
When referencing a class, you must do so very specifically according to its containment hierarchy in order to provide the scripting system with context. To reference a file in Finder, you would specify where the file resides in the folder hierarchy. See Listing 12-1 and Listing 12-2. To reference a message in Mail, you would specify where the message resides in the mailbox and account hierarchy.
APPLESCRIPT
tell application "Finder"
modification date of file "My File.txt" of folder "Documents" of folder "YourUserName" of folder "Users" of startup disk
end tell
--> Result: date "Monday, September 28, 2015 at 10:10:17 AM"
JAVASCRIPT
var Finder = Application("Finder")
Finder.startupDisk.folders["Users"].folders["YourUserName"].folders["Documents"].files["My File.txt"].modificationDate()
// Result: Mon Sep 28 2015 17:10:17 GMT-0700 (PDT)
Anatomy of a Command Definition
The definition of a command in a scripting dictionary is a recipe for using the command, as shown in Figure 12-4, Listing 12-3, and Listing 12-4.
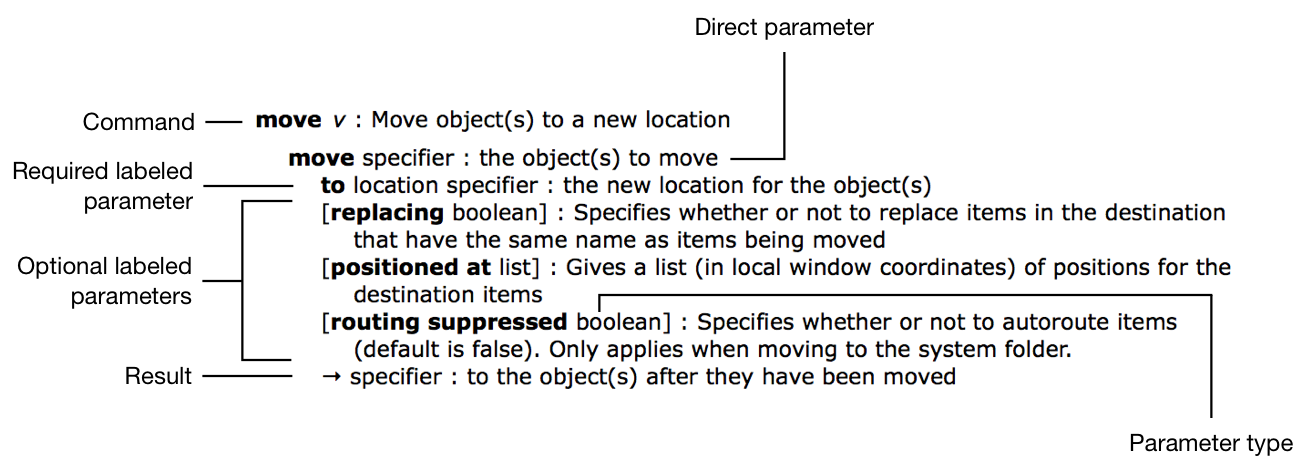
A command definition includes the following elements:
Command name. The name of the command.
Direct parameter. An object to be targeted by the command. For the
move
command in Finder, this is the object to be moved. If a command definition doesn’t specify a direct parameter, then the target object is the application. A direct parameter immediately follows the command.Labeled parameters. Control some aspect of the command’s behavior and are required or optional. Optional parameters are surrounded by brackets. Since these parameters are identified by label, they can be placed in any order when you write your script. The command definition denotes the value type for each labeled parameter. For example, the optional
replacing
parameter for themove
command in Finder takes a boolean value.Result. The result of the command, if any. Often, this is a reference to a newly created or modified object. For the
move
command in Finder, it’s a reference to the moved object.
APPLESCRIPT
tell application "Finder"
move folder someFolder to someOtherFolder replacing true
end tell
JAVASCRIPT
var Finder = Application("Finder")
Finder.move(someFolder, {
to: someOtherFolder,
replacing: true
})
Anatomy of a Class Definition
A class definition describes a class, as shown in Figure 12-5.
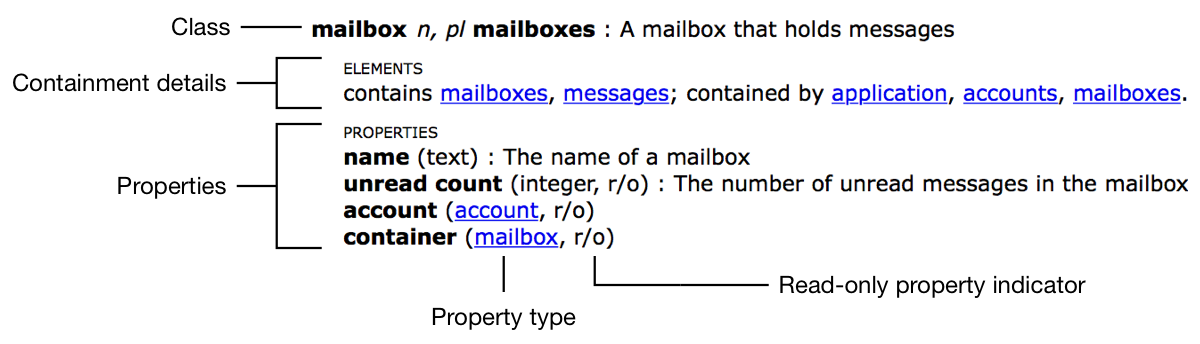
A class definition includes the following elements:
Class name. The name of the class.
Inheritance details. A list of other classes from which properties are inherited, if any. Each class is a hyperlink—clicking it takes you to the definition for the corresponding class.
Containment details. A list of contained classes, if any. May also list other classes containing the class, if any.
Properties. Any properties for the class, along with their data types. Read-only properties include an
r/o
indicator.
To view inherited properties, as well as containing classes in the Script Editor dictionary viewer, select Show inherited items in Preferences > General. See Figure 12-6.
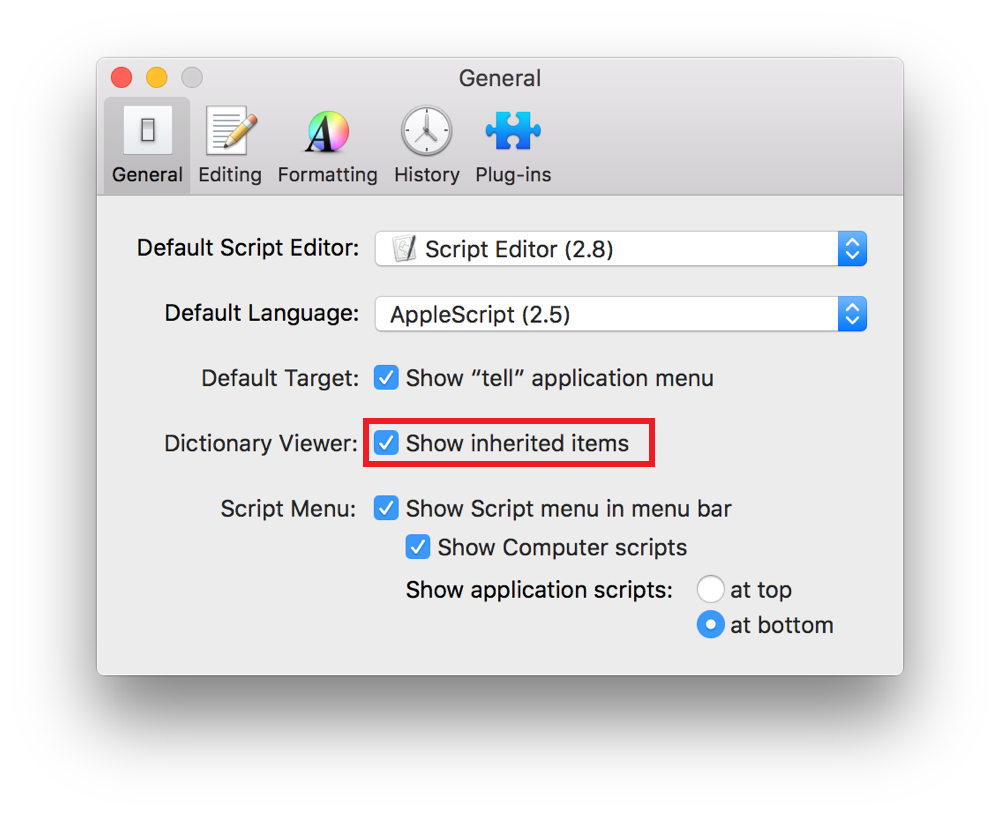
Opening a Scripting Dictionary
Copyright © 2018 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2016-06-13