Using Handlers/Functions
Collections of script statements that can be invoked by name are referred to as handlers in AppleScript, functions or methods in JavaScript, and subroutines in some other languages. Throughout this document, these terms are used interchangeably.
Handlers are generally written to perform a task multiple times throughout a script, such as displaying an alert, writing text to a file, or creating an email message. Instead of inserting the same code over and over, you write it once and give it a name. You can name a handler whatever you like as long as the name contains no special characters, such as punctuation, or spaces, and isn’t a reserved language term. You then call
, or evoke, a handler whenever necessary by referring to it by name. Each time you do, any code in the handler runs. Handlers can optionally be written to receive information as input for processing (parameters), and can return information as output (result or return value).
Handlers provide a way to organize your code by breaking it up into smaller, manageable, modular chunks. This can be useful when troubleshooting; you can narrow in on a single handler to resolve a problem, rather than sorting through a long, complex script. It also makes future script updates easier, as you can change behavior in one place to affect an entire script.
AppleScript Handlers
In AppleScript, a handler begins with the word on
or to
, followed by the handler name and its parameters, if any. It ends with the word end
, followed by the handler name. AppleScript handlers can be written with positional, labeled, or interleaved parameters.
Listing 13-1 shows a simple one-line script that displays a hypothetical error message, which you might want to display numerous times as a script runs.
APPLESCRIPT
display dialog "The script encountered a problem."
In Listing 13-1, the code from Listing 13-1 has been converted to a handler named displayError
, which has no parameters.
APPLESCRIPT
on displayError()
display dialog "The script encountered a problem."
end displayError
Listing 13-3 shows a variation of the handler in Listing 13-1, which uses the to
prefix instead of on
. Either syntax is acceptable.
APPLESCRIPT
to displayError()
display dialog "The script encountered a problem."
end displayError
You can now call the displayError
handler any time you want to display an error, as shown in Listing 13-4.
APPLESCRIPT
try
-- Do something
on error
-- Notify the user that there's a problem
displayError()
end try
try
-- Do something else
on error
-- Notify the user that there's a problem
displayError()
end try
For detailed information about AppleScript handlers, see About Handlers and Handler Reference in AppleScript Language Guide.
AppleScript Handlers with Positional Parameters
Positional parameters are a series of comma-separated variables, contained within parentheses, following the handler name. In Listing 13-6, the displayError
handler from Listing 13-1 has been updated to accept two positional parameters—an error message and a list of buttons to display.
APPLESCRIPT
on displayError(theErrorMessage, theButtons)
display dialog theErrorMessage buttons theButtons
end displayError
To call the handler, refer to it by name and provide a value for each positional parameter, as shown in Listing 13-7. The order of these values should match the parameter positions in the handler definition.
APPLESCRIPT
displayError("There's not enough available space. Would you like to continue?", {"Don't Continue", "Continue"})
For additional information about this style of handler, see Handlers with Positional Parameters in AppleScript Language Guide.
AppleScript Handlers with Interleaved Parameters
Interleaved parameters are a variation of positional parameters, in which the parameter name is split into pieces and interleaved with parameters using colons and spaces. Listing 13-8 shows how the handler from Listing 13-6 can be represented using interleaved parameters.
APPLESCRIPT
tell me to displayError:"There's not enough available space. Would you like to continue?" withButtons:{"Don't Continue", "Continue"}
on displayError:theErrorMessage withButtons:theButtons
display dialog theErrorMessage buttons theButtons
end displayError:withButtons:
Interleaved parameters resemble Objective-C syntax. Therefore, they are typically used to call Objective-C methods in AppleScriptObjC scripts.
Objective-C to AppleScript Quick Translation Guide discusses interleaved parameter use in AppleScriptObjC scripts. For additional information about this style of handler, see Handlers with Interleaved Parameters in AppleScript Language Guide.
AppleScript Handlers with Labeled Parameters
AppleScript also supports labeled parameters, although this style is rarely used when defining custom handlers. Most often, it’s a style used for event handlers. See Event Handlers. Listing 13-9 shows how the displayError
handler might appear if it were written using the labeled parameter style.
APPLESCRIPT
display of "There's not enough available space. Would you like to continue?" over {"Don't Continue", "Continue"}
to display of theErrorMessage over theButtons
display dialog theErrorMessage buttons theButtons
end display
For additional information about this style of handler, see Handlers with Labeled Parameters in AppleScript Language Guide.
JavaScript Functions
In JavaScript, a function name is preceded by the word function
and followed by a list of parameters, if any. The function’s contents are contained within curly braces ({ ... }
).
Listing 13-10 shows a simple script that displays a hypothetical error message.
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
function displayError() {
app.displayDialog("The script encountered a problem.")
}
You can now call the displayError
function any time you want to display an error, as shown in Listing 13-11.
JAVASCRIPT
try {
// Do something
} catch (error) {
// Notify the user that there's a problem
displayError()
}
try {
// Do something else
} catch (error) {
// Notify the user that there's a problem
displayError()
}
Using Parameters
JavaScript functions are written with positional parameters, comma-separated variables, contained within parentheses, following the function name. In Listing 13-12, the displayError
function from Listing 13-10 has been updated to accept two positional parameters—an error message and a list of buttons to display.
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
function displayError(errorMessage, buttons) {
app.displayDialog(errorMessage, {
buttons: buttons
})
}
To call the function, refer to it by name and provide a value for each positional parameter, as shown in Listing 13-13. The order of these values should match the parameter positions in the function definition.
JAVASCRIPT
displayError("There's not enough available space. Would you like to continue?", ["Don't Continue", "Continue"])
Exiting Handlers and Returning a Result
Often, handlers are used to process information and produce a result for further processing. To enable this functionality, add the return
command, followed by a value to provide, to the handler. In Listing 13-14 and Listing 13-15, the displayError
handler returns a Boolean value, indicating whether processing should continue after an error has occurred.
APPLESCRIPT
set shouldContinueProcessing to displayError("There's not enough available space. Would you like to continue?")
if shouldContinueProcessing = true then
-- Continue processing
else
-- Stop processing
end if
on displayError(theErrorMessage)
set theResponse to display dialog theErrorMessage buttons {"Don't Continue", "Continue"} default button "Continue"
set theButtonChoice to button returned of theResponse
if theButtonChoice = "Continue" then
return true
else
return false
end if
end displayError
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
function displayError(errorMessage) {
var response = app.displayDialog(errorMessage, {
buttons: ["Don't Continue", "Continue"],
defaultButton: "Continue"
})
var buttonChoice = response.buttonReturned
if (buttonChoice == "Continue")
return true
else
return false
}
var shouldContinueProcessing = displayError("There's not enough available space. Would you like to continue?")
if (shouldContinueProcessing) {
// Continue processing
} else {
// Stop processing
}
Event Handlers
Some apps, including scripts themselves, can call handlers when certain events occur, such as when launched or quit. In Mail, you can set up a rule to look for incoming emails matching certain criteria. When a matching email is detected, Mail can call a handler in a specified script to process the email. Handlers like these are considered event handlers or command handlers.
Listing 13-16 shows an example of a Mail rule event handler. It receives any detected messages as input, and can loop through them to process them.
APPLESCRIPT
using terms from application "Mail"
on perform mail action with messages theDetectedMessages for rule theRule
tell application "Mail"
set theMessageCount to count of theDetectedMessages
repeat with a from 1 to theMessageCount
set theCurrentMessage to item a of theDetectedMessages
-- Process the message
end repeat
end tell
end perform mail action with messages
end using terms from
Script Event Handlers
As previously mentioned, scripts can contain event handlers too. These handlers run when certain events occur.
Run Handlers
The run
event handler is called when a script runs. By default, any executable code at the top level of a script—that is, not contained within a handler or script
object—is considered to be contained within an implicit run
handler. See Listing 13-17 and Listing 13-18.
APPLESCRIPT
run
handler
display dialog "The script is running."
JAVASCRIPT
run
function
var app = Application.currentApplication()
app.includeStandardAdditions = true
app.displayDialog("The script is running.")
Optionally, the run
handler can be explicitly defined. Listing 13-19 and Listing 13-20 produce the exact same behavior as Listing 13-17 and Listing 13-18.
APPLESCRIPT
run
handler
on run
display dialog "The script is running."
end run
JAVASCRIPT
run
function
function run() {
var app = Application.currentApplication()
app.includeStandardAdditions = true
app.displayDialog("The script is running.")
}
Quit Handlers
The quit
event handler is optional, and is called when a script app quits. Use this as an opportunity to perform cleanup tasks, if necessary, such as removing temporary folders or logging progress. Listing 13-21 and Listing 13-22 demonstrate the use of a quit
handler.
APPLESCRIPT
quit
handler
on quit
display dialog "The script is quitting."
end quit
JAVASCRIPT
quit
function
var app = Application.currentApplication()
app.includeStandardAdditions = true
function quit() {
app.displayDialog("The script is quitting.")
}
Open Handlers
The inclusion of an open
handler or openDocuments
method in a script app automatically makes the app drag-and-droppable. When launched in this way, the open
handler receives a dropped list of files or folders as a direct parameter, as shown in Listing 13-23 and Listing 13-24.
APPLESCRIPT
open
handler
on open theDroppedItems
-- Process the dropped items here
end open
JAVASCRIPT
openDocuments
function
function openDocuments(droppedItems) {
// Process the dropped items here
}
For detailed information about using the open
handler to create drop scripts, see Processing Dropped Files and Folders.
Idle Handlers
When saving a script, you can optionally save it as a stay-open application. See Figure 13-1. In a stay-open script app, the script stays open after the run
handler completes, and an idle
handler is called every 30 seconds. Use the idle
handler to perform periodic processing tasks, such as checking a watched folder for new files to process. To change the duration between idle
calls, return a new duration, in seconds, as the result of the idle
handler. Listing 13-25 and Listing 13-26 demonstrate an idle
handler that delays for five seconds between executions.
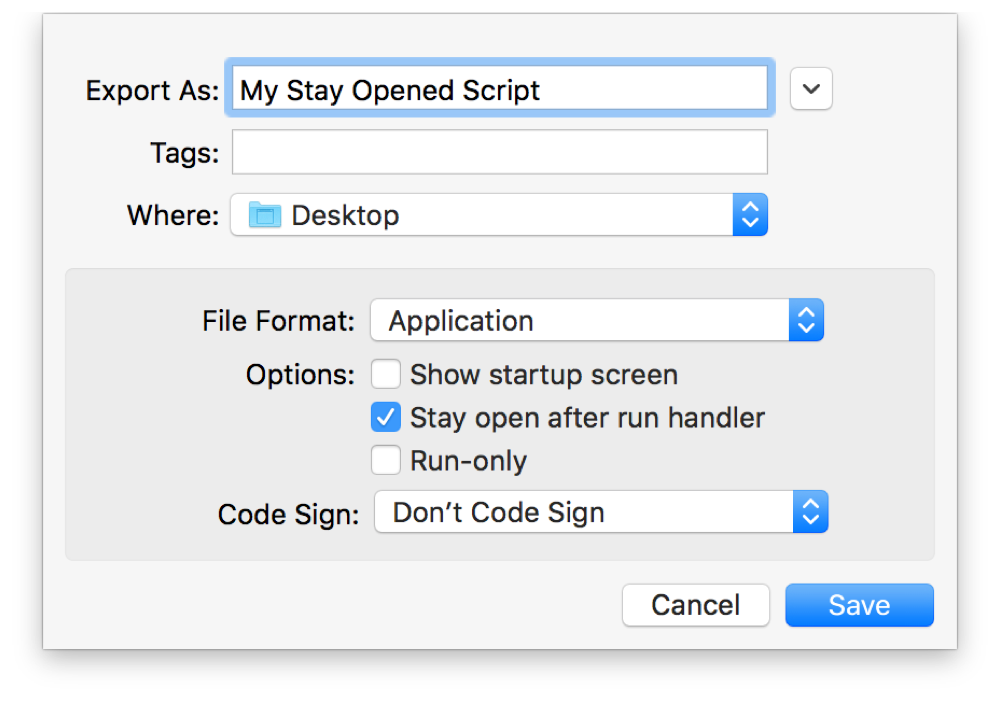
APPLESCRIPT
idle
handler
on idle
display dialog "The script is idling."
return 5
end idle
JAVASCRIPT
idle
function
var app = Application.currentApplication()
app.includeStandardAdditions = true
function idle() {
app.displayDialog("The script is idling.")
return 5
}
For information about using the idle
handler for folder watching, see Watching Folders.
Navigating a Scripting Dictionary
Copyright © 2018 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2016-06-13