Prompting for Files or Folders
It’s generally good practice to avoid hard-coding file and folder paths in a script. Prompting the user to select files and folders makes for a more dynamic script that won’t break when paths change.
Prompting for a File
Use the Standard Additions scripting addition’s choose file
command to prompt the user to select a file. Listing 26-1 and Listing 26-2 demonstrate how to use this command to display the simple file selection dialog with a custom prompt shown in Figure 26-1.
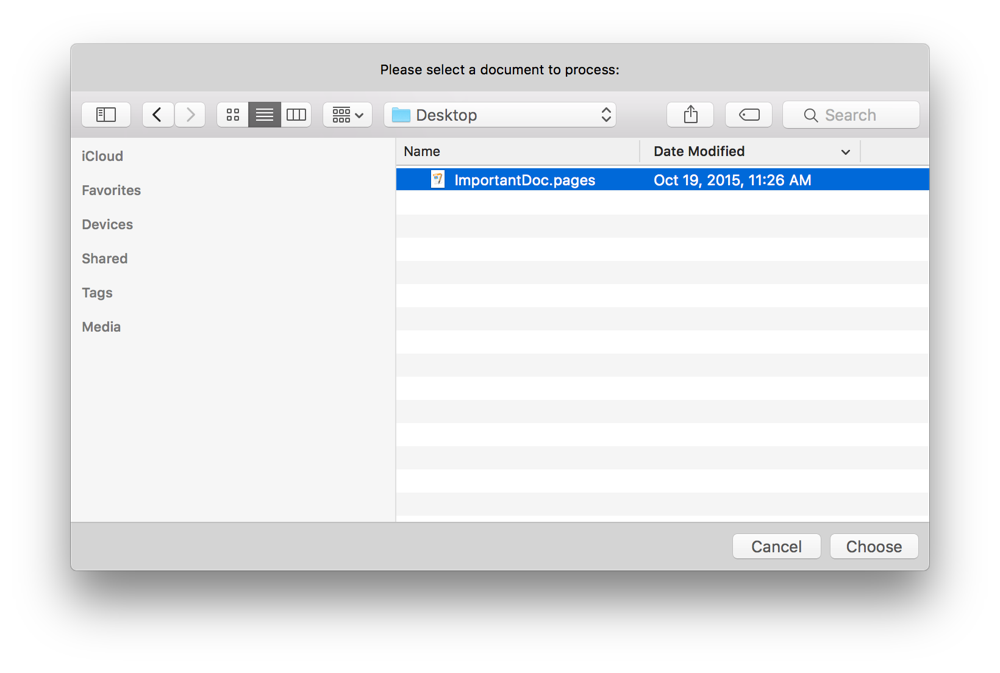
APPLESCRIPT
set theDocument to choose file with prompt "Please select a document to process:"
--> Result: alias "Macintosh HD:Users:yourUserName:Documents:ImportantDoc.pages"
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
var document = app.chooseFile({
withPrompt: "Please select a document to process:"
})
document
// Result: Path("/Users/yourUserName/Documents/ImportantDoc.pages")
Prompting for a Specific Type of File
If your script requires specific types of files for processing, you can use the choose file
command’s optional of type
parameter to provide a list of acceptable types. Types may be specified as extension strings without the leading period (such as "jpg"
or "png"
) or as uniform type identifiers (such as "public.image"
or "com.apple.iwork.pages.sffpages"
). Listing 26-3 and Listing 26-4 show how to prompt for an image.
APPLESCRIPT
set theImage to choose file with prompt "Please select an image to process:" of type {"public.image"}
--> Result: alias "Macintosh HD:Users:yourUserName:Pictures:IMG_0024.jpg"
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
var image = app.chooseFile({
withPrompt: "Please select an image to process:",
ofType: ["public.image"]
})
image
// Result: Path("/Users/yourUserName/Pictures/IMG_0024.jpg")
Prompting for Multiple Files
To let the user choose more than one file, include the choose file
command’s optional multiple selections allowed
parameter. Listing 26-5 and Listing 26-6 display a prompt asking for multiple images, as shown in Figure 26-2.
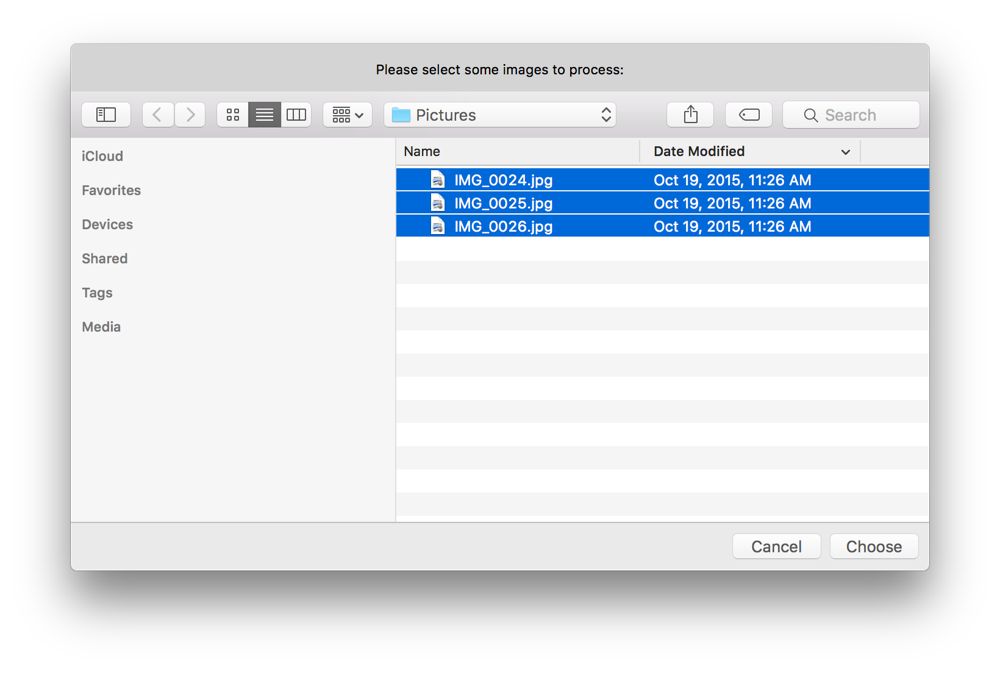
APPLESCRIPT
set theImages to choose file with prompt "Please select some images to process:" of type {"public.image"} with multiple selections allowed
--> Result: {alias "Macintosh HD:Users:yourUserName:Pictures:IMG_0024.jpg", alias "Macintosh HD:Users:yourUserName:Pictures:IMG_0025.jpg", alias "Macintosh HD:Users:yourUserName:Pictures:IMG_0026.jpg"}
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
var images = app.chooseFile({
withPrompt: "Please select some images to process:",
ofType: ["public.image"],
multipleSelectionsAllowed: true
})
images
// Result: [Path("/Users/yourUserName/Pictures/IMG_0024.jpg"), Path("/Users/yourUserName/Pictures/IMG_0025.jpg"), Path("/Users/yourUserName/Pictures/IMG_0026.jpg")]
Prompting for a Folder
Use the Standard Additions scripting addition’s choose folder
command to prompt the user to select a folder, such as an output folder or folder of images to process. Listing 26-7 and Listing 26-8 demonstrate how to use this command to display the simple folder selection dialog with a custom prompt shown in Figure 26-3.
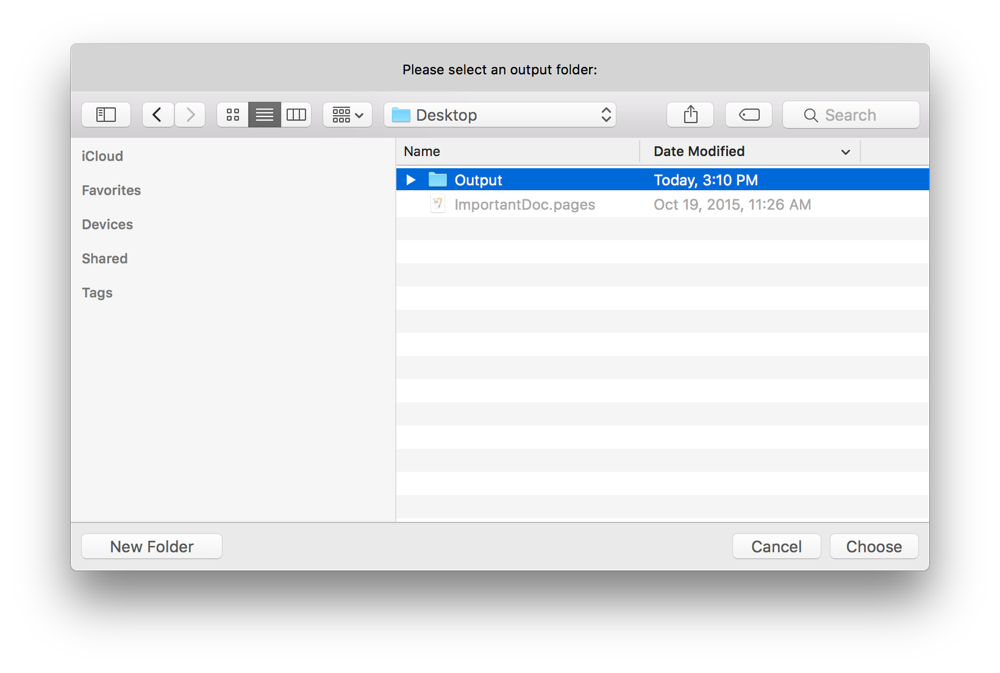
APPLESCRIPT
set theOutputFolder to choose folder with prompt "Please select an output folder:"
--> Result: alias "Macintosh HD:Users:yourUserName:Desktop:"
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
var outputFolder = app.chooseFolder({
withPrompt: "Please select an output folder:"
})
outputFolder
// Result: Path("/Users/yourUserName/Desktop")
Prompting for Multiple Folders
To let the user choose more than one folder, include the choose folder
command’s optional multiple selections allowed
parameter, as shown in Listing 26-9 and Listing 26-10.
APPLESCRIPT
set theFoldersToProcess to choose folder with prompt "Please select the folders containing images to process:" with multiple selections allowed
--> Result: {alias "Macintosh HD:Users:yourUserName:Desktop:", alias "Macintosh HD:Users:yourUserName:Documents:"}
JAVASCRIPT
var app = Application.currentApplication()
app.includeStandardAdditions = true
var foldersToProcess = app.chooseFolder({
withPrompt: "Please select an output folder:",
multipleSelectionsAllowed: true
})
foldersToProcess
// Result: [Path("/Users/yourUserName/Desktop"), Path("/Users/yourUserName/Documents")]
Copyright © 2018 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2016-06-13