Get started with Swift concurrency
October 10, 2022
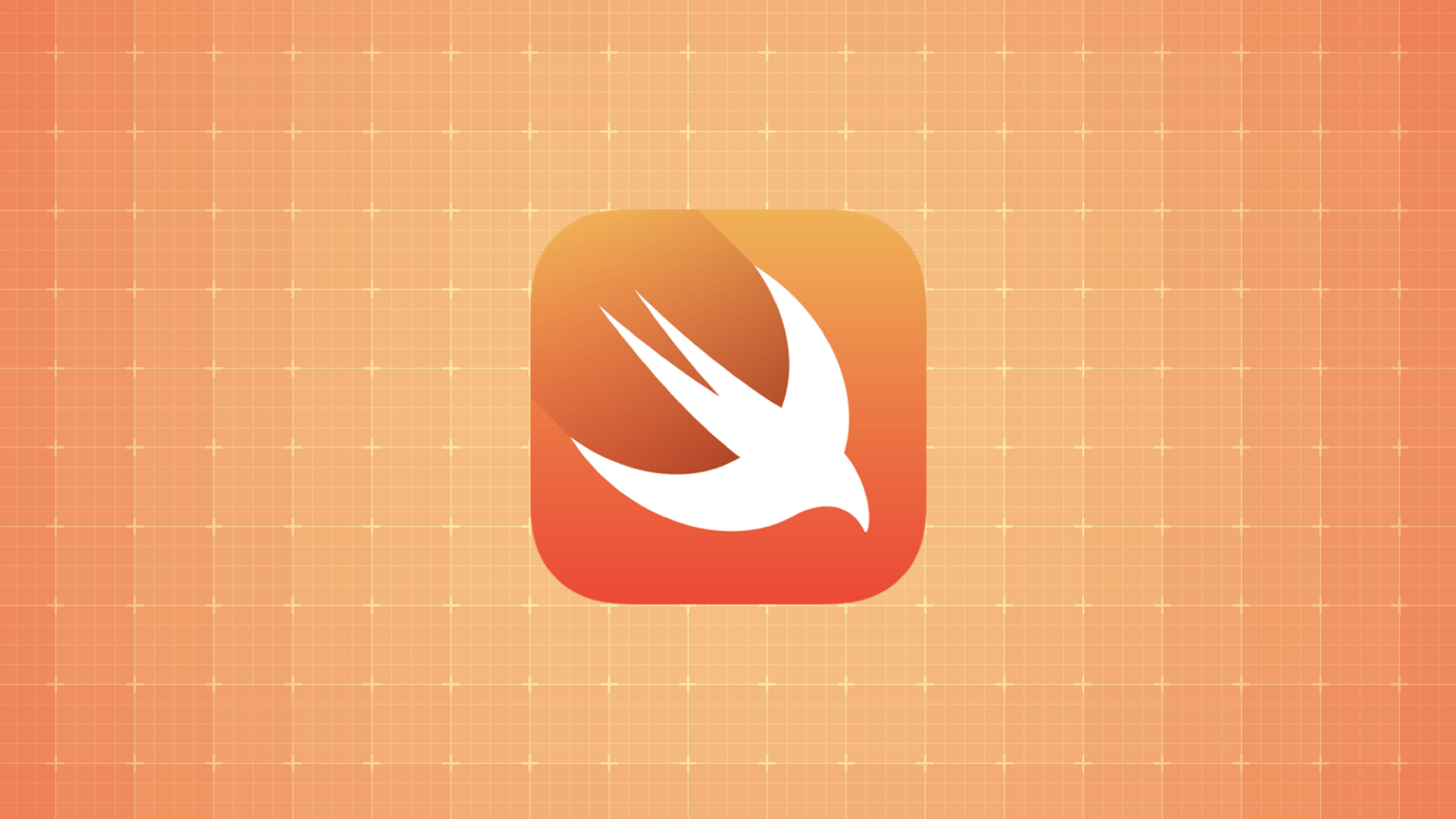
Swift offers built-in support for asynchronous and concurrent code to help you optimize parallelization of tasks — especially on machines with more cores. With Swift concurrency, you can ensure small tasks that require UI updates can be prioritized while longer tasks continue in the background. Discover how you can started with Swift concurrency, debug your tasks and actors, explore the latest tools, and much more. We’ll also show you how you can optimize the execution of asynchronous and parallel tasks when using Swift concurrency without interacting directly with threads.
Get started
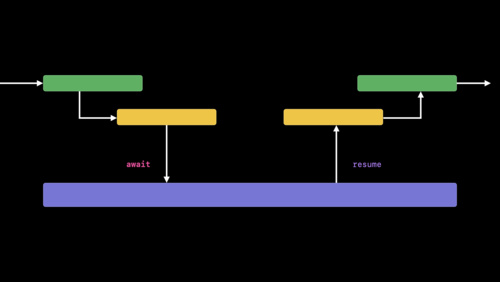
Meet async/await in Swift
Watch now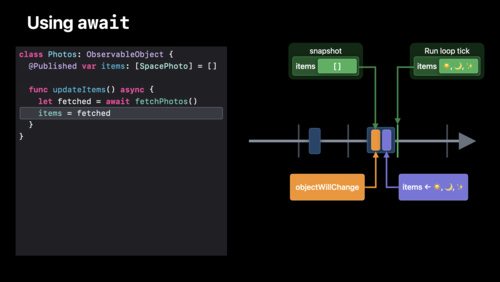
Discover concurrency in SwiftUI
Watch now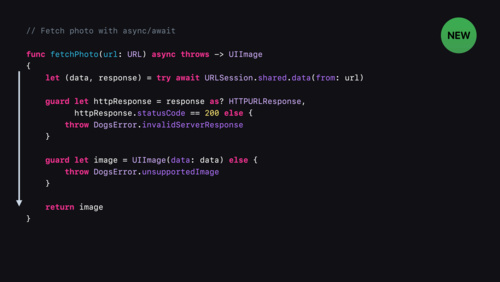
Use async/await with URLSession
Watch now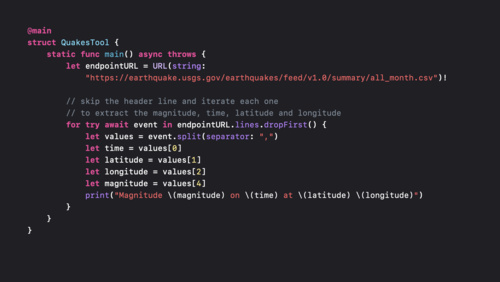
Meet AsyncSequence
Watch now
Meet distributed actors in Swift
Watch now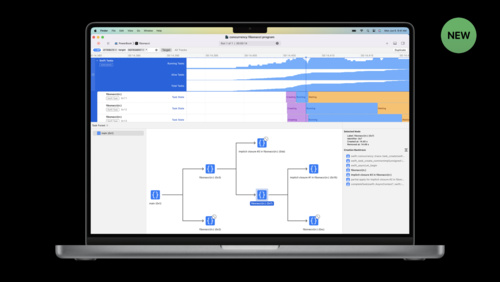
Visualize and optimize Swift concurrency
Watch now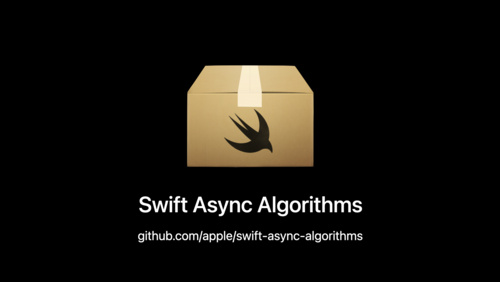
Meet Swift Async Algorithms
Watch nowThe Swift Programming Language: Concurrency
Best Practices

Swift concurrency: Update a sample app
Watch now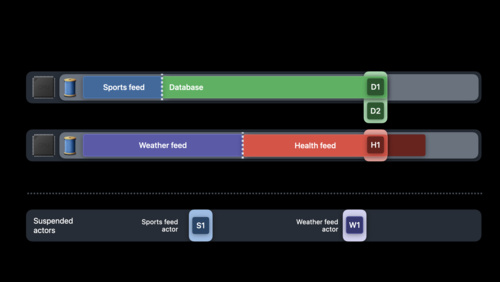
Swift concurrency: Behind the scenes
Watch now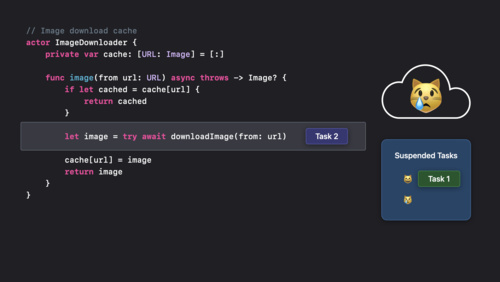
Protect mutable state with Swift actors
Watch now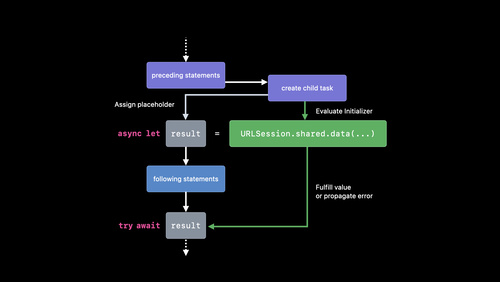
Explore structured concurrency in Swift
Watch now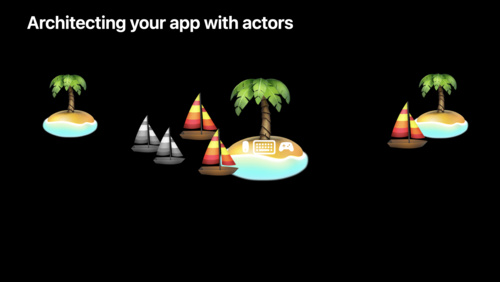