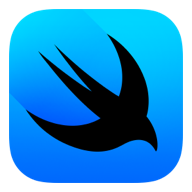
SwiftUI
SwiftUI helps you build great-looking apps across all Apple platforms with the power of Swift — and surprisingly little code. You can bring even better experiences to everyone, on any Apple device, using just one set of tools and APIs.
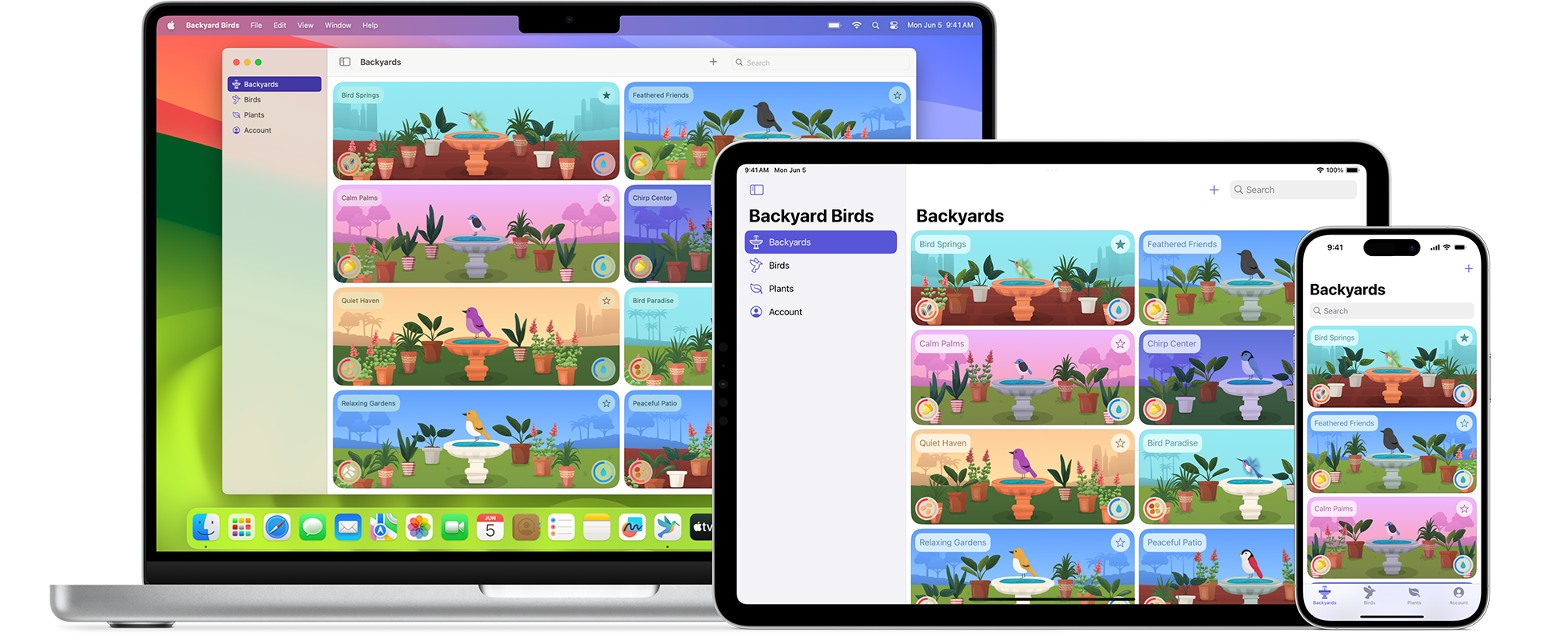
SwiftUI helps you build great-looking apps across all Apple platforms with the power of Swift — and surprisingly little code. You can bring even better experiences to everyone, on any Apple device, using just one set of tools and APIs.
SwiftUI is the best way to build apps across Apple platforms. Discover new capabilities to customize the look and feel of your apps, as well as improved interoperability with UIKit and AppKit when building animations and gestures. You can also take advantage of new text animations, plot functions in charts, take greater control of volumes and spaces in visionOS, and more.
SwiftUI uses a declarative syntax, so you can simply state what your user interface should do. For example, you can write that you want a list of items consisting of text fields, then describe alignment, font, and color for each field. Your code is simpler and easier to read than ever before, saving you time and maintenance.
import SwiftUI
struct AlbumDetail: View {
var album: Album
var body: some View {
List(album.songs) { song in
HStack {
Image(album.cover)
VStack(alignment: .leading) {
Text(song.title)
Text(song.artist.name)
.foregroundStyle(.secondary)
}
}
}
}
}
This declarative style even applies to complex concepts like animation. Easily add animation to almost any control and choose a collection of ready-to-use effects with only a few lines of code. At runtime, the system handles all of the steps needed to create a smooth movement, even dealing with user interaction and state changes mid-animation. With animation this easy, you’ll be looking for new ways to make your app come alive.
Xcode includes intuitive design tools that make it easy to build interfaces with SwiftUI. As you work in the design canvas, everything you edit is completely in sync with the code in the adjoining editor. Code is instantly visible as a preview as you type and you can even view your UI in multiple configurations, such as light and dark appearance.
Live updates. Xcode automatically builds, runs, and displays changes to your app as soon as you make them. The design canvas isn’t an approximation of your interface — it’s your app, running live.
Previews. You can create previews of any SwiftUI view to see how it looks with sample data, and configure almost anything your users might see, such as large fonts, localizations, or Dark Mode. Previews can also display your UI in any device and any orientation.
SwiftUI is designed to work alongside UIKit and AppKit, so you can adopt it incrementally in your existing apps. When it’s time to construct a new part of your user interface or rebuild an existing one, you can use SwiftUI while keeping the rest of your codebase.
And if you want to use an interface element that isn’t offered in SwiftUI, you can mix and match SwiftUI with UIKit and AppKit to take advantage of the best of all worlds.
Download Xcode and use these resources to build apps with SwiftUI for all Apple platforms.