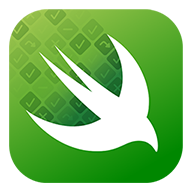
Swift Testing
Swift Testing is a new framework with expressive and intuitive APIs that make testing your Swift code a breeze.
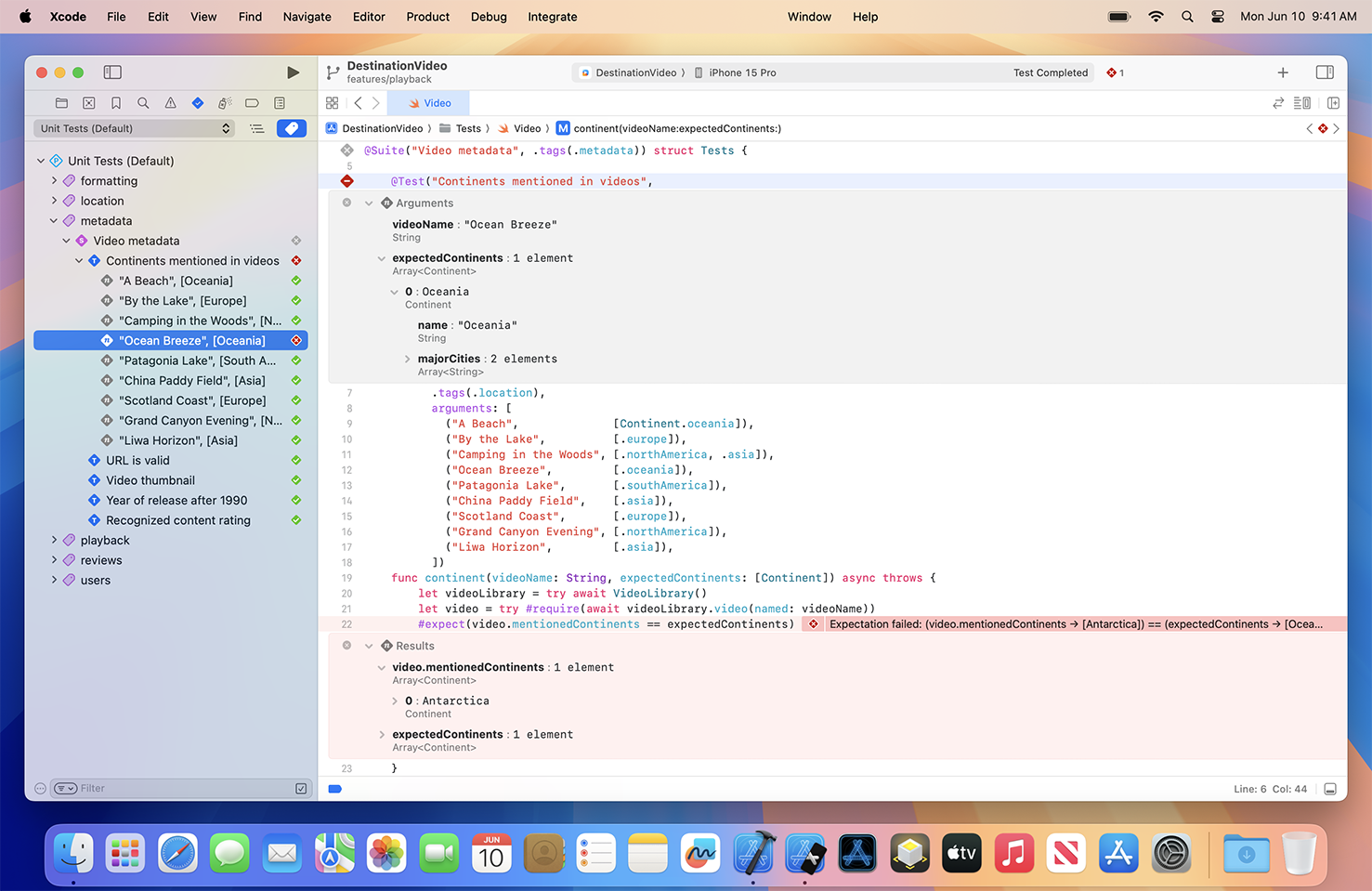
Swift Testing is a new framework with expressive and intuitive APIs that make testing your Swift code a breeze.
Swift Testing has a clear and expressive API built using macros, so you can declare complex behaviors with a small amount of code. The #expect
API uses Swift expressions and operators, and captures the evaluated values so you can quickly understand what went wrong when a test fails. Parameterized tests help you run the same test over a sequence of values so you can write less code. And all tests integrate seamlessly with Swift Concurrency and run in parallel by default.
@Test("Continents mentioned in videos", arguments: [
"A Beach",
"By the Lake",
"Camping in the Woods"
])
func mentionedContinents(videoName: String) async throws {
let videoLibrary = try await VideoLibrary()
let video = try #require(await videoLibrary.video(named: videoName))
#expect(video.mentionedContinents.count <= 3)
}
You can customize the behavior of tests or test suites using traits specified in your code. Traits can describe the runtime conditions for a test, like which device a test should run on, or limit a test to certain operating system versions. Traits can also help you use continuous integration effectively by specifying execution time limits for your tests.
@Test(.enabled(if: AppFeatures.isCommentingEnabled))
func videoCommenting() async throws {
let video = try #require(await videoLibrary.video(named: "A Beach"))
#expect(video.comments.contains("So picturesque!"))
}
Swift Testing provides many ways to keep your tests organized. Structure related tests using a hierarchy of groups and subgroups. Apply tags to flexibly manage, edit, and run tests with common characteristics across your test suite, like tests that target a specific device or use a specific module. You can also give tests a descriptive name so you know what they’re doing at a glance.
@Test("Check video metadata",
.tags(.metadata))
func videoMetadata() {
let video = Video(fileName: "By the Lake.mov")
let expectedMetadata = Metadata(duration: .seconds(90))
#expect(video.metadata == expectedMetadata)
}
You can work with your tests and tests suites in all Xcode workflows. Rich, inline presentations of test results help you see your test and detailed results side-by-side. You can also re-run specific arguments for parameterized tests to debug your code’s behavior. You can run tests in Xcode Cloud to take advantage of parallelization and receive rich summaries of the results. You can also run tests directly from the command line using the Swift Package Manager.
Swift Testing works on all major platforms supported by Swift, including Apple platforms, Linux, and Windows, so your tests can behave more consistently when moving between platforms. It’s developed as open source, with community input on upcoming features so the very best ideas, from anywhere, can help shape the future of testing in Swift.
If you already have tests written using XCTest, you can run them side-by-side with newer tests written using Swift Testing. This helps you migrate tests incrementally, at your own pace.
Download Xcode 16 beta to test your Swift code using the Swift Testing framework.