Hi @JoeKun,
Thanks for the response the other week. Do you know if the API team got anywhere with their investigation? I'm still seeing 500 errors when I hit the /me/library/artists
and /me/library/albums
endpoints.
I've also noticed a couple of other strange things which I think might be related...
Firstly, when I use the Apple Music web player (https://music.apple.com) I see An error occurred
when I try to view my Artists or Albums (although I'm still able to view my Songs and Playlists as expected). I've attached some screenshots to demonstrate:
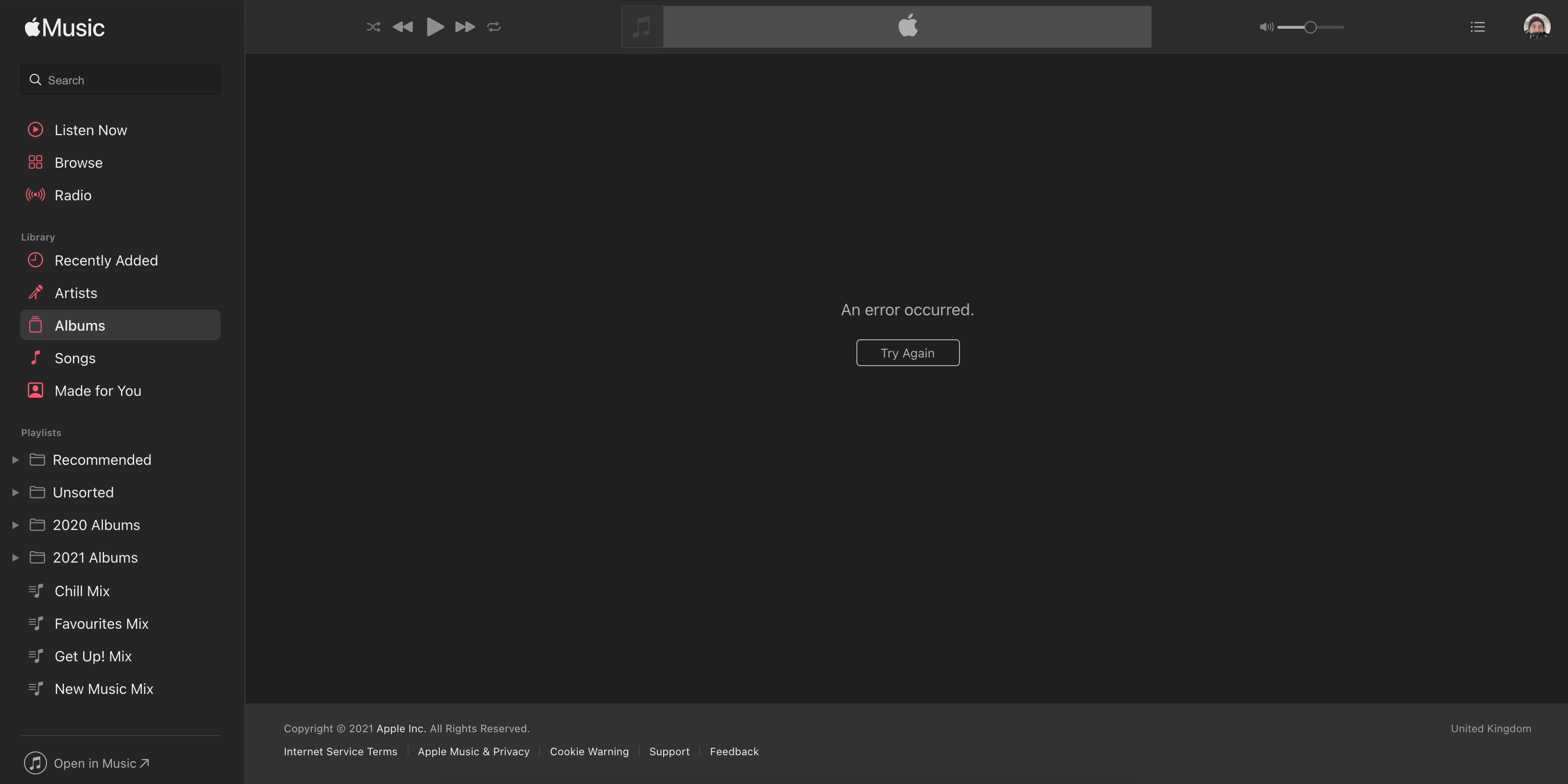
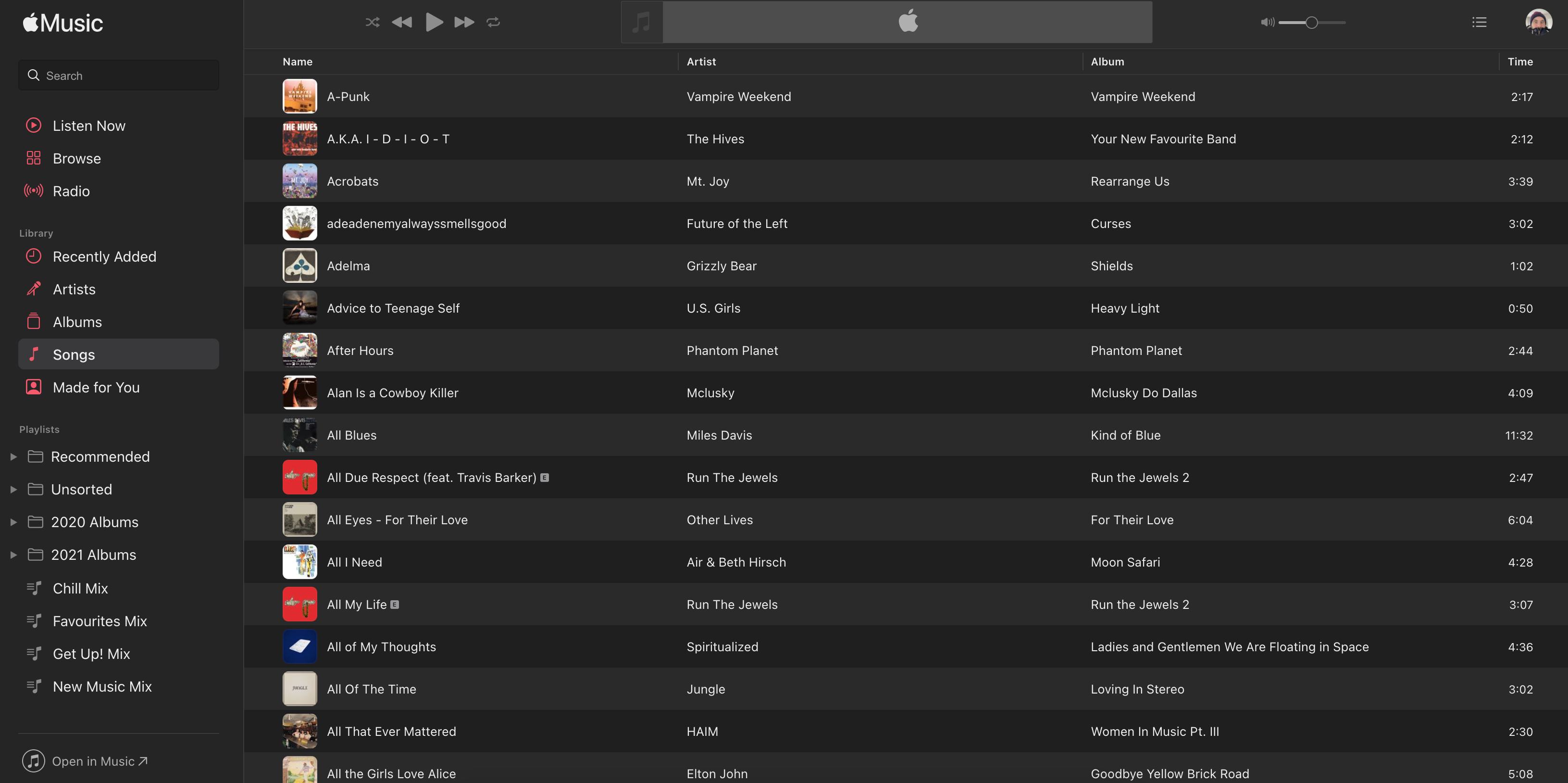
Secondly, when I fetch songs from the /me/library/songs
endpoint I'm seeing a discrepancy in the number of songs returned. In the response JSON I see "meta":{"total":1773}
, but when I page through 100 songs at a time I'm getting less than 100 songs per page, and I'm ending up with a total of just 1721 songs. I've included some console logs to demonstrate:
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=0, data.count: 98, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=100, data.count: 95, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=200, data.count: 99, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=300, data.count: 100, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=400, data.count: 96, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=500, data.count: 98, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=600, data.count: 94, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=700, data.count: 100, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=800, data.count: 96, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=900, data.count: 98, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1000, data.count: 98, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1100, data.count: 97, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1200, data.count: 96, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1300, data.count: 98, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1400, data.count: 97, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1500, data.count: 97, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1600, data.count: 97, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1700, data.count: 67, meta.total: 1773
fetchSongs url: https://api.music.apple.com/v1/me/library/songs?limit=100&offset=1800, data.count: 0, meta.total: 1773
fetchSongs fetched: 1721
I suspect that these issues might be related, I'm not sure if this is a general issue with the API or if it's specific to my account (I'm a solo dev and I haven't had the chance to test with another account yet). Any help or advice would be really appreciated!!