Here's the code.
I have a basic widget bundle
@main
struct TestWidgetBundle: WidgetBundle {
var body: some Widget {
TestCounterWidget()
}
}
And a simple timeline entry
struct TestEntry: TimelineEntry {
var date: Date
}
My timeline provider doesn't do much for now either.
struct TestTimelineProvider: TimelineProvider {
typealias Entry = TestEntry
func placeholder(in context: Context) -> TestEntry {
TestEntry(date: .now)
}
func getSnapshot(in context: Context, completion: @escaping (TestEntry) -> Void) {
let entry = TestEntry(date: .now)
completion(entry)
}
func getTimeline(in context: Context, completion: @escaping (Timeline<TestEntry>) -> Void) {
let calendar = Calendar.current
let currentDate = Date()
let entries = [TestEntry(date: .now)]
let timeline = Timeline(entries: entries, policy: .after(currentDate))
completion(timeline)
}
}
and finally the widget
struct TestCounterWidget: Widget {
let kind: String = "TestCounterWidget"
var body: some WidgetConfiguration {
StaticConfiguration(kind: kind, provider: TestTimelineProvider()) { entry in
if #available(watchOS 10.0, *) {
TestEntryView(entry: entry)
.containerBackground(.black, for: .widget)
} else {
TestEntryView(entry: entry)
.padding()
.background(.black)
}
}
.supportedFamilies([.accessoryRectangular])
.configurationDisplayName("Title")
.description("Description")
}
}
struct TestEntryView: View {
let entry: TestTimelineProvider.Entry
var body: some View {
HStack {
VStack {
Group {
Text("title")
.font(.system(size: 10, weight: .medium))
.foregroundStyle(.brandMain)
Text("subtitle")
.font(.system(size: 14, weight: .medium))
.widgetAccentable()
}
.frame(maxWidth: .infinity, alignment: .leading)
}
Image("placeholder")
.aspectRatio(1, contentMode: .fit)
.clipShape(RoundedRectangle(cornerRadius: 4))
.widgetAccentable() // commenting this line shows a gray box, instead of a pink one on tinted watch faces.
}
}
}
The image placeholder is in the bundle and it's only 5kb.
Here's the result:
A tinted watch face vs a non-tinted one.
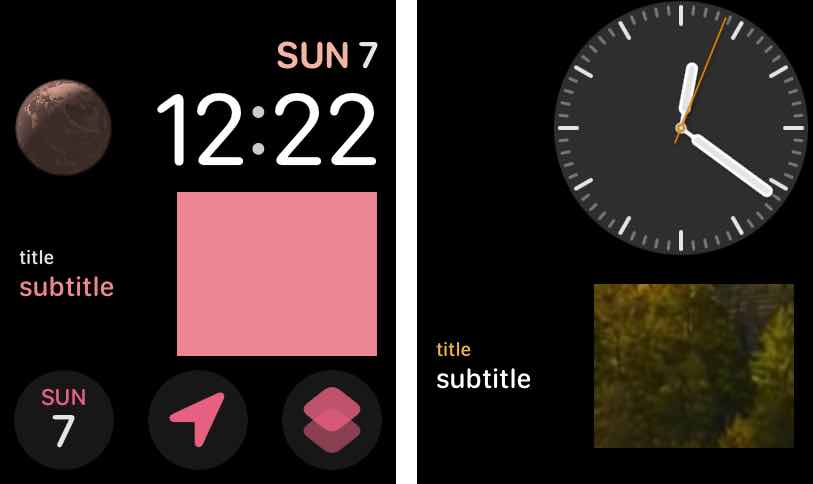