Planning your iOS app
If you’re new to iPhone software development, take a moment to familiarize yourself with the tools and technologies you’ll use. Apple provides everything you need to get started, and iOS technologies help you get the features and performance you want from your apps.
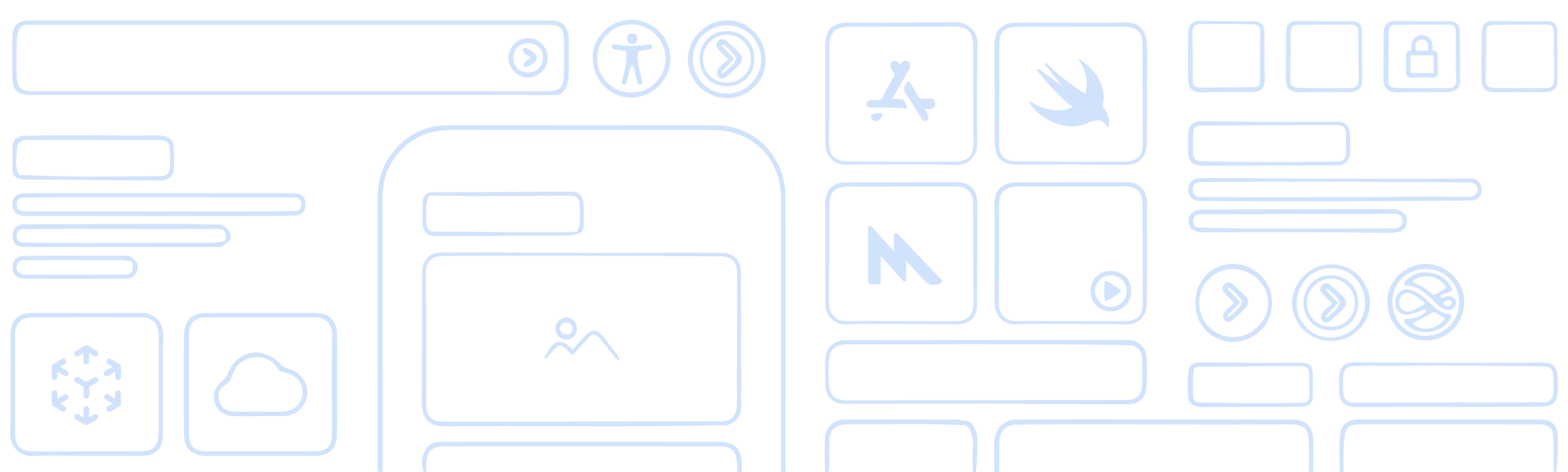
Explore the possibilities
When creating apps for iPhone, your initial development path affects many of the decisions you make later. Choose a path based on the type of content you’re offering, and how you want that content to look:
Assemble your UI from standard views
Build apps quickly from buttons, text labels, and other standard views and controls built into iOS. Customize the appearance of controls, or create entirely new views to present your content in unique ways. This approach works well for most apps and shortens development time.
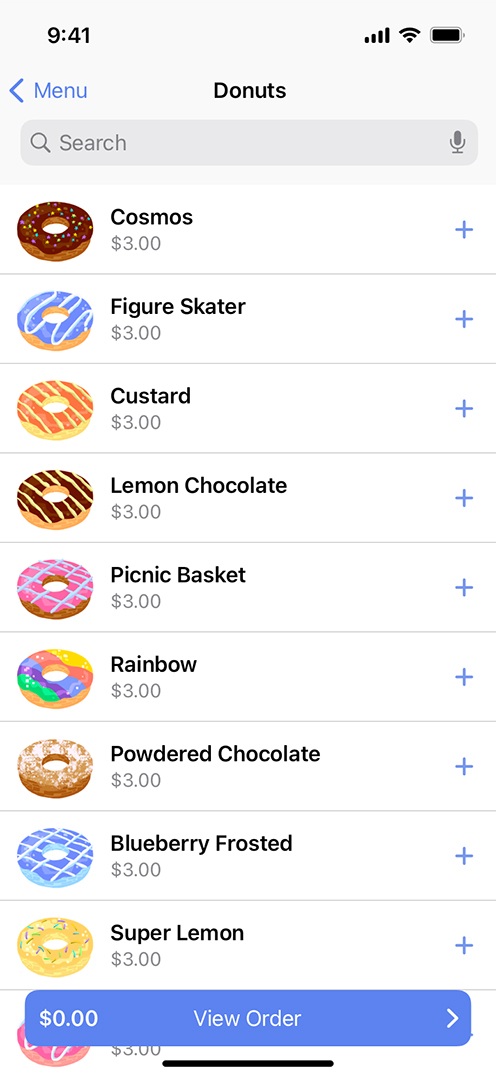
After you choose a path, think about the other technologies you might need. Apart from a few core technologies, you can adopt most technologies as necessary to support specific features. iOS technologies insulate your app from low-level hardware details and provide a stable base for building the rest of your app.
Where to start
Software development starts with Xcode — Apple’s integrated development environment. Xcode offers a complete set of tools for developing software, including project management support, code editors, visual editors for your UI, debugging tools, simulators for different devices, tools for assessing performance, and much more. Xcode also includes a complete set of system code modules — called frameworks — for developing your software.
Download Xcode from the Mac App Store
Xcode includes the SDKs for iOS, iPadOS, macOS, tvOS, and watchOS.
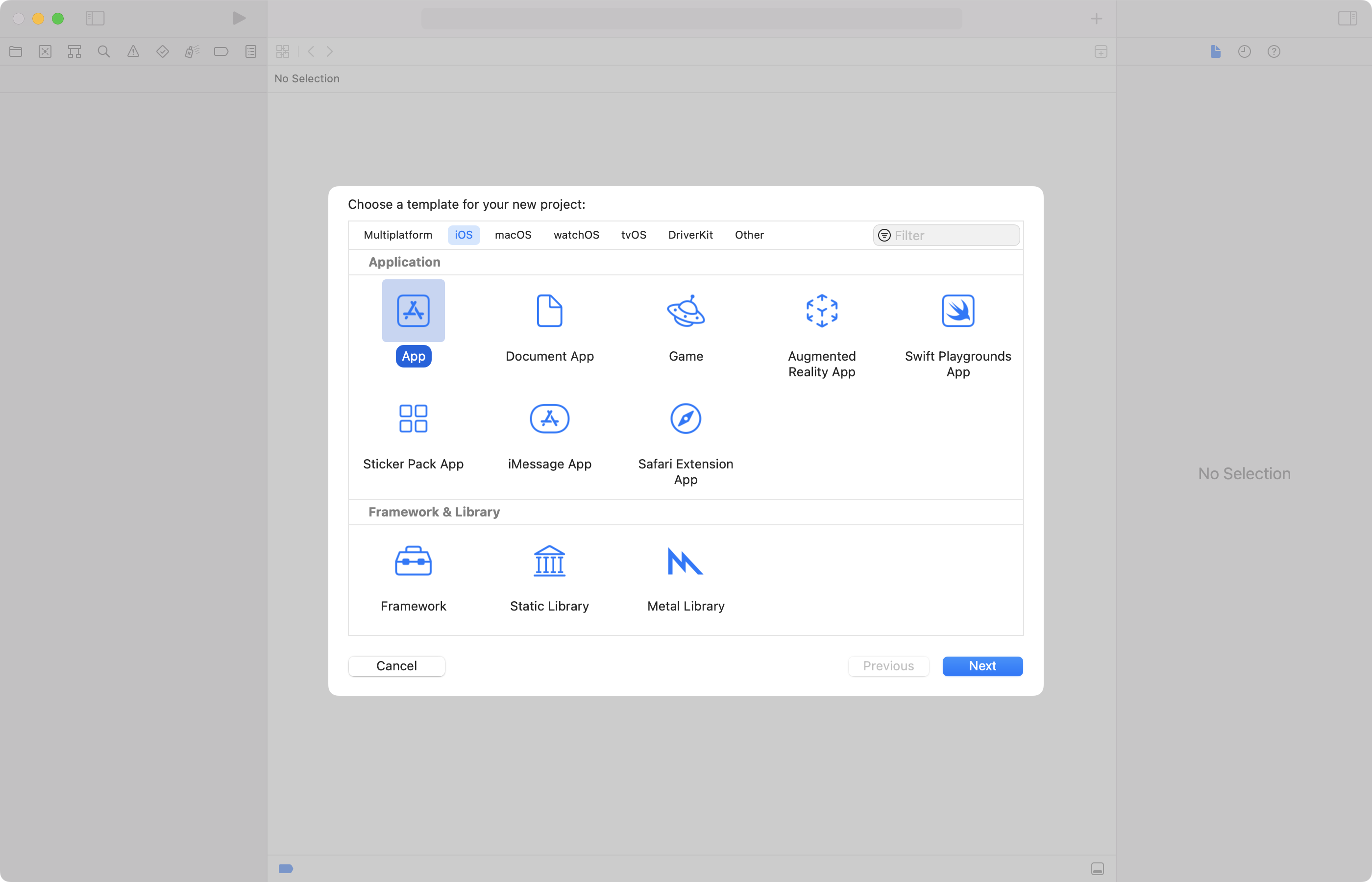
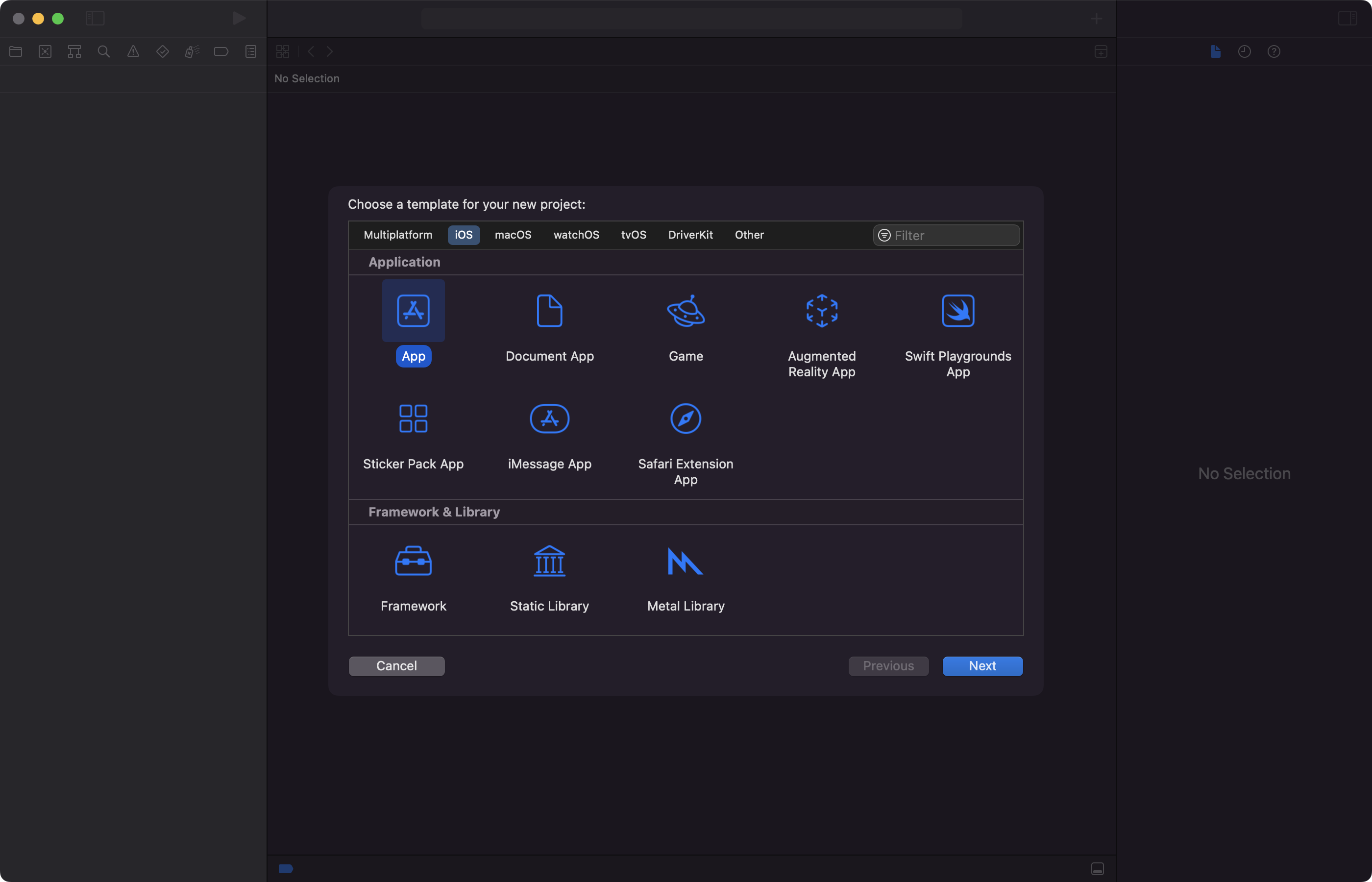
To create a new project in Xcode, choose File > New > Project and follow the prompts. Xcode gives you several options for your initial app type. The option you choose determines the initial configuration of your project, including what default code Xcode provides. The app type also defines the approach for creating your app’s UI. For example, select the Game option to draw your app’s content using one of several graphics technologies. For additional information about how to use Xcode, view Xcode documentation.
Adopt Swift
As you set up your project, consider which programming language to use. Swift is the preferred option because its syntax is concise, safe by design, and has modern features that make your code more expressive. Swift code also produces software that runs lightning-fast, and it’s interoperable with Objective-C so you can include source files for both languages in the same project.
Swift Programming Language Guide
The Swift Programming Language book gives a complete overview of the Swift programming language, and is the perfect place to begin your journey learning Swift.
Choose your app-builder technology
Another early choice to make is which app-builder technology to use for your interface. Apple’s app-builder technologies provide the core infrastructure iOS needs to communicate with your app. They also define the programming model you use to build your interface, handle events, and more.
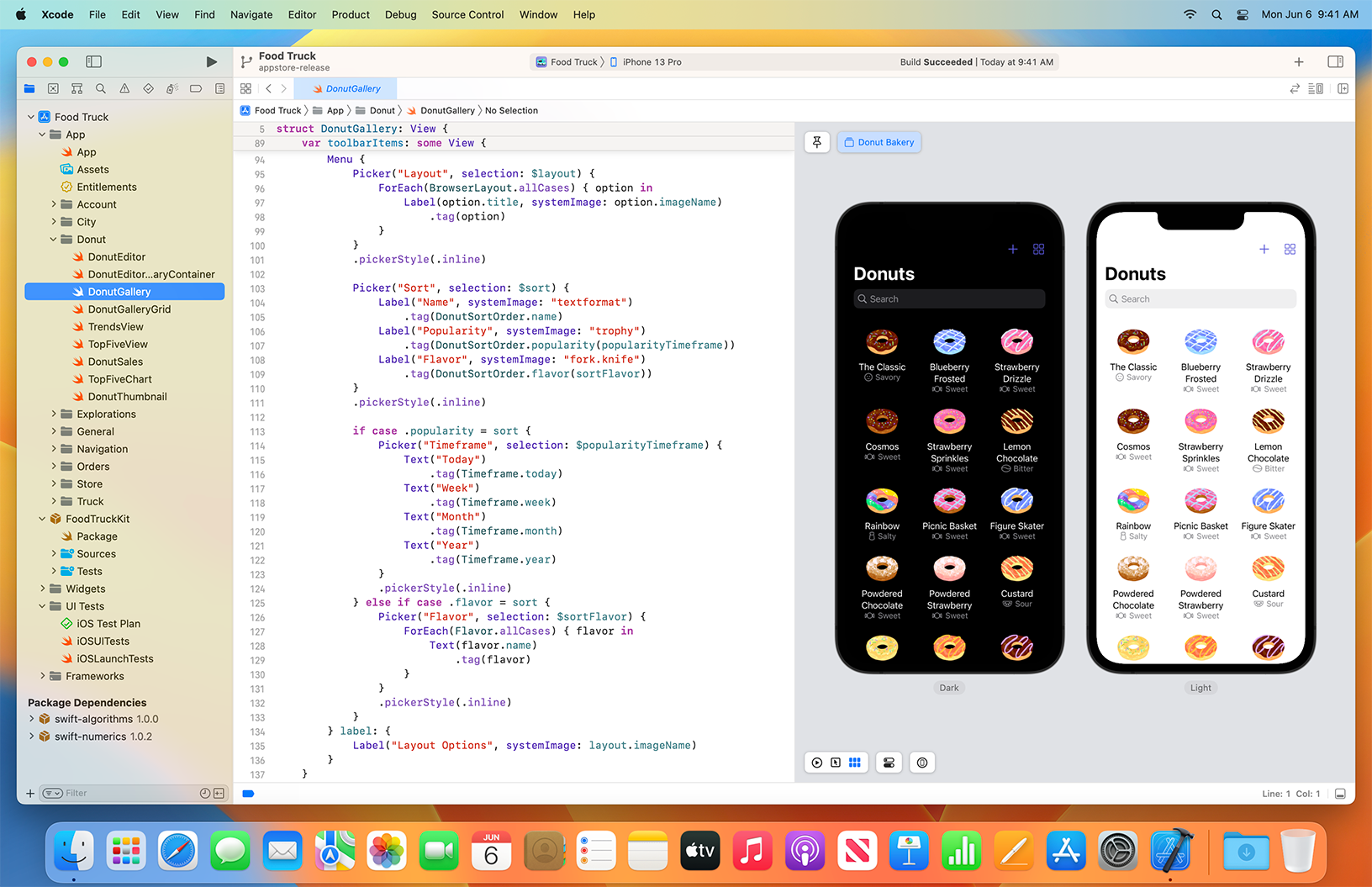
SwiftUI
SwiftUI is the preferred app-builder technology, because it offers a modern, platform-agnostic approach to building your UI and app infrastructure. With SwiftUI, you specify your interface programmatically and let the system display and update that interface dynamically, including inside the Xcode editor. For a guided walkthrough of using SwiftUI, view the Introducing SwiftUI tutorial.
UIKit
UIKit lets you build your interface programmatically in your code or visually using storyboards. UIKit offers a more traditional approach to building apps, giving you full control over the management of interface elements. Write the code you need to update views and controls, change their configuration, and communicate changes to other parts of your app. For a walkthrough of using UIKit to build apps, view the Getting Started with Today tutorial.
If you’re unsure where to start, choose SwiftUI as your app-builder technology. Both SwiftUI and UIKit provide everything you need to write apps, but SwiftUI offers a better starting point for development. In addition, SwiftUI and UIKit are interoperable, so choosing one technology doesn’t preclude you from using the other in the same app. You can easily mix SwiftUI views and UIKit views in the same view hierarchies.
SwiftUI and UIKit work seamlessly with Apple’s data management technologies to support the creation of your interfaces. Swift Standard Library and Foundation framework provide structural types such as arrays and dictionaries, and value types for strings, numbers, dates, and other common data values. For any custom types you define, adopt Swift’s Codable support to persist those types to disk. If your app manages larger amounts of structured data, Core Data and CloudKit offer object-oriented models for managing and persisting your data.
Design the user experience
An app that offers an engaging experience keeps people coming back. To create that experience, you need a UI that looks good, has an easy-to-understand layout, and emphasizes the right content. You also want interactions with your UI to be intuitive and match existing patterns. Most importantly, you want an interface that feels natural on iPhone.
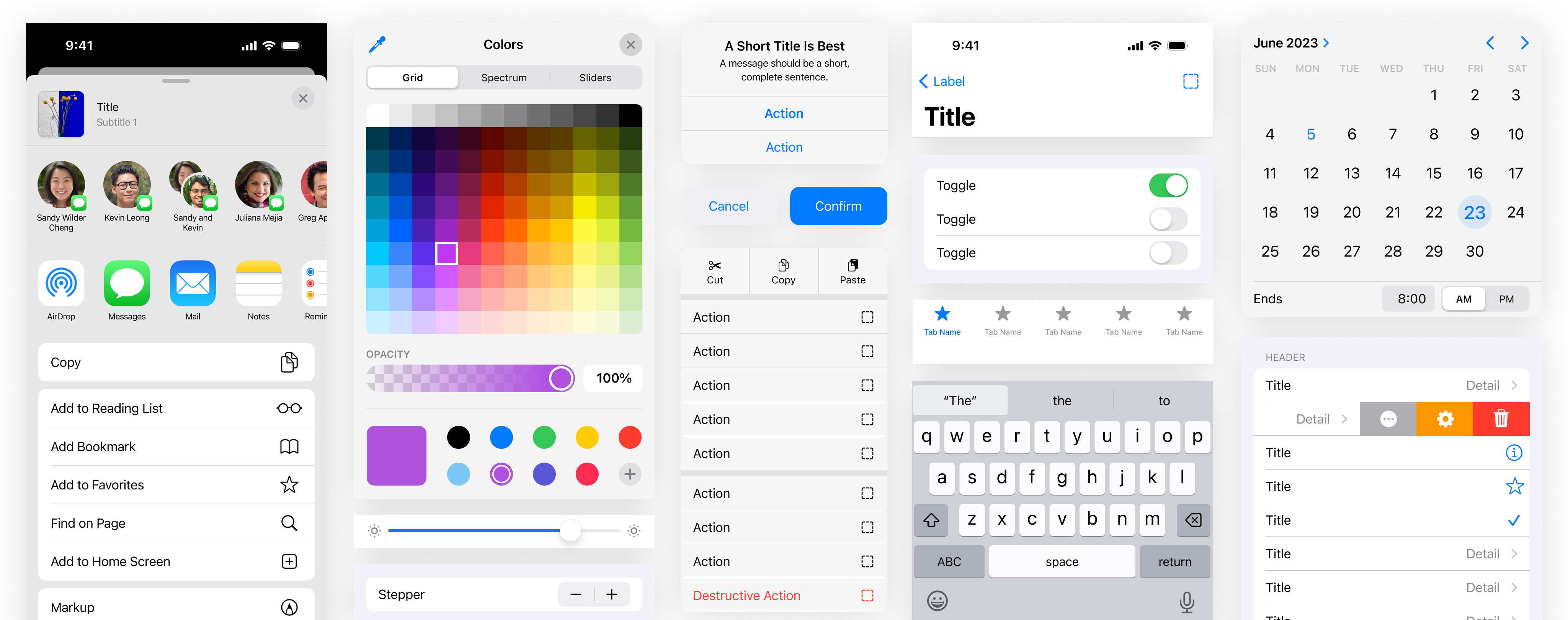
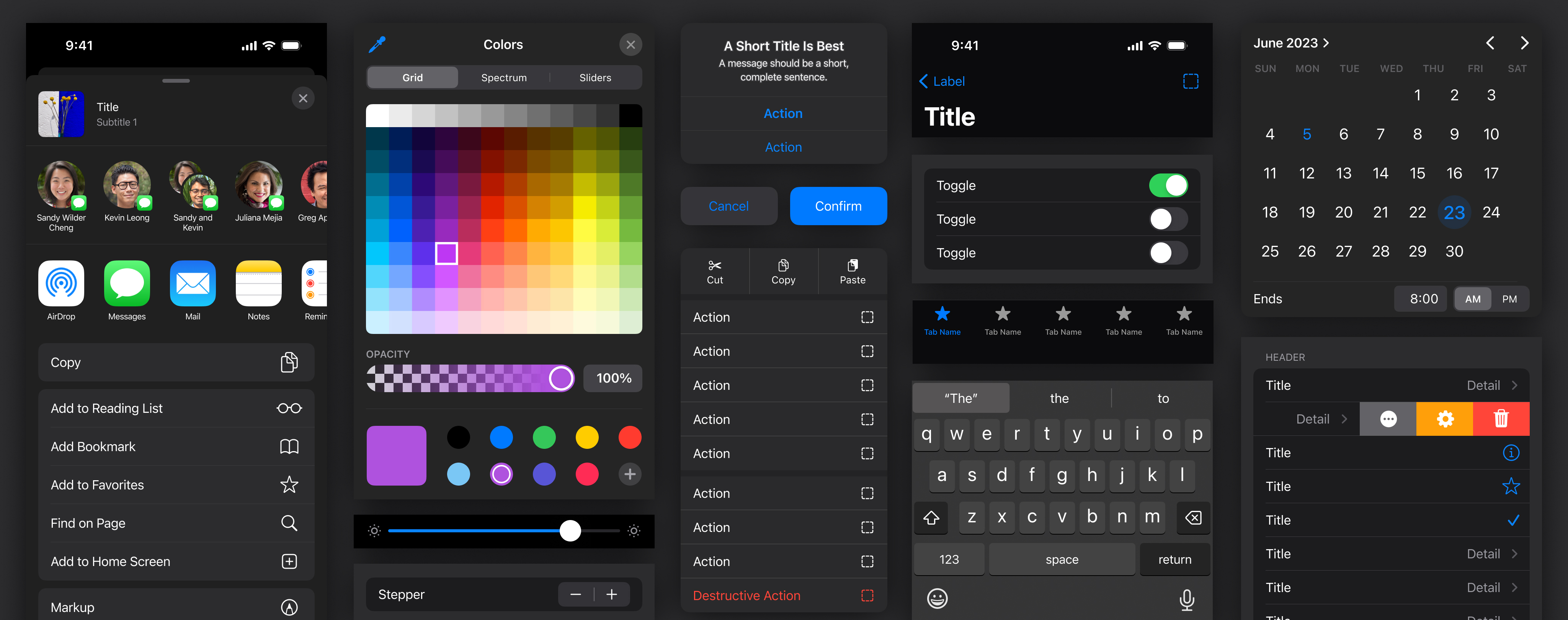
Human Interface Guidelines
Apple’s Human Interface Guidelines offer invaluable information on how to design your app’s interface, navigate content, and manage interactions on iPhone. Make reading these guidelines a priority in your explorations of the iOS ecosystem, and use the corresponding Apple Design Resources to dive into designing your app.
Gracefully handle different iPhone sizes, orientations, and appearance customizations. iPhone comes in many sizes, and people can hold it in a portrait or landscape orientation. They can also customize the appearance of apps and the system by changing some system settings. To support all of these customizations, make sure you adopt the following features:
Scenes
A scene is a program-level object that manages your app’s interface. Adopt scene objects as part of your app’s core infrastructure, and use them to adjust your app’s behavior at important times. iOS relies on scenes to run multiple instances of your interface simultaneously. For information about how to support scenes, view the SwiftUI and UIKit documentation.
Automatic layout
Rather than adjust the position of views manually to accommodate different screen sizes, orientations, and configurations, tell the system how to make those adjustments relative to the current safe areas. SwiftUI adopts automatic layout as part of its UI design approach. To adopt automatic layout in UIKit views, add Auto Layout constraints to your interface.
Appearance variations
Build your UI to support system-level appearance changes. Dark Mode lets people choose between a light or dark UI, and accessibility settings let people choose a high-contrast UI. Different appearances rely on different color palettes and image assets, which you manage with the help of asset catalogs. For other parts of your UI, use system APIs to determine when it’s time to change between light, dark, or high-contrast content. For information, view Supporting Dark Mode in your interface.
Dynamic Type
People can ask the system to use a bigger font to make text more readable or a smaller font to fit more information onscreen. Respect these font-size changes by adjusting your app’s text too. Adopt standard type styles to make these changes automatically, or use system APIs to manually update text that contains custom fonts. In SwiftUI, get the new text size from the view’s environment. In UIKit, get the new text size from trait collections.
Scalable images
Incorporate SF Symbols to make your app more adaptable to changes. The SF Symbols app offers a vast collection of configurable, vector-based images that adapt naturally to appearance and size changes. They also blend well with the San Francisco system font, resulting in a consistent look across Apple platforms. View Configuring and displaying symbol images in your UI.
You might not think an iOS app needs menus, but add them anyway. Adding menus to your iOS app gives people the option to control your app from a connected keyboard. And if you create a Mac version of your iOS app using Mac Catalyst, you’ll use those menus in the Mac version of your app. For more information, view the SwiftUI or UIKit documentation.
Make the experience of using your app’s interface continuous between launches. Each time someone launches your app, restore its previous state and appearance to let the person continue from their last stopping point. This continuity is essential because the system can terminate background apps to reclaim memory and resources. For more information, view Restoring your app’s state with SwiftUI (SwiftUI) or Preserving your app’s UI across launches (UIKit).
Adopt best practices during development
Offer the best possible experience for everyone by doing the following:
Protect people’s privacy
Privacy is important, so keep people informed about how you use their data. If you collect data, offer a privacy statement that explains how you use that information. When you use Apple technologies that operate on personal data, include usage descriptions for the system to display on first use. For more information, view Protecting the user’s privacy.
Secure the data you collect and store
If you do collect data, make sure you protect that data from malicious attacks. Adopt passkeys as a secure alternative to passwords. Store personally identifiable information, financial data, or other sensitive data in the user’s encrypted Keychain. Use on-disk encryption or other Apple security technologies to store other personal data. Use Apple CryptoKit to encrypt data that you store locally or send outside your app.
Audit your accessibility support
Apple builds accessibility support right into its technologies, but screen readers and other accessibility features rely on information your app provides. SwiftUI and UIKit can describe each piece of your UI, but only you know how those pieces work together. Review accessibility labels and other descriptions to make sure they provide helpful information, and make sure focus-based navigation is simple and intuitive. For more information, view Accessibility.
Internationalize and localize your app
Embrace a global market by localizing your app for other regions and languages. Prepare your app using the Foundation framework, which provides code to format strings, dates, times, currencies, and numbers for different languages and regions. Ensure your UI looks good for both left-to-right and right-to-left languages. Localize app resources and add them to your Xcode project. For information about the internationalization and localization process, view Localization.
Design for everyone
Consider social and cultural differences when developing content, and avoid images and terms that have negative or derogatory connotations for portions of your audience. For more information, view Inclusion.
Test and debug your app thoroughly
During the development cycle, debug problems as they arise using the built-in Xcode debugger. Build automated test suites using XCTest and run them during every build to validate new code works as expected. Use the continuous integration system of Xcode Cloud to automate builds, test cycles, and the distribution of your apps to your QA teams.
Optimize your app’s performance
Identify bottlenecks and other performance issues in your code using the Instruments app that comes with Xcode. Profile your running code, find memory leaks, analyze resource usage, and much more. For information about how to gather metrics using Instruments, view Improving your app’s performance.
Choose a business model for your app
Distribute your app worldwide using a variety of business models, including free, free with in-app purchases, pay-to-download, and more. Build your interface in a way that offers a cohesive experience and supports your chosen business model. For more information, view Choosing a business model.
Build for multiple platforms
If you start development on iOS, consider adding support for iPadOS at the same time. iOS and iPadOS share many of the same technologies, making it easy to support both with the same executable.
You can also support other platforms with the same code you write for iOS. You can reuse nearly all of your app’s structural and data-based code on any Apple platform. Apple technologies also make it easy to reuse your UI and other parts of your app:
Reuse SwiftUI views and UI on all Apple platforms
The universal availability of SwiftUI makes it an ideal choice for development, and reduces the time it takes to deliver custom versions of your apps on different platforms.
Create a Mac version of your iOS app quickly using Mac Catalyst
Rebuild your app using Mac Catalyst to get a version that runs on macOS. Spend the rest of your time tuning the experience of your app to fit more naturally onto the Mac platform. For more information, view Mac Catalyst.
Run your iOS app unmodified on Macs with Apple silicon
For information, view Running your iOS apps on macOS.
How to take it further
Once you have an app up and running, look for additional ways to improve the experience. Little things can make a big difference, whether it’s adding a particular feature, or presenting your content in a different way. For example:
Add Wallet and Apple Pay support
Make it easier for people to use your app’s services using Wallet and Apple Pay. If your app provides rewards cards, boarding passes, tickets, gift cards, or other types of digital cards, make them easier to access by adding them to someone’s Wallet. To make it easier for people to pay for goods and services, add Apple Pay support to your apps.
Deliver contextually appropriate content to people
Think about how people use your app, and find ways to surface important content quickly. Use location data or the current date and time to filter results, or to generate important notifications. Use machine learning to analyze data and offer better solutions. Rearrange your interface dynamically to make frequently used content more accessible.
Help people find your app’s content
Provide a comprehensive description of items to Spotlight search to make those items more findable. When someone selects a search result, use the provided user activity object to put your app in the proper state to display the result.
Consider other forms of input
People use touch input for most interactions, but iPhone also supports other types of input. SwiftUI interaction types and UIKit interaction objects handle complex event sequences for drag and drop, band selection, scribble input, and more. The Core Motion framework supports motion-based input using the device’s built-in accelerometers and gyroscopes. The AVFoundation framework supports audio and video capture using the built-in cameras and microphone. Games can adopt the Game Controller framework to support external game controller hardware.
Add runtime condition checks around new features
When you update your app to support a new version of iOS, place runtime condition checks around code for new features. Runtime checks eliminate the need to ship different versions of your app: one for people running the new version of iOS, and one for people running older versions. Everyone runs the same app, and people on the newer version of iOS gain access to the new features you added. For information about how to add runtime checks, view Running code on a specific platform or OS version.
Beyond the app
Your app isn’t the only way to support interactions with your content. Adopt the following technologies to extend the reach of your app to other parts of the system.
Integrate your content with other system services
Some system services incorporate app-specific information, and use app extensions to collect that information. For example, a widget app extension lets you display your app’s content on the Home screen or lock screen. iOS supports many types of app extensions, including ones to support custom keyboards, Siri integration, notifications, and more. For a list of extensions and more information, view App Extensions.
Add an App Clip to provide fast access to your app’s features
An App Clip is a lightweight version of your app that lets people test out some of your app’s features without downloading and installing the full app. For example, a bike rental service might provide an App Clip to let someone rent a bike. When someone scans an App Clip Code for your service, the system downloads your App Clip and presents it. For more information, view App Clips.
Support the continuation of tasks on other devices
Handoff lets someone start an activity on one device and continue it in the same app on another device tied to the same Apple ID. For example, someone who starts an activity on their iPhone can continue that activity on their iPad or Mac. You define the activities you want to hand off between devices, and tell the system when one of those activities occurs. For more information, view Implementing Handoff in your app.
Support universal links for your app’s content
If your website and app offer similar content, add universal link support to your app. With universal links, you don’t need to create separate URLs to open content in your app. One URL opens your app when it’s installed or your website when it isn’t. For more information, view Allowing apps and websites to link to your content.
Explore more
Learn more about technologies that provide unique capabilities, yet integrate tightly with Apple platforms to form a seamless ecosystem for apps and games across iOS, iPadOS, macOS, tvOS, visionOS, and watchOS.